Introduction
Are you ready to dive into the world of synchronization in Java programming? Whether you’re developing an e-commerce platform, a real-time game, or a social media app, understanding Java synchronization is essential. This guide will explore key concepts and best practices for achieving thread safety, using practical examples to illustrate synchronization in Java and why it’s crucial in multithreaded applications.
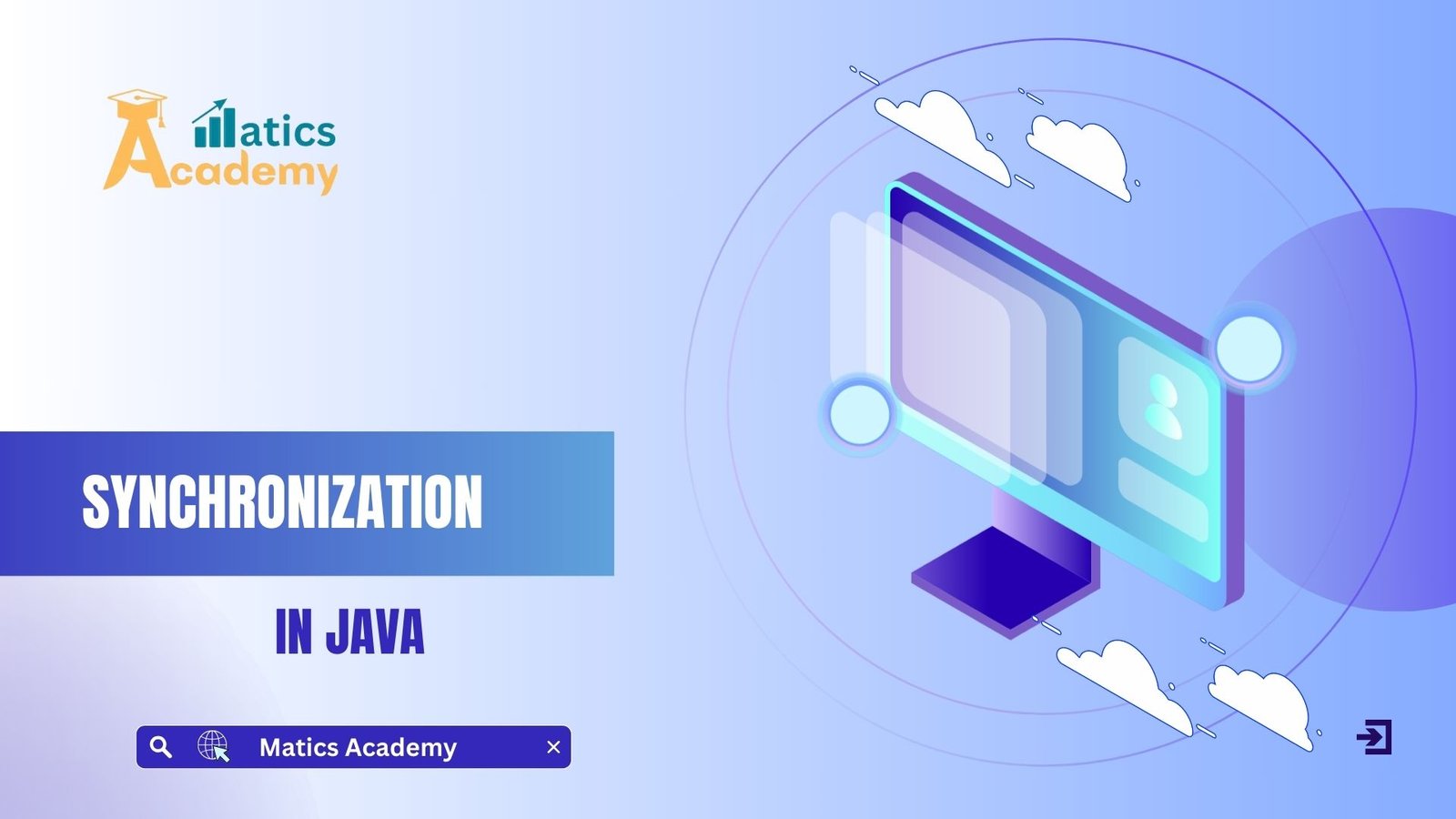
The Challenge of Multithreading
Imagine a busy coffee shop where multiple baristas are working simultaneously to prepare orders. Without proper coordination, chaos could easily ensue, with orders mixed up or delayed. This is similar to what happens in a multithreaded Java application without proper synchronization: multiple threads may access the same data concurrently, potentially causing data inconsistencies and errors.
When using multithreading in Java, some of the key challenges include:
- Race Conditions: Occur when multiple threads access shared data simultaneously, leading to potential errors.
- Data Inconsistency: Arises when one thread interrupts another’s operation on shared data.
- Deadlocks: Occur when threads are waiting for each other to release resources, causing the program to freeze.
These challenges highlights why synchronization in Java programming is necessary to maintain data integrity.
What is Synchronization in Java?
In Java programming, synchronization is a control mechanism that manages thread access to shared resources. It’s like a traffic light for threads, ensuring that only one thread can access a resource at any given time, preventing race conditions and data inconsistencies. This helps improve data consistency and thread safety in your Java programs.
Key Benefits of Synchronization:
- Prevents Thread Interference: Avoids situations where threads interrupt each other’s operations on shared resources.
- Maintains Data Consistency: Ensures data accuracy by restricting concurrent access.
- Establishes Happens-Before Relationships: Helps control the execution order of threads for improved stability.
Types of Synchronization in Java
Java provides several methods for synchronizing threads, including synchronized methods, synchronized blocks, static synchronization, and the volatile keyword.
- Synchronized Methods: Synchronized methods in Java allow only one thread to execute a method at a time.
public synchronized void synchronizedMethod() { // Code to execute }
- Synchronized Blocks: Synchronized blocks provide more granular control by locking only a specific portion of the code rather than the entire method.
public void methodWithSynchronizedBlock() { synchronized(this) { // Synchronized code block } }
- Static Synchronization: Static synchronization locks the entire class rather than just an object, making it ideal for data shared across all instances of a class.
public class ExampleClass { private static int sharedResource; public static synchronized void staticSynchronizedMethod() { // Code that accesses sharedResource } }
- Volatile Keyword: The
volatile
keyword ensures that a variable is always read from and written to main memory, bypassing the CPU cache. This guarantees that threads access the latest version of the variable.
private volatile int sharedVariable;
Implementing Synchronization: A Step-by-Step Guide
Let’s apply these concepts in a real-world example with a simple bank account transaction system.
Step 1: Identify the Shared Resource In this scenario, the account balance is the shared resource that multiple threads (transactions) may access.
Step 2: Create the Account Class
public class BankAccount { private double balance; public BankAccount(double initialBalance) { this.balance = initialBalance; } public synchronized void deposit(double amount) { balance += amount; System.out.println("Deposited: " + amount); } public synchronized void withdraw(double amount) { if (balance >= amount) { balance -= amount; System.out.println("Withdrew: " + amount); } else { System.out.println("Insufficient balance."); } } public double getBalance() { return balance; } public static void main(String[] args) { BankAccount account = new BankAccount(1000); // Initial balance is 1000 // Perform some deposit and withdrawal operations account.deposit(500); // Expected output: Deposited: 500.0 account.withdraw(300); // Expected output: Withdrew: 300.0 account.withdraw(1500); // Expected output: Insufficient balance. // Display the final balance System.out.println("Final balance: " + account.getBalance()); // Expected output: Final balance: 1200.0 } }
Output :
Deposited: 500.0
Withdrew: 300.0
Insufficient balance
Final balance: 1200.0
Use a synchronized method to allow only one transaction to modify the balance at a time, ensuring thread safety.
What is Synchronization in Java?
Synchronization in Java is like a traffic light for your code. It ensures that multiple threads access shared resources in an orderly fashion, preventing data corruption and race conditions. Imagine a social media app where thousands of users are liking posts simultaneously – synchronization keeps everything in check!
Multithreading and Synchronization: A Perfect Pair
In the world of Java, multithreading and synchronization go hand in hand. While multithreading allows your program to juggle multiple tasks, synchronization ensures they don’t step on each other’s toes. It’s like coordinating dancers in a complex choreography – each move must be perfectly timed.
Synchronization in Action: A Real-World Example
Let’s look at a simple example of synchronization in Java:
public class BankAccount { private int balance = 0; public synchronized void deposit(int amount) { balance += amount; } public synchronized void withdraw(int amount) { if (balance >= amount) { balance -= amount; } } }
In this e-commerce-inspired example, the synchronized
keyword ensures that deposits and withdrawals are handled one at a time, preventing any mix-ups in the account balance.
Types of Synchronization: Your Toolkit for Thread Safety
Java offers various synchronization techniques:
- Synchronized Methods: As seen in our example above.
- Synchronized Blocks: For more fine-grained control.
- Locks: Offering advanced features like timed waiting.
- Atomic Variables: For simple, high-performance operations.
Each type has its use case, much like choosing the right tool for a specific job in game development.
Synchronization in Threads
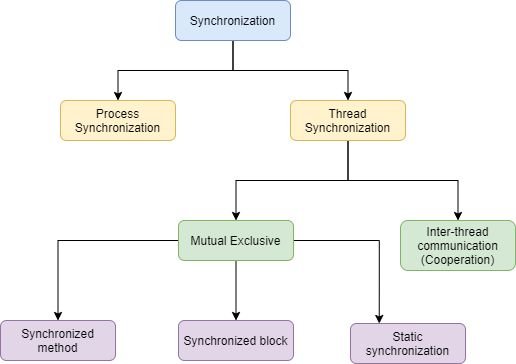
Advanced Concepts and Best Practices
As you delve deeper, explore concepts like:
- Deadlock prevention: Avoiding the “deadly embrace” of threads.
- Thread pooling: Managing resources efficiently in high-load scenarios.
- Volatile keyword: Ensuring visibility across threads.
Further Reading and Resources : Oracle Java Documentation on Synchronization
Interview Questions:
1.Explain the significance of the synchronized
keyword in Java and its impact on multithreaded applications.? (Google)
The synchronized
keyword ensures that only one thread can execute a block or method at a time, which helps prevent issues like race conditions and data inconsistencies. In a multithreaded environment, it’s crucial to protect shared resources to ensure data integrity, as Java threads may attempt concurrent access. Using synchronized
helps enforce a lock, so only one thread can access the code at any time, making it thread-safe.
2.What is a race condition, and how does Java synchronization help prevent it? (Microsoft)
A race condition occurs when two or more threads access shared data and try to change it simultaneously, leading to unpredictable results. Synchronization in Java helps prevent race conditions by allowing only one thread to access the critical section at a time. This ensures changes are made sequentially, preserving data consistency and preventing conflicts.
3.Can you explain the difference between a synchronized
method and a synchronized
block? (Amazon)
A synchronized
method locks the entire method, meaning only one thread can access it at a time. A synchronized
block, however, only locks a specific section of code, allowing finer control over the synchronized part. Synchronized
blocks are more efficient when only a part of the method needs thread safety, as they reduce the time a lock is held, improving performance.
4.How does the volatile
keyword differ from synchronized
in Java? (Facebook,Meta)
The volatile
keyword in Java ensures that updates to a variable are immediately visible to all threads. It does not create a lock but rather forces threads to read from the main memory instead of the cache, making it useful for variables that are read frequently and modified occasionally. In contrast, synchronized
ensures exclusive access to a code block, allowing only one thread to execute it at a time. Volatile
is thus useful for lightweight visibility control, while synchronized
is better suited for critical sections that require full locking.
5.Explain deadlock in Java and give an example of how it can occur with synchronized blocks? (Apple)
Deadlock in Java occurs when two or more threads are waiting for each other to release resources, resulting in an infinite wait. For example, if thread A holds a lock on object X and needs object Y to proceed, while thread B holds a lock on object Y and waits for object X, neither thread can proceed. Deadlock can often be avoided by acquiring locks in a consistent order or using Java’s tryLock()
method.
Test Your Knowledge: Synchronization in Java Challenge!
Question
Your answer:
Correct answer:
Your Answers