Reflection in Java is an advanced feature that, nonetheless, proves highly accessible and beneficial for various programming needs. Essentially, it allows developers to inspect and interact with classes, methods, and fields during runtime. While this might initially sound complex, it is, in fact, quite straightforward when broken down and applied step-by-step. In this beginner’s guide, we will explore what reflection truly entails, delve into how it works, and, furthermore, examine its applications in Java programming.
By understanding reflection, developers can, in turn, create more flexible and dynamic applications. Additionally, reflection is particularly useful in scenarios where certain classes, methods, or fields are only known at runtime. As we progress through this guide, you’ll see how reflection works seamlessly within Java, allowing for a deeper level of interaction with code that, otherwise, would be difficult to achieve.
What is Reflection in Java?
In simple terms, It is the ability of a Java program to look at itself. This means you can:
- Get Information About Classes: Learn about the fields and methods of a class.
- Create Objects Dynamically: Instantiate classes at runtime without knowing their names at compile time.
- Access Private Members: Access fields and methods that are normally hidden from outside the class.
Key Concepts of Reflection
- Class Class: The foundation of reflection is the
Class
class. Every class in Java has an associatedClass
object that you can use to get information about the class. - Method Class: This represents a method in a class and allows you to invoke it dynamically.
- Field Class: This represents a field (or variable) in a class, allowing you to read or modify its value.
- Constructor Class: This represents a constructor, which you can use to create new instances of classes.
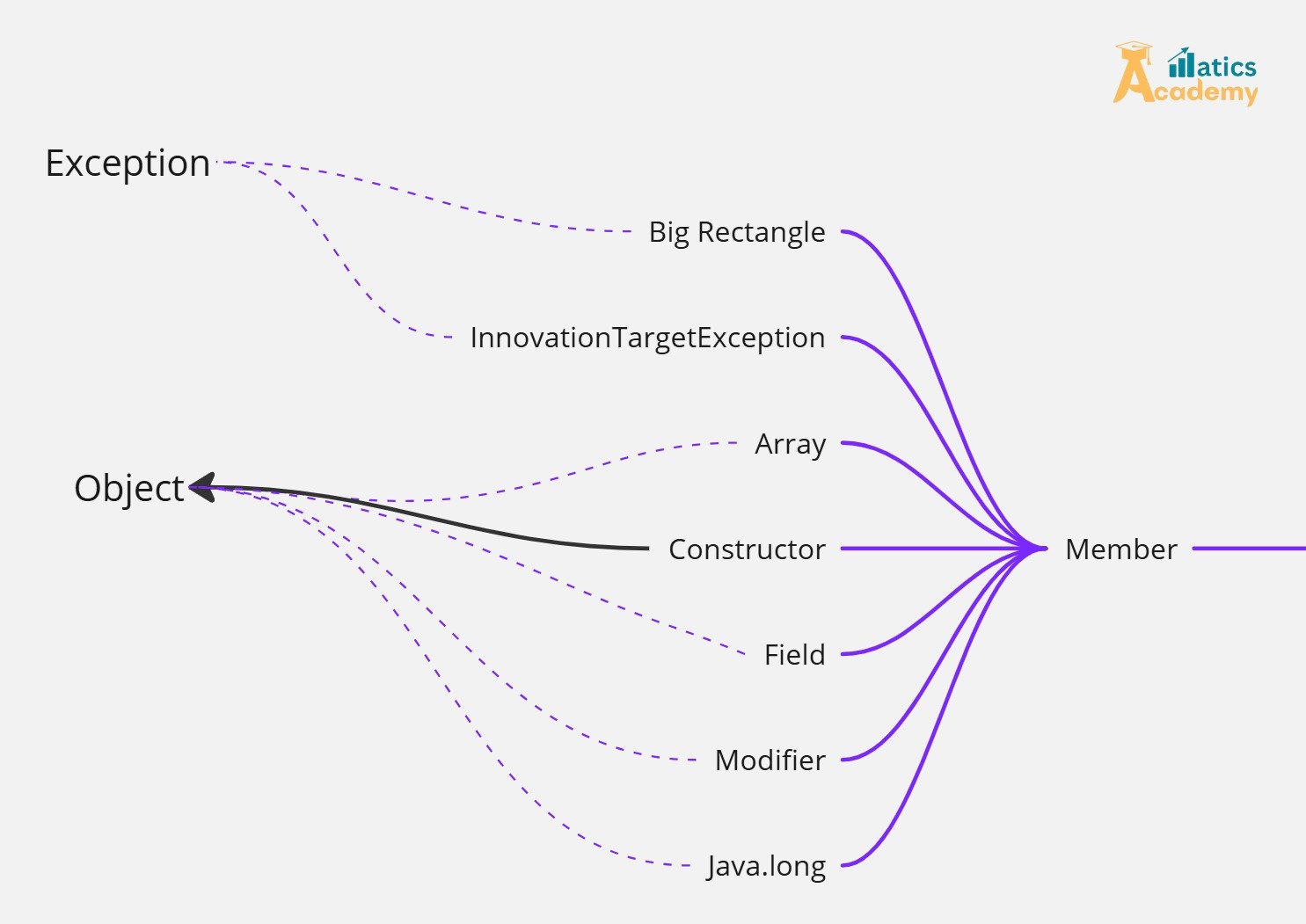
Reflection Syntax in Java
- Import Required Packages
import java.lang.reflect.Constructor; import java.lang.reflect.Field; import java.lang.reflect.Method;
2. Getting the Class Object
Class<?> clazz = Class.forName("YourClassName");
3.Creating an Instance Using Reflection
Constructor<?> constructor = clazz.getConstructor(parameterTypes); Object instance = constructor.newInstance(arguments);
4. Accessing Fields
Field field = clazz.getDeclaredField("fieldName"); field.setAccessible(true); // Allows access to private fields Object fieldValue = field.get(instance); // Get value field.set(instance, newValue); // Set value
5. Invoking Methods
Method method = clazz.getMethod("methodName", parameterTypes); method.invoke(instance, methodArguments);
How to Use Reflection in Java
Let’s look at a simple example to understand how reflection works in Java. Let’s try It out!
Example Code
import java.lang.reflect.Constructor; import java.lang.reflect.Field; import java.lang.reflect.Method; public class ReflectionExample { private String name; // Constructor public ReflectionExample(String name) { this.name = name; } // Method to display the name public void display() { System.out.println("Hello, " + name); } public static void main(String[] args) { try { // Get the Class object Class<?> clazz = Class.forName("ReflectionExample"); // Create an instance using the constructor Constructor<?> constructor = clazz.getConstructor(String.class); Object instance = constructor.newInstance("Java Reflection"); // Invoke the display method Method displayMethod = clazz.getMethod("display"); displayMethod.invoke(instance); // Access and modify the private field Field nameField = clazz.getDeclaredField("name"); nameField.setAccessible(true); // Allow access to the private field nameField.set(instance, "Updated Name"); // Display the modified name displayMethod.invoke(instance); // Outputs: Hello, Updated Name } catch (Exception e) { e.printStackTrace(); } } }
Explanation of the Code
- Getting the Class Object:
Class.forName("ReflectionExample")
retrieves the class object associated withReflectionExample
. - Creating an Instance: We use
getConstructor(String.class)
to find the constructor that takes aString
argument, andnewInstance()
to create a new object. - Invoking a Method: The
getMethod("display")
retrieves thedisplay()
method, andinvoke(example)
calls that method on our object.
Project: Using Reflections to Inspect a Class
Objective:
- To demonstrate how to inspect the fields, methods, and constructors of a class at runtime using Java’s Reflection API.
Steps:
- Create a simple class (
Person
) with some fields and methods. - Use reflection to inspect the
Person
class, print its fields, methods, and constructor.
Let’s see How this simple Project works! Click Here.
Explanation of Code:
The Class.forName("Person")
statement dynamically loads the Person
class at runtime. Specifically, the forName()
method accepts the fully qualified name of the class as a string, which enables loading classes dynamically based on external input or configuration. Consequently, it returns a Class
object representing the Person
class.
Getting Fields:
Next, by calling personClass.getDeclaredFields()
, we retrieve an array of Field
objects. This array includes all fields in the Person
class, even private ones. Then, using a loop, we iterate over each Field
object, printing out both the field names and their respective data types. This approach gives a complete view of the class’s attributes.
Getting Methods:
Similarly, personClass.getDeclaredMethods()
returns an array of Method
objects, representing all methods defined in the Person
class, including private ones. By looping over this array, we print out each method’s name and return type, which provides insights into the functionality exposed by the Person
class.
Getting Constructor:
Moreover, the line personClass.getDeclaredConstructor(String.class, int.class)
retrieves a constructor that takes specific parameters, in this case, a String
and an int
. This particular constructor can then be used to instantiate an object of the Person
class with these parameter types, providing flexibility in object creation.
Creating an Object and Invoking a Method:
To create an object of the Person
class, the newInstance()
method is called, resulting in a new instance. Finally, Method.invoke()
is used to call the printPersonInfo
method on this newly created object. This enables calling specific methods dynamically, which is especially useful when method names or parameters may only be known at runtime.
Interview Questions
1. Company: Zoho
Question: What is Java Reflection, and how does it work?
Answer: Java Reflection is a powerful API that allows inspection and manipulation of classes, fields, methods, and constructors at runtime. It works by using classes from the java.lang.reflect
package, such as Class
, Method
, and Field
, to access metadata and invoke methods or modify fields dynamically. This feature is useful in frameworks like Zoho’s CRM, where dynamic functionality is essential.
2. Company: TCS
Question: What are the key advantages and disadvantages of using Reflection in Java?
Answer: Reflection allows dynamic code execution, providing flexibility in frameworks, testing, and serialization. However, it has downsides, such as performance overhead due to runtime processing and security risks from accessing private fields. Careful use is needed in enterprise applications like TCS projects, balancing flexibility with efficiency and security.
3. Company: Infosys
Question: What is the difference between Class.forName()
, getClass()
, and getDeclaredMethods()
in Java Reflection?
Answer: Class.forName()
loads a class at runtime by name, getClass()
retrieves the runtime class of an instance, and getDeclaredMethods()
returns all methods declared in a class, including private ones. In Infosys projects, these methods can enable flexible design by dynamically loading and analyzing classes based on context.
4. Company: Zoho
Question: Explain how you would use reflection to dynamically call a method on an object without knowing the method name at compile time.
Answer: To call a method dynamically, first obtain the method name at runtime, then use Class.getMethod()
or Class.getDeclaredMethod()
with the method’s name and parameter types. Afterward, invoke method.invoke(object, args...)
to call the method on an instance. This technique could add flexibility in CRM systems, where unknown methods may be invoked based on user input or configurations.
5. Company: TCS
Question: How does Java Reflection interact with Java access control mechanisms (private, protected, and public members)? How can you access private members via reflection?
Answer: Reflection can override Java’s access controls, allowing access to private and protected members by calling setAccessible(true)
. In TCS enterprise projects, this enables special testing or framework needs, though it should be used cautiously to maintain encapsulation and security.
Conclusion
Reflection in Java is a powerful feature that allows you to inspect and manipulate classes at runtime. While it has its advantages, such as flexibility and dynamic behavior, it’s essential to use it wisely due to potential performance issues and complexity. By understanding how reflection works, you can enhance your Java programming skills and open up new opportunities for your projects.