Introduction to String Methods In Python
In Python, string methods are built-in functions that can be used to manipulate, format, and analyze strings. These methods make working with text data much easier by allowing you to modify case, search for substrings, replace text, split strings, and much more. Understanding these methods, especially String Methods in Python, is essential for efficient text processing.
Additionally, Python provides various string methods for handling whitespace, checking string properties, and transforming data for better readability. Whether you’re cleaning up user input, extracting meaningful information, or formatting text for display, these methods help streamline the process. Mastering them can significantly enhance your ability to work with text-based applications, making data handling more efficient.
What Are String Methods In Python?
String methods are functions that are specifically designed to work with string objects. They are called directly on strings using dot notation (e.g., string.method()
). Mastery of String Methods in Python can significantly enhance your coding skills.
Example
text = "hello world" capitalized_text = text.capitalize() print(capitalized_text) # Output: Hello world
Data Types
- Integers, Floats, Strings, Lists, Tuples, Sets, Dictionaries
- Type conversion (casting)
- Mutable vs Immutable types
Operators
- Arithmetic, Comparison, Logical, Bitwise, Assignment
- Membership and Identity operators in Python
Control Flow
if
,elif
,else
- Nested conditions
- Short-hand
if
statements
Commonly Used String Method in Python
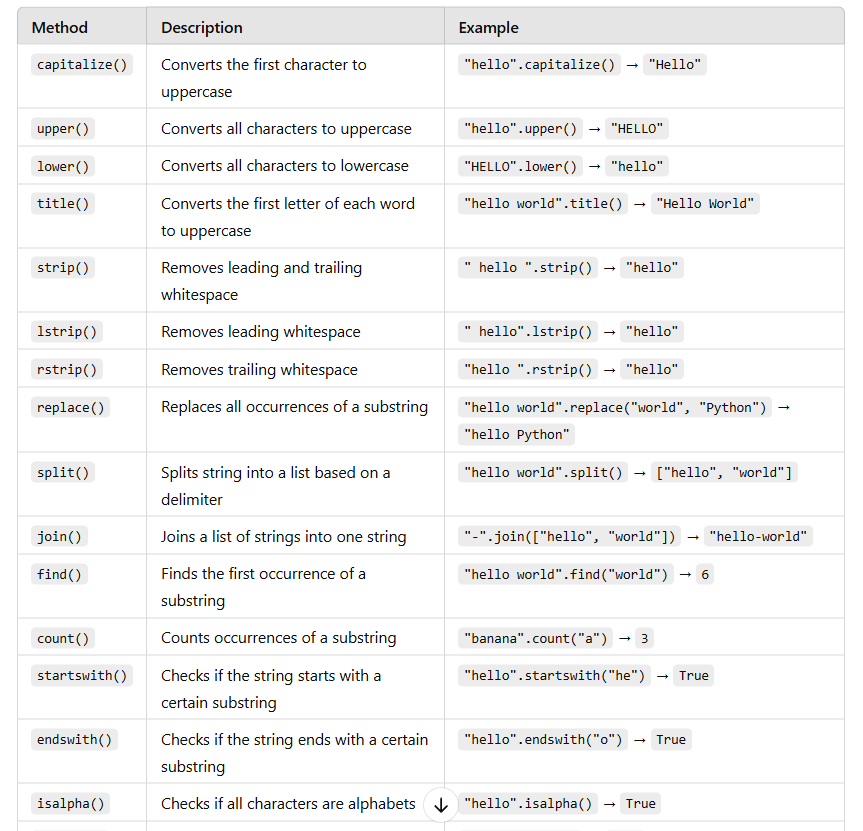
Examples in Action
text = " python programming " # Strip whitespace print(text.strip()) # Convert case print(text.upper()) print(text.title()) # Find and Replace print(text.replace("python", "JavaScript")) # Split and Join words = text.split() print(words) print("-".join(words))
Output
python programming
PYTHON PROGRAMMING
Python Programming
JavaScript programming
['python', 'programming']
python-programming
Why String Methods Matter
- They simplify text processing tasks by using various string methods in Python.
- They make data cleaning and formatting easier through efficient string manipulation.
- They allow quick validation and filtering of input data, which is crucial when dealing with strings in Python.
- They enhance readability and maintainability in code that includes string operations.
Additional Resources
Python Official Documentation – String Methods
Explore more String Methods in Python here.