Introduction
Are you ready to take your Java programming skills to the next level? If so, then understanding generics is essential. This powerful feature significantly enhances the flexibility, reusability, and type safety of your code. In this comprehensive guide, we’ll dive deep into the world of generics in Java, focusing on why developers use them, the advantages they offer, and how you can implement them effectively in your projects.
Why We Use Generics in Java
Java introduced generics in version 5 to help developers write type-safe, reusable code. Before generics, developers often needed to use explicit type casting when working with collections. This practice could lead to runtime errors if not handled carefully.
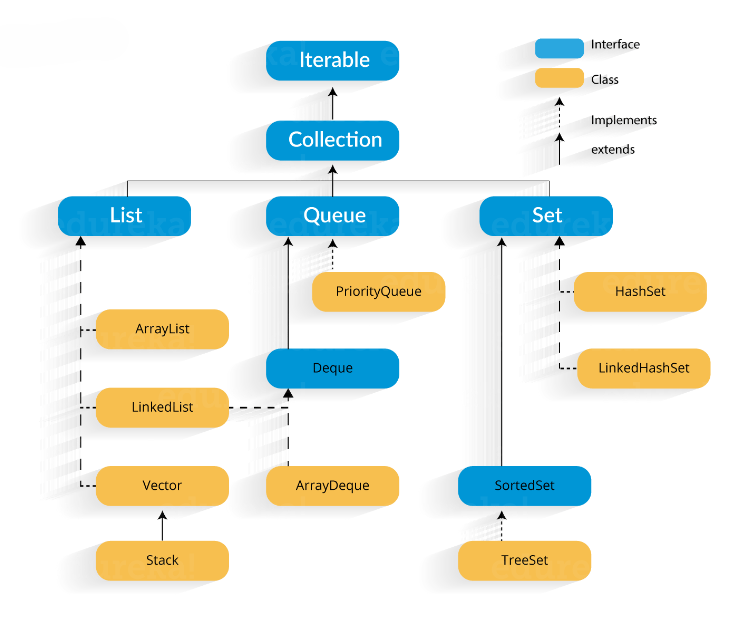
Consider this example, which demonstrates the limitations of pre-generics code:
List list = new ArrayList(); list.add("Hello"); String s = (String) list.get(0); // Requires explicit casting
In this code, the list doesn’t specify the type of elements it will hold, requiring explicit casting when retrieving an element. This could cause errors if the code retrieves an element of the wrong type.
With generics, you can specify the type of elements a collection will hold, making your code safer and clearer:
List<String> list = new ArrayList<>(); list.add("Hello"); String s = list.get(0); // No casting needed!
This simple but powerful change leads to numerous advantages when using generics in Java.
Advantages of Generics in Java
1.Type Safety: Generics enforce the use of the correct data types at compile-time, reducing the risk of ClassCastException
at runtime. This improves reliability and safety in your applications.
2.Code Reusability: With generics, you can write code that works seamlessly with different data types. This reduces code duplication, resulting in cleaner and more maintainable code.
3.Elimination of Casting: As shown in the earlier example, generics eliminate the need for explicit type casting. This not only makes your code cleaner but also minimizes the risk of casting errors, which can enhance application robustness.
4.Better Performance: Generics help avoid runtime type checking and casting, which can improve application performance, especially in critical scenarios.
5.Enhanced Readability:Code that uses generics often explains itself better, making it easier for other developers (and your future self) to understand and maintain. Clear code is crucial for long-term project success.
Generics Programming in Java
Let’s look at how you can implement generics effectively in your Java code.
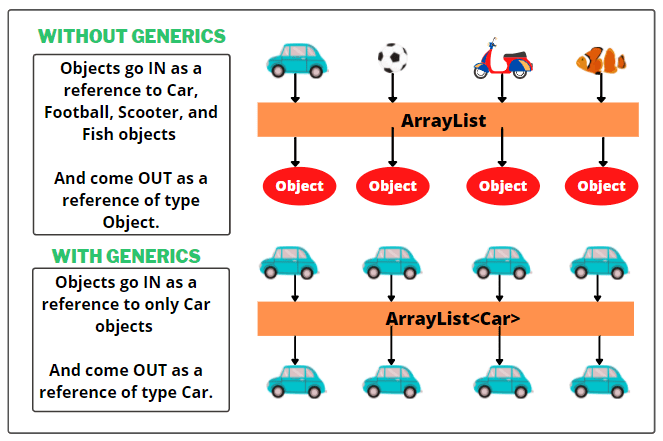
Generic Classes
Creating a generic class is straightforward and involves using type parameters. Here’s a simple example of a generic class:
public class Box<T> { private T content; public void set(T content) { this.content = content; } public T get() { return content; } }
In this example, we define a generic class named Box
that can hold any type of content. The type parameter T
allows flexibility in the type of data the class can store.
Now, you can use this Box
class with any data type:
Box<Integer> intBox = new Box<>(); intBox.set(42); int value = intBox.get(); Box<String> stringBox = new Box<>(); stringBox.set("Hello, Generics!"); String message = stringBox.get();
In these examples, we instantiate the Box
class with both Integer
and String
types, demonstrating the versatility of generics.
Generic Methods
Besides creating generic classes, you can also create generic methods within non-generic classes. This approach allows even greater flexibility in your code. Here’s an example of a generic method:
public class Utilities { public static <T> void printArray(T[] array) { for (T element : array) { System.out.print(element + " "); } System.out.println(); } }
This example defines a static method named printArray
that accepts an array of any type and prints its elements. The type parameter <T>
provides the flexibility needed for this functionality.
You can use the printArray
method with arrays of different types:
Integer[] intArray = {1, 2, 3, 4, 5}; String[] stringArray = {"Hello", "Generics", "in"}; Utilities.printArray(intArray); Utilities.printArray(stringArray);
In these examples, we print both an array of integers and an array of strings using the same method, showing how generics promote code reusability
Conclusion
In summary, generics in Java represent a significant advancement in programming. They enable developers to create type-safe, reusable, and efficient code. By understanding how generics work and implementing them effectively, you can write more reliable code with fewer errors. The advantages of generics—type safety, code reusability, elimination of casting, better performance, and enhanced readability—make them an essential tool in any Java programmer’s toolkit.
As you continue developing your Java skills, using generics will lead to more robust and maintainable applications. Follow the examples and guidelines provided in this guide, and you’ll be well on your way to mastering generics in Java and maximizing their potential in your projects.
you can refer https://docs.oracle.com/javase/tutorial/java/generics/index.html
Interview Questions:
1.What are generics in Java, and why were they introduced? (Amazon)
Generics allow Java developers to define classes, interfaces, and methods with type parameters, enforcing compile-time type safety. Introduced in Java 5, generics reduce the need for casting and enhance code reusability, making collections safer and preventing ClassCastException
.
2.How does type safety work with generics, and why is it beneficial? (TCS)
Generics enforce type safety by ensuring that only objects of a specified type are added to a collection or used in a generic class. This eliminates runtime casting errors and reduces the risk of ClassCastException
, enhancing code reliability and reducing debugging.
3.Can you explain the use of bounded type parameters in generics with an example? (Microsoft)
Bounded type parameters restrict a generic type to a subtype, providing more control. For instance, <T extends Number>
means that T can only be Number
or a subclass like Integer
or Double
. This allows using numeric operations within the method.
4.What are wildcards in generics, and when would you use ? extends
vs. ?
(IBM)
Wildcards allow flexibility with unknown types. ? extends
is used when reading data (covariance), like List<? extends Number>
, to accept any Number subtype. ? super
is for writing data (contravariance), as in List<? super Integer>
, to allow adding integers or subclasses.
5.How do generics improve the performance of Java applications? (Oracle)
Generics improve performance by eliminating the need for casting at runtime, thus avoiding the overhead associated with type checking and casting. Additionally, they help prevent runtime exceptions, leading to cleaner, faster code and optimized memory usage.
Test Your Knowledge: Generics in Java Challenge!
Question
Your answer:
Correct answer:
Your Answers