In Python, lists are mutable, meaning you can modify their elements. This flexibility allows you to update, replace, insert, and delete items based on your requirements. Let’s explore various ways to change list items.
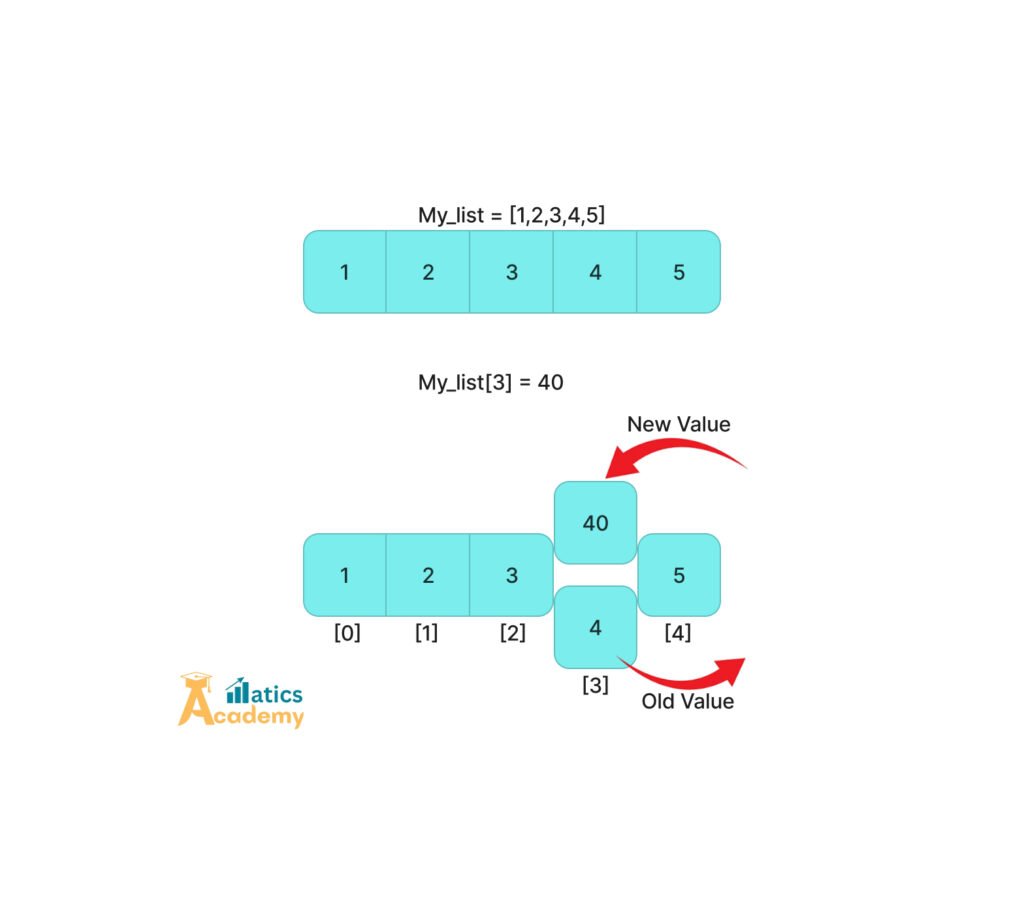
1. Changing an Item’s Value
You can directly modify a list element by referencing its index.
Syntax:
list[index] = new_value
Example:
numbers = [10, 20, 30, 40] numbers[1] = 25 print(numbers)
2. Changing a Range of Item Values
To update multiple elements simultaneously, use slicing.
Syntax:
list[start:end] = [new_value1, new_value2]
Example:
numbers = [10, 20, 30, 40] numbers[1:3] = [21, 31] print(numbers)
3. Inserting Items at a Specific Position
The .insert()
method allows you to insert an item at a specific index without replacing existing elements.
Syntax:
list.insert(index, item)
Example:
fruits = ['apple', 'banana', 'cherry'] print(fruits) fruits.insert(1, 'orange') print(fruits)
Interview Questions:
1. How would you modify the third element of a list in Python, and what happens if the index is out of range?(Google)
solution:
my_list = [10, 20, 30, 40, 50] my_list[2] = 100 print("Modified List:", my_list) try: my_list[10] = 200 except IndexError as e: print("Error:", e)
2. Given a list numbers = [10, 20, 30, 40, 50]
, how can you change the range of elements from index 1 to 3 to [100, 200, 300]
?
Solution:
numbers = [10, 20, 30, 40, 50] numbers[1:4] = [100, 200, 300] print("Modified List:", numbers)
3. How do you replace a list slice my_list[2:5]
with a single element in Python?(Microsoft)
Solution:
my_list = [1, 2, 3, 4, 5, 6] my_list[2:5] = [100] print(my_list)
4. What is the difference between positive and negative indexing in lists? Provide an example of each.(Infosys)
Solution:
Positive Indexing:
- Starts from the beginning of the list, with the first element at index 0.
my_list = [10, 20, 30, 40, 50] print(my_list[2])
Negative Indexing:
- Starts from the end of the list, with the last element at index -1.
my_list = [10, 20, 30, 40, 50] print(my_list[-2])
Remember What You Learn with Lists!
Question
Your answer:
Correct answer:
Your Answers