Introduction to while
Loop in Java
Loops are an essential part of programming, allowing us to repeat a block of code multiple times until a certain condition is met. The while
loop in Java is especially useful when you don’t know the exact number of times you need to repeat the loop—unlike the for
loop, which is ideal when the number of iterations is predetermined. Understanding the while loop in Java syntax is crucial for writing efficient code.
The while
loop is used to execute a block of code continuously as long as a specified condition evaluates to true
. Once the condition becomes false
, the loop exits, and the program proceeds with the next line of code. This type of loop is particularly helpful in situations with dynamic or variable-based conditions.
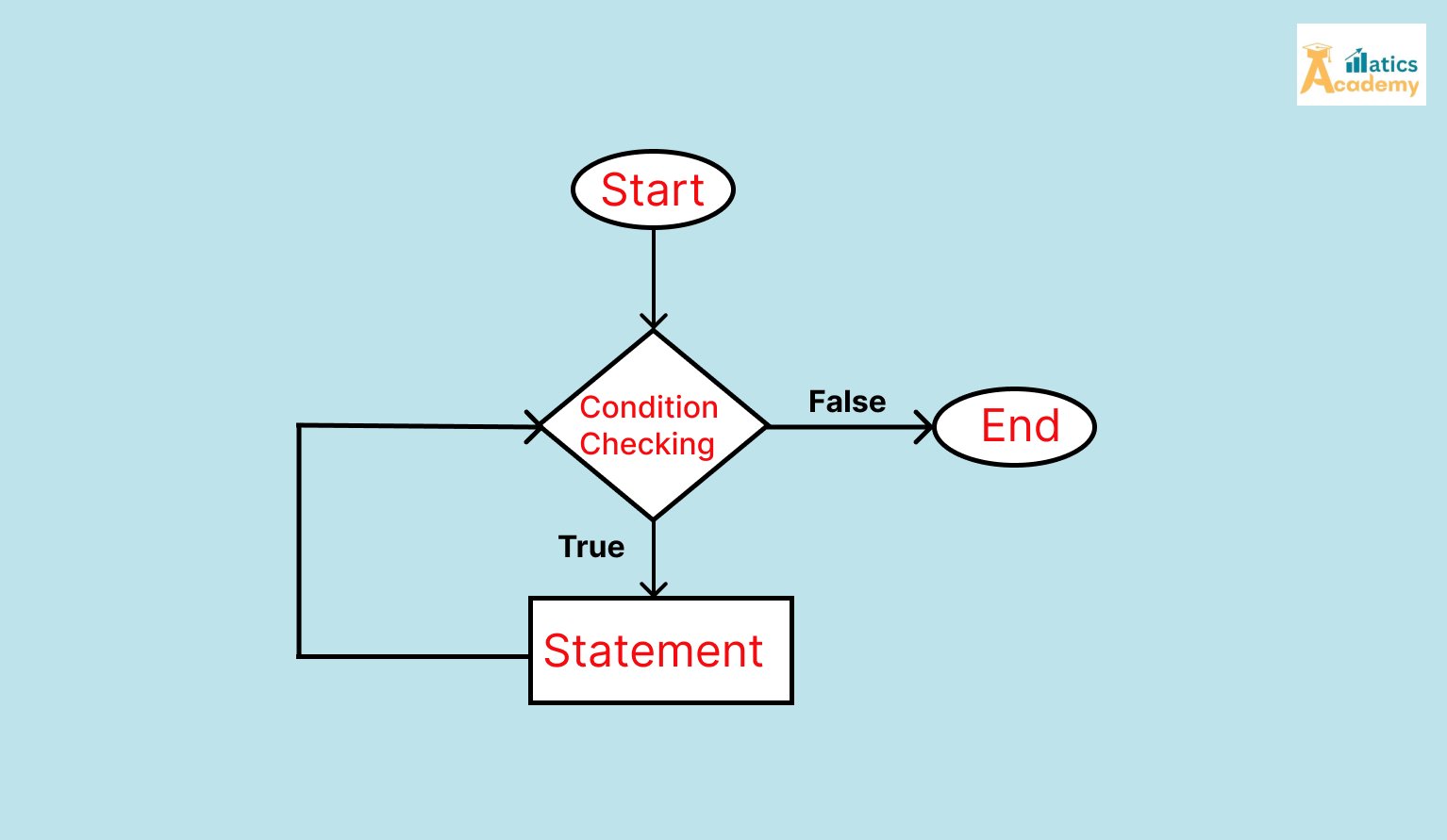
Algorithm for a while
Loop
- Initialize any required variables: Set up variables that may affect the loop’s condition before entering the loop.
- Check the loop condition: Each time the loop runs, Java checks if the condition is
true
. If so, the loop body executes. - Execute the loop body: Run the statements within the
{}
braces if the condition istrue
. - Update any variables as necessary: Modify any variables that may impact the condition, ensuring that the loop eventually ends.
- Exit the loop: When the condition becomes
false
, exit the loop and proceed with the next part of the program.
What is a while
Loop in Java?
A While
Loop in Java is a control flow statement that continuously executes a block of code as long as a specified condition remains true
. This loop is ideal for handling situations where the required number of iterations is unknown, making it flexible for scenarios where user input or other dynamic factors affect loop termination.
Syntax of the while
Loop in Java
The syntax of the while
loop in Java is straightforward:
while (condition) { // Code to execute }
Explanation of Syntax Components:
- condition: This is a boolean expression (evaluates to
true
orfalse
). The loop will continue to run as long as this condition remainstrue
. Once it becomesfalse
, the loop stops. - Code Block
{ }
: The code inside the curly braces{ }
is the body of the loop. This code will execute in each iteration as long as the condition istrue
. When the condition becomesfalse
, the loop exits, and the program moves to the next part of the code.
Java while
Loop Example
Let’s look at a practical example to better understand how the while
loop works in Java:
int count = 1; while (count <= 5) { System.out.println("Current count is: " + count); count++; // increment count by 1 }
Step-by-Step Explanation:
- Initialization: We start by setting the
count
variable to1
. - Condition Check: Before each iteration, Java checks if
count
is less than or equal to5
. Iftrue
, the loop body runs; otherwise, the loop exits. - Execution: When the condition is
true
, Java prints the current value ofcount
. - Increment: We increment the
count
by1
(count++
) at the end of each iteration.
Output:
Current count is: 1
Current count is: 2
Current count is: 3
Current count is: 4
Current count is: 5
When to Use the while
Loop in Java
The while
loop is especially useful in scenarios such as:
- Unknown Iterations: Use the
while
loop when the number of iterations is not fixed and depends on a condition that could change during runtime. - Condition-Driven Loops: Ideal for situations where you want to execute code based on a condition that might be influenced by user input, calculations, or other dynamic factors.
Common Pitfalls and Best Practices for Using while
Loops
Avoiding Infinite Loops
An infinite loop occurs when the while
loop’s condition never becomes false
. This can cause the program to run endlessly, which may freeze your program or crash the system. To avoid infinite loops:
- Ensure the condition will eventually become
false
. - Update variables in the loop body to eventually break out of the loop.
Example of an Infinite Loop (to Avoid):
int i = 1; while (i <= 5) { System.out.println(i); // Missing increment step for i, causing an infinite loop }
Handling Off-by-One Errors
An off-by-one error is a logic error where the loop runs one time too many or too few. It’s easy to accidentally set the loop to run for an extra iteration or stop one iteration too early. Double-check your loop condition and any increment/decrement statements to avoid this.
Using the do-while
Loop as an Alternative
In situations where you need to run the code at least once, even if the condition is false
, the do-while
loop is an alternative to while
. Unlike the while
loop, the do-while
loop checks the condition after executing the loop body, guaranteeing one execution.
Syntax of a do-while
Loop:
do { // Code to execute at least once } while (condition);
Example of a do-while
Loop
int count = 6; do { System.out.println("Count is: " + count); count++; } while (count <= 5);
Explanation: The above code prints “Count is: 6” once because the code block executes before the condition check. This is different from a while
loop, which wouldn’t execute if the condition were false initially.
Interview Questions
1. What is a while
loop, and how does it differ from a for
loop in Java?
- Company: TCS, Cognizant, Wipro
- Explanation: Interviewers may ask candidates to explain the basic structure of a
while
loop and how it works. Candidates should also compare it to afor
loop, noting that awhile
loop is often used when the number of iterations is unknown, as it only checks the condition at the start of each iteration.
int i = 0; while (i < 5) { System.out.println("Number: " + i); i++; }
2. What is the potential risk of an infinite loop with while
, and how can you prevent it?
- Company: Infosys, Accenture, Capgemini
- Explanation: Interviewers may ask about infinite loops, which can happen if the loop condition is always true. Candidates should explain that updating the loop variable or ensuring the condition will eventually evaluate to false is necessary to avoid an infinite loop.
- Example of Infinite Loop:
int i = 0; while (i < 5) { // Missing increment System.out.println("This will be an infinite loop!"); }
- Fix: Add
i++
to incrementi
, ensuring the condition will eventually be false.
Here are five frequently asked interview questions about while loops in Java, along with examples of companies that may focus on these concepts:
3. How does a do-while
loop work, and how is it different from a while
loop?
- Company: Oracle, Microsoft, Goldman Sachs
- Explanation: Candidates may be asked to explain the do-while loop, which is similar to a
while
loop but guarantees that the loop will execute at least once, as the condition is checked at the end. This is useful when the loop should run at least once regardless of the condition.
int i = 5; do { System.out.println("Number: " + i); i++; } while (i < 5);
In this case, the loop will print Number: 5
once, even though i
is initially not less than 5, because the condition is checked after the first iteration.
4. How can you use a while
loop to iterate through a collection, and when is this approach preferable?
- Company: Amazon, IBM, Deloitte
- Explanation: This question assesses a candidate’s understanding of using a
while
loop to iterate through collections, especially when using an iterator with a non-indexed collection like aSet
. Awhile
loop with an iterator can be particularly useful here.
List,[object Object]
In this example, the loop iterates through each element of the List
without requiring an index.
5. How can you use the break
and continue
statements in a while
loop, and why would you do this?
- Company: JP Morgan Chase, HCL, Google
- Explanation: Interviewers often ask about using
break
to exit a loop early andcontinue
to skip the current iteration. Candidates should explain how these statements provide more control over loop flow, especially when certain conditions require breaking out of or skipping loop iterations.
int i = 0; while (i < 10) { if (i == 5) { break; // Exit loop when i is 5 } if (i % 2 == 0) { i++; continue; // Skip even numbers } System.out.println("Odd number: " + i); i++; }
- In this example, the loop skips even numbers, only prints odd numbers, and exits when
i
reaches 5.
Conclusion
The while
loop in Java is a powerful tool when the number of iterations depends on a condition rather than a fixed count. Understanding its syntax, common pitfalls, and best practices will help you write effective loops and avoid issues like infinite loops and off-by-one errors. Experimenting with examples and varying conditions can help you gain confidence in using the while
loop in your projects.
With this guide, you should now have a solid understanding of how to use the while
loop effectively in Java.
Happy coding!
Quizzes
while loop in java Quiz
Question
Your answer:
Correct answer:
Your Answers