Object-Oriented Programming (OOP) helps Java developers organize code and make it reusable. By learning OOP, you can improve your coding skills and solve problems more easily.
In this guide, we’ll cover the basics of OOP in Java, explain four key principles, and provide simple coding exercises.
What is OOP in Java?
OOP organizes code around “objects” that represent real-world items. This approach makes code easier to read and faster to create. In Java, OOP allows you to build reusable code, which helps in making complex programs.
In OOP, you create classes that are like blueprints. These classes define objects with attributes (data) and actions (methods). Think of a class as a plan, and an object as a product built from that plan.
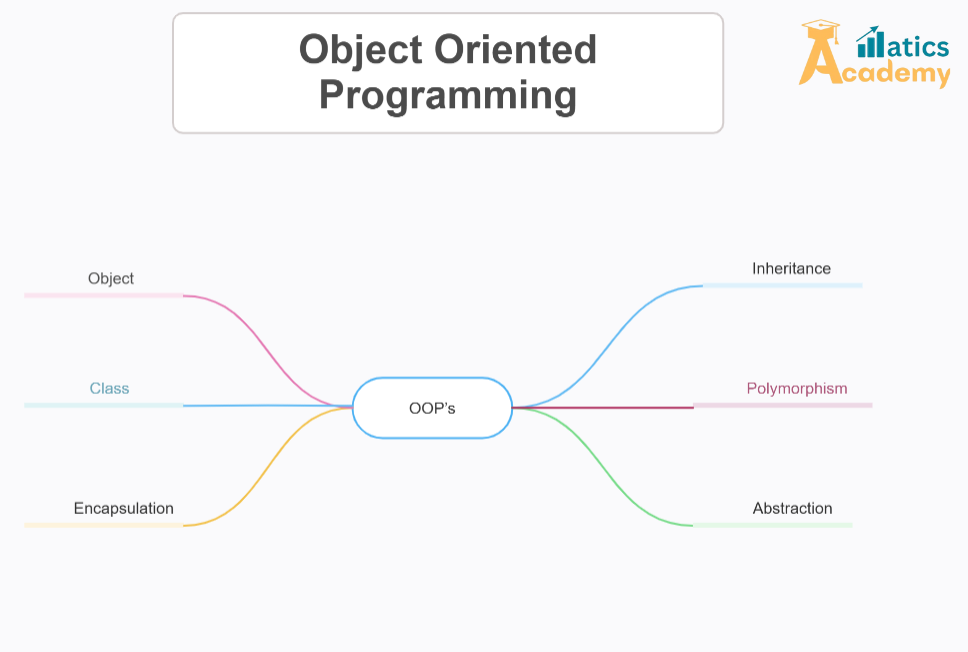
Key OOP Principles
Understanding these principles helps you write better code:
- Encapsulation: Groups data and methods in one class and keeps data private.
- Inheritance: Allows new classes to use code from existing ones, reducing duplication.
- Polymorphism: Lets objects act as their parent class, making code flexible.
- Abstraction: Hides complex details and shows only what’s important.
Why Use OOP in Java?
OOP has many benefits:
- Modularity and Reusability: Makes code easy to reuse in different projects.
- Simpler Debugging: Organized code is easier to fix.
- Scalability: You can add new features without breaking old code.
- Real-World Modeling: OOP works well for real-life problems.
Common Myths
Here are some myths about OOP:
- “Only for Big Projects”: Even small projects benefit from OOP.
- “Inheritance is Best”: Sometimes, combining classes is more flexible.
- “OOP Slows Code”: Modern Java optimizations keep OOP efficient.
Best Practices
Here are some tips for writing OOP code:
- One Purpose per Class: Each class should have one job.
- Clear Names: Use simple names for classes and methods.
- Private Data: Keep data private and control access with methods.
- Avoid Repeating Code: Reuse code whenever possible.
Example: A Basic Library Management System
1. Define the Book
Class
This class has attributes like title
, author
, and ISBN
. It also has methods to display the book details.
class Book { private String title; private String author; private String ISBN; // Constructor public Book(String title, String author, String ISBN) { this.title = title; this.author = author; this.ISBN = ISBN; } // Getters (Encapsulation) public String getTitle() { return title; } public String getAuthor() { return author; } public String getISBN() { return ISBN; } // Display method public void displayBookInfo() { System.out.println("Title: " + title); System.out.println("Author: " + author); System.out.println("ISBN: " + ISBN); } }
Simple OOP Project in Java: Library Management System
Below is a simple Java project using Object-Oriented Programming (OOP) principles like Encapsulation, Abstraction, Inheritance, and Polymorphism. The project allows you to manage books in a library, including borrowing and returning books.
1. Book
Class (Encapsulation)
public class Book { private String title; private String author; private boolean isAvailable; public Book(String title, String author) { this.title = title; this.author = author; this.isAvailable = true; // Initially, the book is available } public String getTitle() { return title; } public String getAuthor() { return author; } public boolean isAvailable() { return isAvailable; } public void setAvailable(boolean isAvailable) { this.isAvailable = isAvailable; } public void displayDetails() { System.out.println("Title: " + title + ", Author: " + author + ", Available: " + isAvailable); } }
2. Member
Class (Abstraction, Inheritance)
public class Member { private String name; public Member(String name) { this.name = name; } public String getName() { return name; } public void borrowBook(Library library, String bookTitle) { library.borrowBook(this, bookTitle); } public void returnBook(Library library, String bookTitle) { library.returnBook(this, bookTitle); } }
3. Library
Class (Reusability, Polymorphism)
import java.util.ArrayList; import java.util.List; public class Library { private List<Book> books; public Library() { books = new ArrayList<>(); } public void addBook(Book book) { books.add(book); } public void listBooks() { for (Book book : books) { book.displayDetails(); } } public Book findBook(String title) { for (Book book : books) { if (book.getTitle().equalsIgnoreCase(title)) { return book; } } return null; } public void borrowBook(Member member, String title) { Book book = findBook(title); if (book != null && book.isAvailable()) { book.setAvailable(false); System.out.println(member.getName() + " has borrowed the book: " + title); } else { System.out.println("The book \"" + title + "\" is not available."); } } public void returnBook(Member member, String title) { Book book = findBook(title); if (book != null && !book.isAvailable()) { book.setAvailable(true); System.out.println(member.getName() + " has returned the book: " + title); } else { System.out.println("The book \"" + title + "\" was not borrowed."); } } }
4. Main
Class (Execution)
public class Main { public static void main(String[] args) { // Create a library Library library = new Library(); // Create books Book book1 = new Book("Harry Potter", "J.K. Rowling"); Book book2 = new Book("Java Programming", "James Gosling"); // Add books to the library library.addBook(book1); library.addBook(book2); // Create a library member Member member = new Member("Alice"); // List all available books library.listBooks(); // Member borrows a book member.borrowBook(library, "Java Programming"); // Try borrowing the same book again member.borrowBook(library, "Java Programming"); // Member returns the book member.returnBook(library, "Java Programming"); // List all available books after returning library.listBooks(); } }
Interview Questions
1. Company: Google
- Question: Can you explain the difference between
==
and.equals()
in Java, and when would you use each? - Reason: Google often focuses on testing knowledge of fundamental Java concepts like object comparison and equality in code reviews and interviews.
2. Company: Microsoft
- Question: How would you implement a thread-safe singleton design pattern in Java?
- Reason: Microsoft looks for solid understanding of design patterns and concurrency concepts, especially for scalable enterprise applications.
3. Company: Amazon
- Question: What are some performance optimizations you can make when dealing with large datasets in Java? Can you explain how you would choose between using an
ArrayList
or aLinkedList
? - Reason: Amazon often asks candidates to think about system scalability, performance optimization, and when to use the right data structures.
4. Company: Oracle
- Question: What is the difference between a
HashMap
and aTreeMap
in Java? How do their internal implementations differ, and when would you use each? - Reason: Oracle expects candidates to have deep knowledge of Java collections and their performance characteristics.
5. Company: Adobe
- Question: What is the purpose of the
transient
keyword in Java, and how is it used in serialization? - Reason: Adobe focuses on candidates who understand Java’s serialization process, especially in distributed systems and large-scale applications.
These interview questions test a range of essential Java concepts and the ability to apply them in real-world scenarios.
Conclusion
Using OOP in Java helps you create code that is organized, easy to read, and easy to maintain. Practice with coding exercises to get comfortable with these concepts.
Happy coding!