Introduction
The For Loop in Java Programming is a fundamental control structure that allows developers to automate repetitive tasks, making it an essential tool for beginners learning programming. This guide will walk you through how the for loop works in Java, with detailed examples, syntax breakdowns, and best practices. Mastering Java’s for loop will enable you to write efficient and concise code for repetitive tasks, such as iterating over collections or performing calculations. Understanding For Loops in Java Programming is crucial for any aspiring developer.
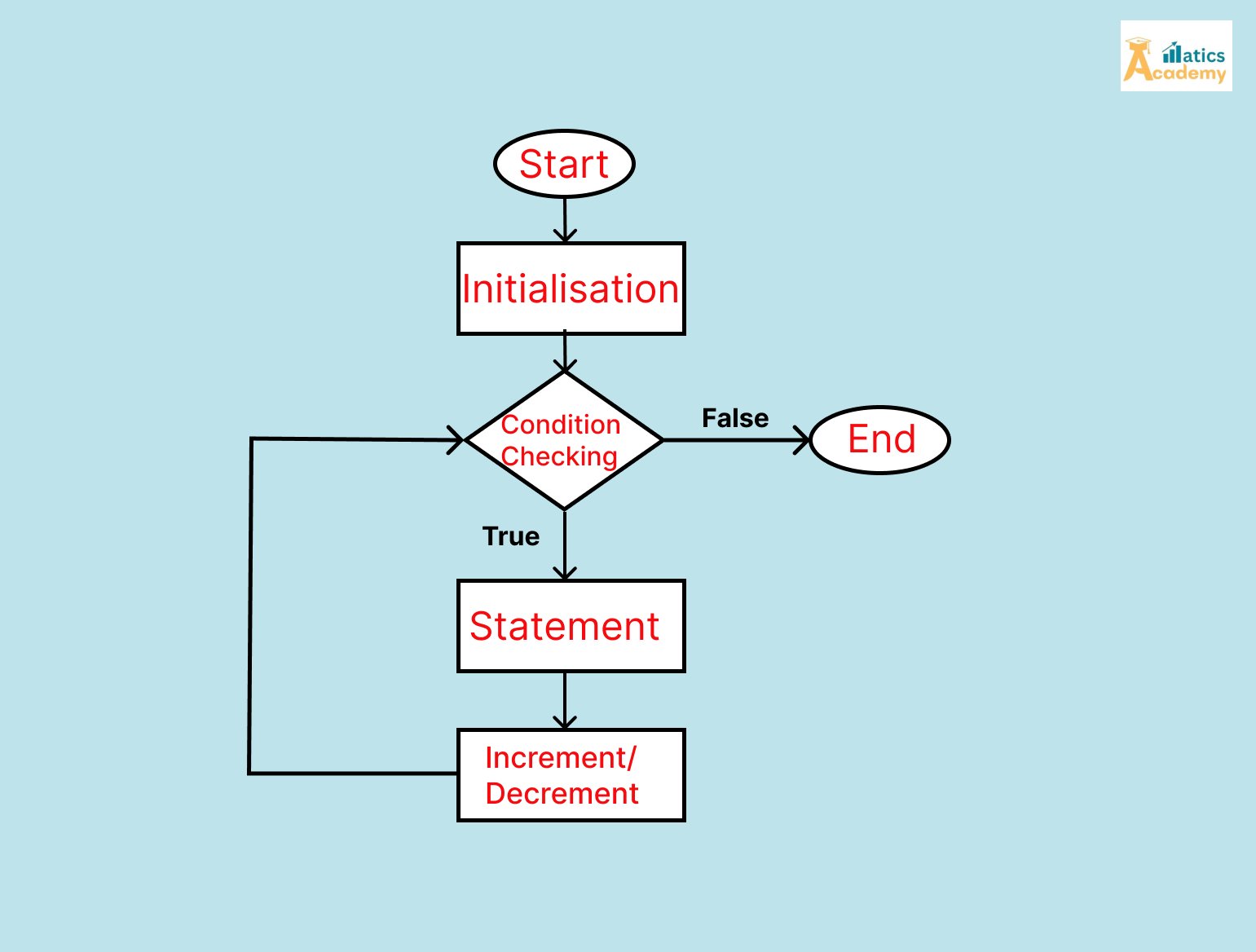
What is a For Loops in Java Programming?
A For Loops in Java Programming is a control flow statement that repeatedly executes a block of code as long as a specified condition remains true. For loops are commonly used for tasks that need repetition, such as processing items in arrays, iterating through a range of numbers, or performing cumulative calculations.
Syntax of a For Loop in Java Programming
The basic syntax of a for loop in Java is as follows:
for (initialization; condition; update) { // Code to execute }
Explanation of Syntax:
- Initialization: Sets the starting point for the loop, usually by defining and assigning a value to the loop counter (e.g.,
int i = 0
). - Condition: The loop continues as long as this condition evaluates to
true
. If it becomesfalse
, the loop stops. - Update: Executes after each iteration, usually to increment or decrement the counter variable.
- Flow of the For Loop:
Example: Basic For Loop in Java Programming
Let’s start with a simple example of a for
loop that prints numbers from 1 to 5.
public class ForLoopExample { public static void main(String[] args) { for (int i = 1; i <= 5; i++) { System.out.println("Number: " + i); } } }
Output:
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
In this example:
Update: i++
increments i
by 1 after each loop iteration, so i
moves closer to the condition becoming false
.
Initialization: int i = 1
sets the loop counter i
to 1.
Condition: i <= 5
ensures that the loop will run as long as i
is 5 or less.
Types of For Loops in Java Programming
Java provides two main types of for loops:
- Simple For Loop: Repeats a block of code a specific number of times, as shown in the example above.
- Enhanced For Loop (For-Each Loop): Used to iterate over arrays and collections without managing an index manually. This loop is ideal when you need to process each element in a collection without modifying the data structure directly
For Loops in Java Programming Example
The Enhanced For Loop (or For-Each Loop) simplifies array and collection traversal by eliminating the need for a loop counter. This makes the code cleaner and less prone to errors.
public class ForEachExample { public static void main(String[] args) { String[] colors = {"Red", "Green", "Blue"}; for (String color : colors) { System.out.println("Color: " + color); } } }
Output:
Color: Red
Color: Green
Color: Blue
In this example:
- No explicit counter is needed, as the for-each loop automatically goes through each element in the array.
color
takes each value in the arraycolors
during each iteration.
Practical Applications of For Loops in Java Programming
The For
Loops in Java Programming can be used in numerous ways in Java programs, such as:
- Calculating sums or averages: Iteratively process elements in an array or list to compute totals.
- Iterating over arrays or lists: Traverse through each element to perform actions on collections.
- Repeating tasks: Run code a set number of times (e.g., retrying a failed operation up to a certain limit).
Common Mistakes with For Loops in Java Programming
While For Loops in Java Programming are straightforward, common errors can lead to unintended results:
- Infinite Loops: Forgetting to update the loop variable can result in an infinite loop, which can freeze or crash your program. For instance:
for (int i = 0; i < 5;) { // Missing update System.out.println("This is an infinite loop!"); }
In this example, the i++
increment is missing, so i
will always be 0
, causing the loop to run indefinitely.
- Incorrect Conditions: Using the wrong condition can lead to unexpected results or skipped iterations. For example, using
i <= 5
instead ofi < 5
will cause the loop to execute one extra time. - Semicolon at the End of Loop Statement:Placing a semicolon (
;
) at the end of the loop header creates an empty loop body, causing the loop to run but do nothing.
Conclusion
The for loop is a powerful tool in Java programming, allowing you to automate repetitive tasks efficiently and improve code readability. By understanding the syntax and types of for loops, along with common mistakes to avoid, you are well-prepared to implement for loops in your Java projects.
Happy coding!
QUIZZES
Java For Loops Quizz
Question
Your answer:
Correct answer:
Your Answers