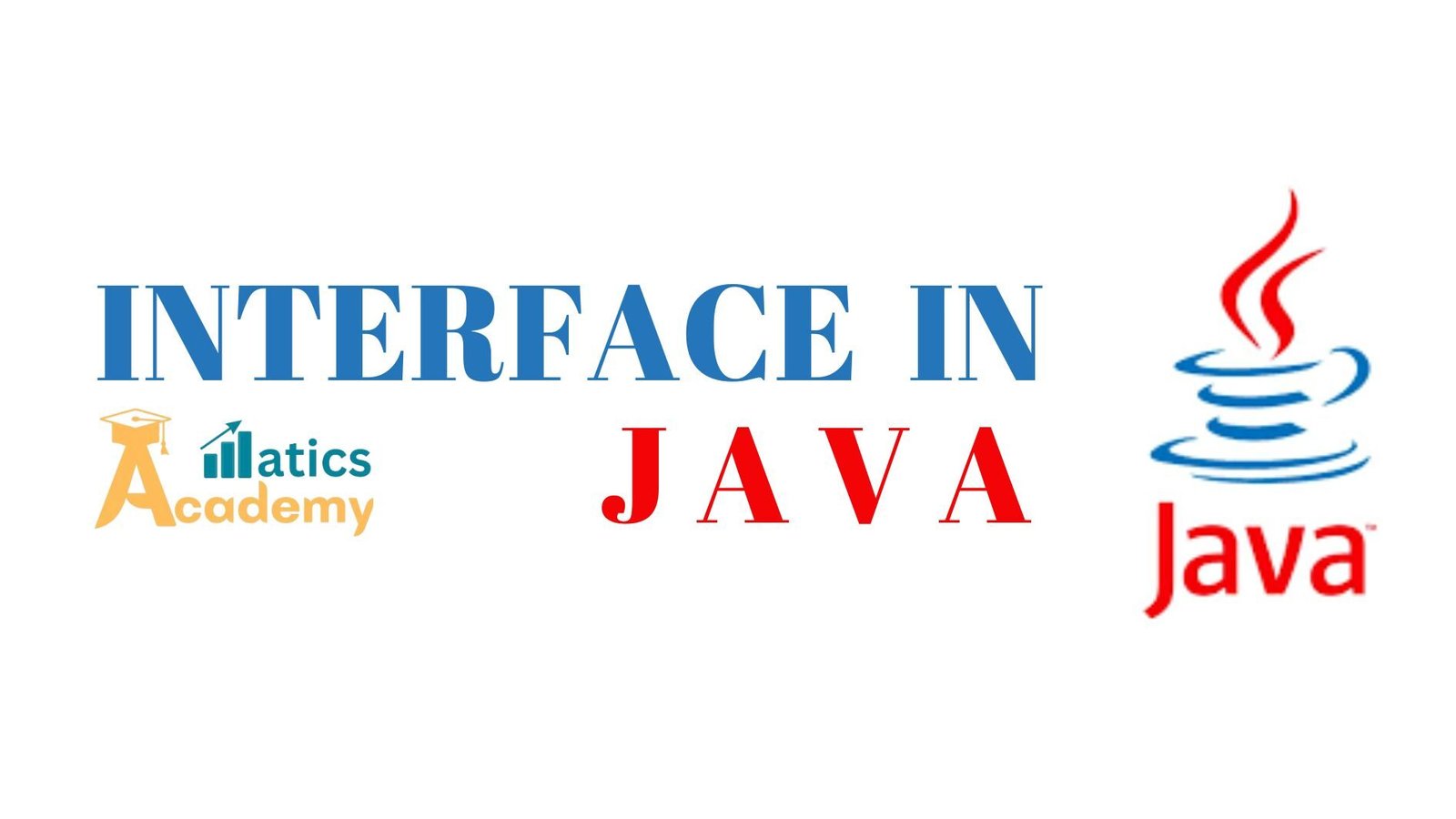
In Java, interfaces are essential in creating a contract that classes can implement without defining specific details. By using interfaces in Java, you can design flexible code that is also easy to maintain. This article explains the basics of interfaces, how to implement them, and why they are crucial to object-oriented programming.
What is an Interface in Java?
An interface in Java is a blueprint for a class that only contains abstract methods and constants. Unlike classes, interfaces cannot be instantiated directly; they only provide method signatures without implementations
When we decide on a type of entity by its behavior and not via attribute we should define it as an interface.
Syntax:
interface Animal { void makeSound(); void eat(); }
In the example above, the Animal interface outlines two methods: makeSound() and eat(). This setup defines a contract for any class implementing the Animal interface.
How to Implement an Interface in Java:
To use an interface, a class must implement it by providing concrete versions of each method. Here’s how:
// Define the Animal interface with two abstract methods interface Animal { // Abstract method to define sound behavior void makeSound(); // Abstract method to define eating behavior void eat(); } // Dog class implements the Animal interface and provides implementations for both methods class Dog implements Animal { // Implementing the makeSound method @Override public void makeSound() { System.out.println("Bark"); } // Implementing the eat method @Override public void eat() { System.out.println("Dog is eating"); } } // Main class to test the interface and implementation public class Main { public static void main(String[] args) { // Create an instance of Dog Animal myDog = new Dog(); // Calling makeSound method myDog.makeSound(); // Output: Bark // Calling eat method myDog.eat(); // Output: Dog is eating } }
Here, the Dog class provides its own version of makeSound() and eat(), fulfilling the contract set by the Animal interface.
Benefits of Using Interfaces in Java:
- Polymorphism: Interfaces in Java support polymorphism, allowing code flexibility and adaptability.
- Code Reusability: Classes implementing the same interface can be used interchangeably, which improves code reuse.
- Dependency Decoupling: By relying on interfaces, you can separate code logic from implementation details, making your code easier to modify.
Key Features of Interfaces:
- Abstract Methods: Traditional interfaces only contain abstract methods, but since Java 8, they can include default and static methods.
// Define the Animal interface with an abstract method and a default method interface Animal { // Abstract method (no implementation) void makeSound(); // Default method with a body default void sleep() { System.out.println("Sleeping..."); } } // Dog class implements the Animal interface and provides an implementation for makeSound class Dog implements Animal { // Implementing the abstract method @Override public void makeSound() { System.out.println("Woof!"); } } // Cat class implements the Animal interface and provides an implementation for makeSound class Cat implements Animal { // Implementing the abstract method @Override public void makeSound() { System.out.println("Meow!"); } } // Main class to test the interface and its implementations public class Main { public static void main(String[] args) { // Creating objects of Dog and Cat Animal myDog = new Dog(); Animal myCat = new Cat(); // Calling the makeSound method, which is implemented in each class myDog.makeSound(); // Output: Woof! myCat.makeSound(); // Output: Meow! // Calling the default sleep method from the interface myDog.sleep(); // Output: Sleeping... myCat.sleep(); // Output: Sleeping... } }
Output -> Click Here
Explanation:
- Interface Definition:
Animal
is an interface with an abstract methodmakeSound()
and a default methodsleep()
.makeSound()
: This is an abstract method with no implementation, requiring any class that implements theAnimal
interface to provide its own version.sleep()
: This is a default method with an implementation, so any class implementing theAnimal
interface can either use this implementation directly or override it.
- Implementing the Interface:
Dog
andCat
Classes: Both classes implement theAnimal
interface, so they must define themakeSound
method.- Each class has its own implementation of
makeSound
, displaying unique sounds for a dog and a cat.
- Using the Interface in Main:
- The
Main
class creates instances ofDog
andCat
. - It calls
makeSound()
on each instance, demonstrating polymorphism: both objects are treated asAnimal
types, but they respond with their specific implementations. - The
sleep()
method is called on both instances, using the default implementation provided by theAnimal
interface.
- The
- Constant Fields: Interfaces may only include public, static, and final fields, so all fields are constants.
// Define an interface with a constant field interface Constants { int MAX_SPEED = 100; // Implicitly public, static, and final } // Class implementing the Constants interface class Car implements Constants { private int speed; // Method to set speed up to MAX_SPEED public void setSpeed(int speed) { if (speed <= MAX_SPEED) { this.speed = speed; System.out.println("Speed set to " + this.speed + " km/h"); } else { System.out.println("Speed exceeds maximum limit of " + MAX_SPEED + " km/h"); } } } // Main class to test the constant field usage public class Main { public static void main(String[] args) { // Create an instance of Car Car myCar = new Car(); // Set speed within the allowed limit myCar.setSpeed(80); // Output: Speed set to 80 km/h // Attempt to set speed beyond MAX_SPEED myCar.setSpeed(120); // Output: Speed exceeds maximum limit of 100 km/h } }
Output -> Click Here
- Multiple Inheritance: While Java doesn’t support multiple inheritance for classes, it allows a class to implement multiple interfaces.
Default and Static Methods in Java Interfaces:
With Java 8, interfaces can now include default and static methods.
- Default Methods: Provide a default behavior, enabling interfaces to be backward-compatible without breaking existing implementations.
interface Animal { void makeSound(); default void sleep() { System.out.println("Sleeping..."); } }
- Static Methods: Allow utility methods within an interface to be called on the interface itself, not on its instances.
interface MathOperations { static int add(int a, int b) { return a + b; } }
Real-World Applications of Java Interfaces:
- API Design: Interfaces create consistent APIs, like Java’s List, Map, and Set interfaces, which structure the data collection framework.
- Dependency Injection: Interfaces in Java support dependency injection by providing a standard contract, enabling modularity.
- Multiple Implementations: You can provide distinct implementations for different scenarios. For instance, a PaymentProcessor interface might have implementations for credit card, PayPal, and bank transfers.
Best Practices When Using Interfaces in Java:
- Common Behaviors: Use interfaces to define common behaviors across different classes.
- Avoid Overusing Default Methods: Default methods should be limited to specific, reusable behaviors.
- Interface over Abstract Class: When the goal is to define a contract without shared state or code, use an interface.
Conclusion:
Interfaces in Java are fundamental in structuring clean, reusable, and maintainable code. They support polymorphism, separate code dependencies, and facilitate API design. As you deepen your Java skills, mastering interfaces will enhance your ability to build modular and adaptable applications, making them invaluable for creating reliable, flexible systems.
Interview Questions
1. What are the advantages of using interfaces in Java?(Wipro)
Interfaces allow you to define a contract for what a class should do, without specifying how. They enable multiple inheritance, improve code modularity, and allow for easier testing and maintenance.
2. Can an interface have constructors in Java?(Wipro)
No, interfaces cannot have constructors in Java because they cannot be instantiated.
3. How do interfaces support loose coupling in Java?(Cognizant)
Interfaces help achieve loose coupling by allowing classes to interact with one another through interfaces, rather than through direct implementation. This makes it easier to modify the code without affecting other components.
4. Can an interface extend multiple interfaces?(Cognizant)
Yes, an interface can extend multiple interfaces, allowing it to inherit methods from all of the extended interfaces. This supports multiple inheritance in Java interfaces.
Remember What You Learn!
Question
Your answer:
Correct answer:
Your Answers