Closures in Python: Unlocking the Power of Nested Functions
Closures in Python are a powerful and elegant feature in Python, enabling the creation of functions with their own encapsulated environments. They are widely used in scenarios that require state retention, callback functions, and decorators. In this article, we’ll explore what closures are, how they work, and their real-world applications.
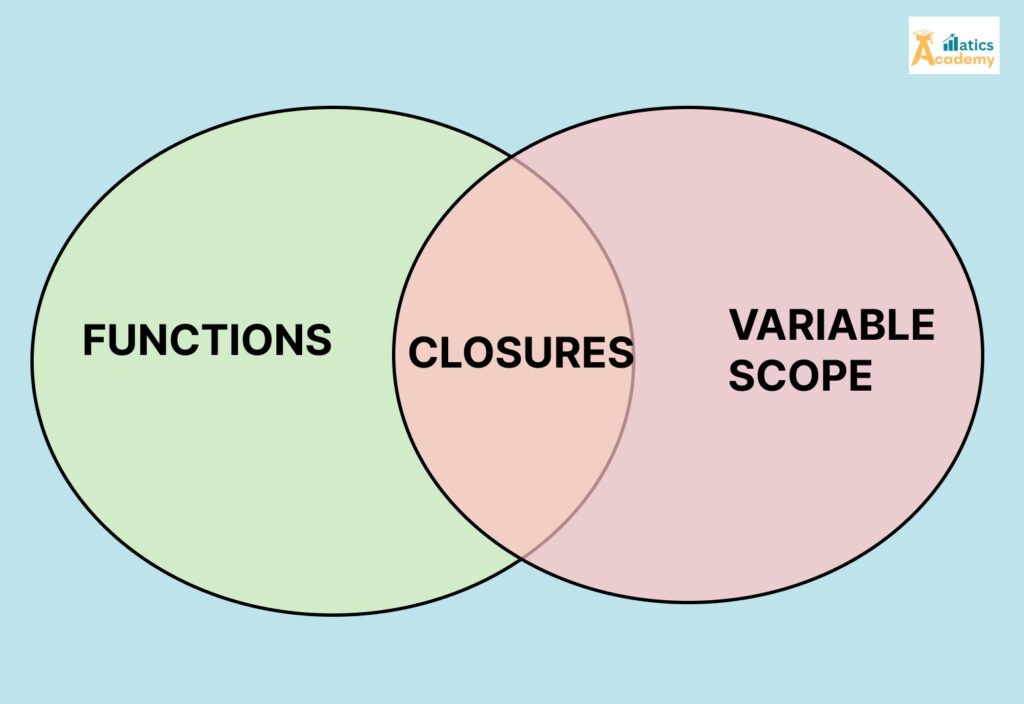
What Are Closures?
A Closure in Python is a nested function that retains access to the variables in its enclosing scope, even after the outer function has completed execution. This behavior is possible because Python treats functions as first-class objects and allows functions to capture and “close over” their surrounding variables.
Closures are created when:
- A nested function is defined inside an outer function.
- The nested function references variables from the enclosing scope.
- The outer function returns the nested function.
How Closures in Python Work
Here’s a step-by-step breakdown with an example:
def outer_function(x): def inner_function(y): return x + y # 'x' is captured from the outer function return inner_function closure_example = outer_function(10) # Returns 'inner_function' with 'x' bound to 10 print(closure_example(5)) # Output: 15
Define the Outer Function:
outer_function
defines a variablex
and a nestedinner_function
.
Return the Nested Function:
- The
inner_function
is returned byouter_function
.
Retain Variables in Scope:
- The returned function (
closure_example
) retains access tox
from theouter_function
, even though the outer function has finished executing.
Why Use Closures?
- Data Encapsulation:
- Closures provide a way to encapsulate data and logic within a function without exposing it globally.
- State Retention:
- Closures allow functions to retain state between calls, making them ideal for scenarios like counters or accumulators.
- Dynamic Function Generation:
- Generate specialized functions on the fly by capturing specific parameters.
- Cleaner Code:
- Closures help create concise and readable code, especially for callbacks and decorators.
Real-World Applications of Closures
Custom Counters:
def counter(start=0): def increment(): nonlocal start start += 1 return start return increment count = counter(5) print(count()) # Output: 6 print(count()) # Output: 7
2.Dynamic Function Creation:
def multiplier(factor): def multiply(number): return number * factor return multiply double = multiplier(2) triple = multiplier(3) print(double(5)) # Output: 10 print(triple(5)) # Output: 15
Callbacks:
- Closures are often used in GUI programming or asynchronous tasks to retain references to specific data.
Decorators:
- Closures form the foundation of Python decorators by wrapping functions to modify their behavior.
Closures vs Other Scoping Mechanisms
Feature | Closures | Global Variables | Classes |
---|---|---|---|
Encapsulation | High | Low | High |
Memory Usage | Efficient | Inefficient for multiple vars | Can be high for objects |
Ease of Use | Moderate | Easy | Moderate |
Common Mistakes with Closures
1.Modifying Variables:
Use the non local
keyword if you need to modify the captured variable within the nested function.
def outer(): x = 5 def inner(): nonlocal x x += 1 return x return inner
2.Confusion with Scope:
Ensure variables in closures are not accidentally shadowed by other variables with the same name.
Conclusion
Closures are a versatile feature in Python, enabling functions to retain access to their enclosing scope and enhancing code re usability. From building stateful functions to designing decorators, closures empower developers to write cleaner, more efficient code.
By mastering closures, you can unlock powerful programming patterns that simplify complex tasks and improve the modularity of your code. Start exploring closures today and elevate your Python programming skills!
INTERVIEW QUESTIONS
1.How would you write a Doc-string for a Python closure? Provide an example.
Company:Google
- Answer: A closure’s Doc-string should describe the outer function, its parameters, and what the inner function does. Example:
def multiplier(factor): """ Create a closure that multiplies a given number by a specified factor. Parameters: factor (int or float): The factor by which to multiply numbers. Returns: function: A function that takes a number and returns it multiplied by the factor. """ def multiply_by_factor(number): """ Multiply the given number by the outer factor. Parameters: number (int or float): The number to multiply. Returns: int or float: The product of the number and the factor. """ return number * factor return multiply_by_factor
2.How would you document the purpose of the outer function in a closure?
Company:Google
- Answer: The doc-string for the outer function should explain:
- The purpose of the closure.
- The parameters passed to the outer function.
- The return value (typically the inner function).
- Example:
def adder(addend): """ Create a closure that adds a specified value to a given number. Parameters: addend (int or float): The value to add. Returns: function: A function that takes a number and returns the sum. """ pass
3.What should the doc-string for the inner function of a closure include?
Company:Amazon
- Answer: The inner function’s doc-string should describe:
- The arguments it accepts.
- The operation it performs using variables from the outer scope.
- The value it returns.
4.Why is it important to document closures in Python?
Company:Google
- Answer: Documenting closures is important because:
- Closures involve multiple scopes, and the relationship between outer and inner functions can be complex.
- Clear documentation helps developers understand the role of captured variables and how the closure behaves.
- It improves maintainability and ensures proper use of the closure.
5.How do you describe the use of non-local variables in a closure’s doc-string?
Company:TCS
- Answer: The doc-string should explain that the inner function uses non-local variables from the outer scope and specify their purpose. Example:
def counter(): """ Create a closure that acts as a counter. Returns: function: A function that increments and returns a nonlocal counter value. """ count = 0 def increment(): """ Increment the nonlocal counter by 1. Returns: int: The updated counter value. """ nonlocal count count += 1 return count return increment
QUIZZES
Closure in python Quiz
Question
Your answer:
Correct answer:
Your Answers