What Are Java Annotations?
Java annotations are special notes in your code. They give information about the code but do not change how it works. It help make your Java programs easier to read and understand. You can use them for many reasons, such as helping tools find errors or providing information to libraries and frameworks.
here quick guide through image:
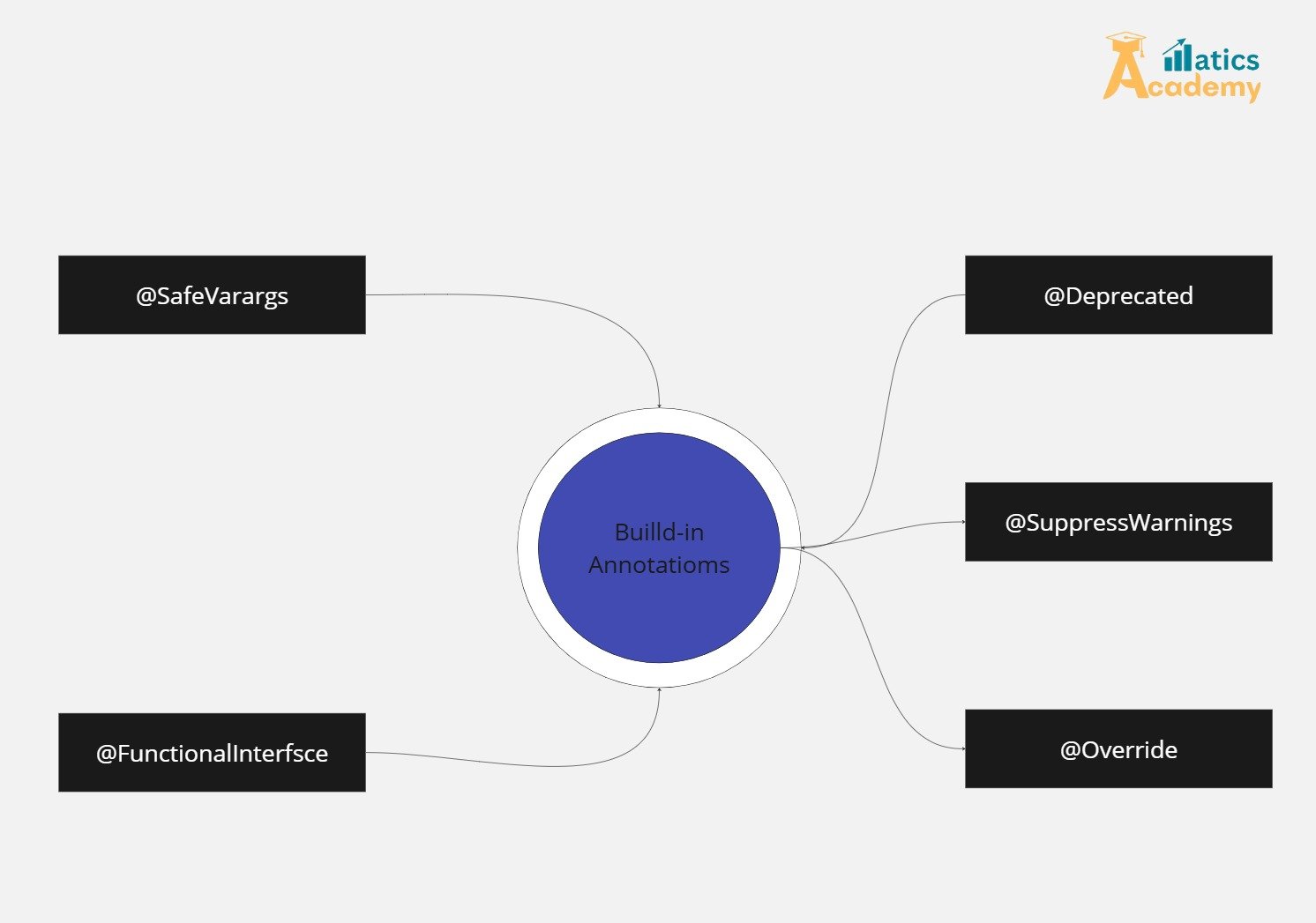
Why Use Annotations?
several reasons:
- Configuration: They simplify the setup of frameworks.
- Documentation: They add extra details about the code.
- Code Analysis: They help tools analyze your code for errors.
- Behavior Change: They can change how classes or methods work.
Basic Structure of Annotations
Let’s guide through java annotations through practical experience!
In Java, you create annotations using the @interface
keyword. Here is how to define one:
public @interface My Annotation{ String value();
Common Annotations
@Override: Indicates that a method overrides a method in its superclass. It’s useful for preventing errors, as the compiler will warn if the method does not actually override anything.
@Override public String toString() { return "Example"; }
@Deprecated: Marks a method, field, or class as outdated and suggests it should not be used in future code. You can add a message to specify alternatives.
@Deprecated public void oldMethod() { // Do something }
@SuppressWarnings: Tells the compiler to ignore specific warnings, which can be useful in situations where certain warnings are known but not problematic.
@SuppressWarnings("unchecked") public void someMethod() { // Code with unchecked operations }
@FunctionalInterface: Applied to interfaces to indicate that the interface is intended to be a functional interface (i.e., an interface with only one abstract method). The compiler will enforce this structure.
@FunctionalInterface public interface MyFunctionalInterface { void execute(); }
@SafeVarargs: Used with methods that take varargs parameters, especially in generic types, to avoid “unchecked” warnings. It can only be applied to final or static methods.
@SafeVarargs public static <T> void process(T... args) { // Process args }
@Retention: Specifies how long annotations with this type are to be retained. Options include SOURCE
(discarded during compilation), CLASS
(kept in class files but not available at runtime), and RUNTIME
(available at runtime).
@Retention(RetentionPolicy.RUNTIME) public @interface CustomAnnotation { String value(); }
@Target: Defines the contexts in which an annotation can be used, such as METHOD
, FIELD
, or TYPE
:
@Target(ElementType.METHOD) public @interface MyMethodAnnotation { // Annotation details }
Basic Syntax:
In Java, annotations are a form of metadata that provide data about a program but are not part of the program itself. Here’s the basic syntax for defining an annotation in Java:
// Define an annotation public @interface MyAnnotation { // Define annotation elements (methods without body) String value() default "default value"; // Optional element with default value int count() default 0; // Optional element with default value boolean active() default true; // Optional element with default value }
Explanation of the Syntax:
public @interface MyAnnotation
: This declares a new annotation type namedMyAnnotation
. The@interface
keyword is used instead ofclass
orinterface
.- Elements: Inside the annotation, you can define methods (called elements) that can have parameters.
- They can have default values (as shown in the examples), meaning that if the user does not provide a value for that element
- Annotations can contain any number of elements, but they should not have a body (i.e., no implementation).
- Usage: You can use this annotation in your code as follows:
@MyAnnotation(value = "example", count = 5) public class MyClass { // class implementation }
How to Create a Custom Annotation
Let’s create a simple code that marks important methods.
Step 1: Define the Annotation
import java.lang.annotation.ElementType; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.annotation.Target; @Target(ElementType.METHOD) // Can be used on methods @Retention(RetentionPolicy.RUNTIME) // Available during runtime public @interface Important { String value() default "This method is important!"; }
Step 2: Use the Annotation
Now, let’s use our @Important
annotation in a Java class:
public class MyClass { @Important("Pay attention to this method") public void myImportantMethod() { System.out.println("This method is important."); } public void myNormalMethod() { System.out.println("This is a normal method."); } }
Step 3: Access the Annotation
import java.lang.reflect.Method; public class AnnotationExample { public static void main(String[] args) { MyClass myClass = new MyClass(); myClass.myImportantMethod(); myClass.myNormalMethod(); // Check for annotations for (Method method : myClass.getClass().getDeclaredMethods()) { if (method.isAnnotationPresent(Important.class)) { Important important = method.getAnnotation(Important.class); System.out.println("Found important method: " + method.getName() + " with message: " + important.value()); } } } }
Let’s Brush Up your learning with the simple project! click here
Interview Questions
1. Company: Zoho
Question: What are Java annotations, and how are they used in a real-world application like Zoho CRM?
Answer: Java annotations provide metadata that can influence code behavior. In applications like Zoho CRM, annotations might be used to configure aspects such as validation, security, and database mappings. For example, @Entity
and @Table
annotations in an ORM (like Hibernate) can define how CRM data maps to a database, streamlining development and reducing boilerplate code.
2. Company: TCS
Question: What is the role of Java annotations in enterprise applications? How would you use them to manage configurations in a large-scale TCS project?
Answer: Annotations help manage configurations by allowing concise, declarative setup in enterprise applications. In a large-scale TCS project, annotations such as @Component
, @Service
, and @Autowired
could be used to automate dependency injection, while @Configuration
and @Value
would handle externalized configurations, making complex systems easier to maintain and extend.
3. Company: Infosys
Question: How would you implement a logging system using annotations in a Java application at Infosys?
Answer: Using a custom annotation like @LogExecution
, you could implement aspect-oriented logging by automatically logging method entry and exit. This approach, often achieved with @Aspect
and @Around
from Spring AOP, provides detailed logging without polluting the business logic, thus improving modularity and traceability.
4. Company: Zoho
Question: How can you use annotations to improve code maintainability and readability in Zoho’s fast-paced development environment?
Answer: Annotations simplify repetitive tasks, keeping code cleaner. In a fast-paced Zoho environment, annotations like @Override
, @Deprecated
, and custom validation annotations can reduce code clutter, making maintenance easier and allowing developers to understand and update code faster.
Conclusion
Java annotations are helpful tools that add information to your code without changing its structure. By learning how to create and use annotations, you can improve your code’s clarity and help others understand your work better.