In the world of Java programming, handling data is essential. Input/Output streams in Java provide a powerful way to read from and write to different data sources, such as files, memory, and network connections. This guide will explore the fundamentals of I/O streams in Java, their types, and practical examples to help you master data handling in your applications.

What Are Input/Output Streams in Java?
Input/Output streams in Java are abstractions that facilitate reading and writing data. They act as conduits between your program and data sources. Streams can be categorized into two main types: input streams and output streams.
- Input Streams: These streams read data from a source. For example, you can use an input stream to read data from a file or receive data from a network connection.
- Output Streams: These streams write data to a destination. You can write data to files or send data over a network using output streams.
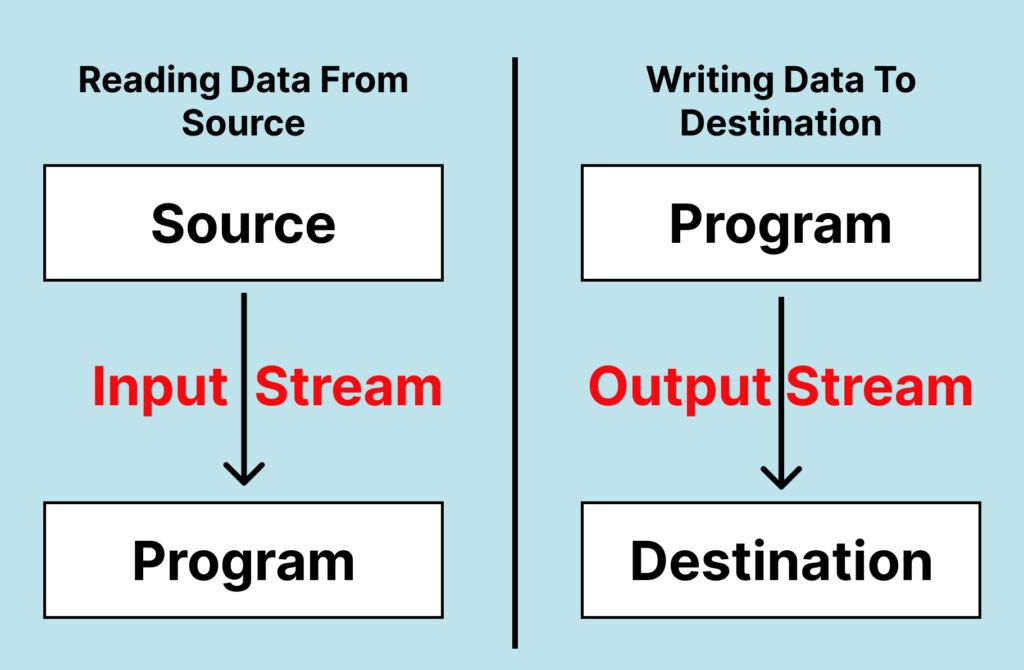
Types of Input/Output Streams in Java
Java provides a rich set of classes to handle I/O operations. The primary types of streams include:
- Byte Streams: These streams deal with raw binary data. They are ideal for reading and writing binary files, such as images or audio. Key classes include:
InputStream
: The abstract class for input byte streams.OutputStream
: The abstract class for output byte streams.FileInputStream
andFileOutputStream
: These classes read from and write to files, respectively.
- Character Streams: These streams handle character data. They are useful for text files, allowing the reading and writing of characters. Key classes include:
Reader
: The abstract class for character input streams.Writer
: The abstract class for character output streams.FileReader
andFileWriter
: These classes read from and write to text files, respectively.
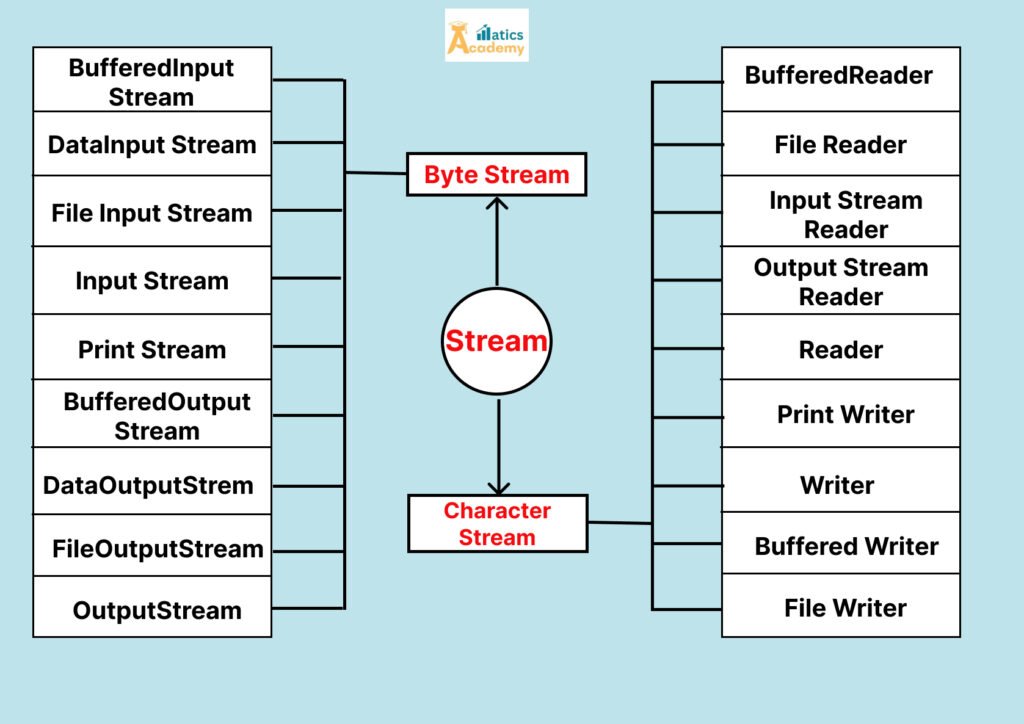
How to Work with Input/Output Streams in Java
Reading Data Using Input Streams
To read data from a file, you can use the FileInputStream
class. Here’s a simple example:
import java.io.*; public class FileReadExample { public static void main(String[] args) { try (FileInputStream fileInputStream = new FileInputStream("example.txt")) { int data; while ((data = fileInputStream.read()) != -1) { System.out.print((char) data); } } catch (IOException e) { e.printStackTrace(); } } }
Writing Data Using Output Streams
To write data to a file, you can use the FileOutputStream
class. Here’s how you can do it:
import java.io.*; public class FileWriteExample { public static void main(String[] args) { try (FileOutputStream fileOutputStream = new FileOutputStream("output.txt")) { String content = "Hello, Java I/O Streams!"; fileOutputStream.write(content.getBytes()); System.out.println("Data written to file successfully."); } catch (IOException e) { e.printStackTrace(); } } }
Using Buffered Streams for Efficiency
Buffered streams enhance the performance of I/O operations by reducing the number of reads and writes. You can wrap an InputStream
or OutputStream
in a BufferedInputStream
or BufferedOutputStream
. Here’s an example:
import java.io.*; public class BufferedExample { public static void main(String[] args) { try (BufferedOutputStream bufferedOutputStream = new BufferedOutputStream(new FileOutputStream("buffered_output.txt"))) { String content = "Using BufferedOutputStream for efficient writing."; bufferedOutputStream.write(content.getBytes()); System.out.println("Buffered data written successfully."); } catch (IOException e) { e.printStackTrace(); } } }
Best Practices for Using Input/Output Streams in Java
- Always Close Streams: Ensure that you close your streams to free up system resources. Using the try-with-resources statement simplifies this process.
- Handle Exceptions: I/O operations can fail for various reasons. Implement proper exception handling to manage errors gracefully.
- Use Buffered Streams: For better performance, especially with large files, use buffered streams.
- Prefer Character Streams for Text Data: Use character streams when working with text files to handle character encoding correctly.
- Consider Using NIO: For more advanced I/O operations, consider using Java NIO (New I/O) for non-blocking I/O and improved scalability.
Conclusion
Mastering input/output streams in Java is crucial for effective data handling in your applications. By understanding the types of streams, how to read and write data, and the best practices for managing I/O operations, you can significantly enhance your Java programming skills. Start implementing these concepts today, and unlock the full potential of Java I/O streams in your projects!
Interview Questions
1. What are input and output streams in Java, and how do they work?
- Company: TCS, Accenture, Infosys
- Explanation: Java’s I/O streams are used to read data from an input source (like a file or keyboard) and write data to an output destination (like a file or console). Streams are categorized into byte streams (
InputStream
andOutputStream
classes) and character streams (Reader
andWriter
classes).
2. What is the difference between byte streams and character streams in Java?
- Company: IBM, Wipro, Capgemini
- Explanation: Byte streams are used for handling raw binary data (like image files) and operate on bytes (
InputStream
andOutputStream
). Character streams are used for handling text data and operate on characters (Reader
andWriter
), supporting Unicode and handling conversions between bytes and characters automatically.
3. How does Java’s BufferedReader
improve performance, and how do you use it?
- Company: Oracle, Amazon, Google
- Explanation:
BufferedReader
is a character stream that reads data from anotherReader
(likeFileReader
) and buffers the input to optimize reading speed. Instead of reading each character individually, it reads chunks of data, reducing the number of I/O operations.
4. What are FileInputStream
and FileOutputStream
, and how do they work in Java?
- Company: Microsoft, JP Morgan Chase, Goldman Sachs
- Explanation: Java developers use FileInputStream and FileOutputStream to read and write binary data to files. FileInputStream reads byte data from a file, while FileOutputStream writes byte data to a file. These classes are especially useful for handling non-text files, such as images and videos.
5. What is serialization in Java, and how do you serialize an object?
- Company: Deloitte, Cognizant, HCL
- Explanation: Serialization in Java lets you convert an object’s state to a byte stream so you can save it to a file or transfer it across a network. Developers commonly use this technique when they need to store or transmit objects, such as in caching, deep cloning, or distributed computing.
Quizzes
Input/Output Streams Quiz
Question
Your answer:
Correct answer:
Your Answers