Introduction to Arrays in Java Programming
Arrays in Java Programming allow developers to store multiple values of the same type in a single variable, making data handling more efficient. Thus, they’re essential in software development, data processing, and algorithms, which, in turn, helps boost both problem-solving skills and career potential.
Key Features of Arrays in Java Programming
- Fixed Size: The size is set upon declaration and cannot change.
- Index-Based: Elements are accessed via indices, starting at 0.
- Homogeneous Elements: All elements must be of the same type.
What You’ll Learn
In this guide, you’ll explore:
- Basics of Arrays: Definition, characteristics, and usage.
- Declaration and Initialization: How to set up arrays in Java.
- Multi-Dimensional Arrays: Working with 2D arrays for grid structures.
- Best Practices: Essential tips for using arrays effectively.
Array Fundamentals
Arrays are zero-indexed collections in Java that store data in contiguous memory. They can contain both primitive data types (e.g., int
, char
) and objects. Consequently, they are versatile and widely used in programming.
dataType[] arrayName = new dataType[size]; // Declare and initialize in one line
Array Declaration and Initialization
The syntax for declaring and initializing an array is as follows:
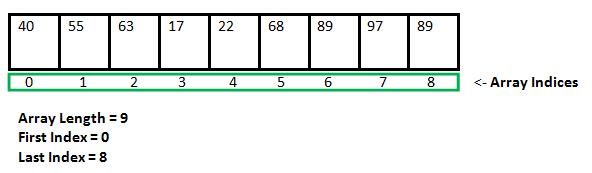
Example:
int[] numbers = {10, 20, 30, 40, 50};
Accessing Array Elements
You can access elements in an array by iterating over its indices.
for (int i = 0; i < numbers.length; i++) { System.out.println("Element at index " + i + ": " + numbers[i]); }
In this example, the code iterates through the numbers
array and prints each element, providing an intuitive look at array access.
One Dimensional Array in Java
A 1D (one-dimensional) array is the simplest type of array that stores a collection of elements in a linear format. It is widely used for handling lists of values such as student marks, employee salaries, and numerical sequences.
How It Works:
- The array
numbers
holds 5 integer values. - Elements are accessed using an index (starting from
0
). - It is useful for handling simple lists and sequential data.
Example :
public class OneDArrayExample { public static void main(String[] args) { int[] arr = {1, 2, 3, 4, 5}; System.out.println("1D Array Elements:"); for (int i = 0; i < arr.length; i++) { System.out.println("Element at index " + i + ": " + arr[i]); } } }
2D Array in Java
A 2D (two-dimensional) array is an array of arrays, essentially forming a matrix-like structure.
How It Works:
- The rows and columns define the structure.
- Access elements using two indices:
matrix[row][column]
. - Useful for applications requiring structured data.
Example :
public class TwoDArrayExample { public static void main(String[] args) { int[][] matrix = { {10, 20, 30}, {40, 50, 60}, {70, 80, 90} }; System.out.println("2D Array Elements:"); for (int i = 0; i < matrix.length; i++) { for (int j = 0; j < matrix[i].length; j++) { System.out.print(matrix[i][j] + " "); } System.out.println(); } } }
Multi-Dimensional Arrays
Java also supports multi-dimensional arrays, which are useful for organizing data in a grid-like format. Specifically, the most common form is the two-dimensional array.
Example:
In this example, the code creates a 2D array and then uses nested loops to print each element, arranged as a matrix.
int[][] matrix = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} }; for (int i = 0; i < matrix.length; i++) { for (int j = 0; j < matrix[i].length; j++) { System.out.print(matrix[i][j] + " "); } System.out.println(); }
Example program for getting input from the users :
import java.util.Scanner; public class OneDArrayInput { public static void main(String[] args) { Scanner sc = new Scanner(System.in); System.out.print("Enter the size of the array: "); int n = sc.nextInt(); // Getting array size int[] arr = new int[n]; // Declaring the array System.out.println("Enter " + n + " elements:"); for (int i = 0; i < n; i++) { arr[i] = sc.nextInt(); // Taking input for each element } System.out.println("1D Array Elements:"); for (int i = 0; i < n; i++) { System.out.print(arr[i] + " "); } sc.close(); } }
Summary
In summary, arrays are powerful data structures in Java that allow efficient data management through fixed sizes and homogeneous elements. Additionally, multi-dimensional arrays enable the organization of complex data structures, making them invaluable in many applications.
Learning Outcomes
After completing this guide, learners will be able to:
- Implement arrays in Java applications.
- Access and modify elements within one- and two-dimensional arrays.
- Recognize when to use alternative structures like
ArrayList
.
Common Interview Questions
What is an Array in Java, and how is it different from ArrayList?
Companies: Amazon, TCS, Accenture
How do you initialize an Array in Java, and what are the various ways to do so?
Companies: Infosys, Cognizant, Wipro
Explain the concept of a multidimensional array in Java and provide examples.
Companies: Google, Microsoft, IBM
How can you find the largest and smallest elements in an array?
Companies: Facebook, Oracle, Capgemini
What are the limitations of arrays in Java? How can these limitations be addressed?
Companies: Adobe, SAP, JPMorgan Chase
Practice Exercises
- String Array: Write a program that stores names in an array and prints them.
- Average Calculation: Create a program that calculates the average of numbers in an integer array.
Additional Resources
Books:
- “Java: The Complete Reference” by Herbert Schildt
- “Effective Java” by Joshua Bloch
Arrays
Question
Your answer:
Correct answer:
Your Answers