In Python, Naming a thread is a crucial practice for improving code readability and debugging efficiency. When working with Python’s threading
module, assigning meaningful names to threads helps you easily identify their purpose during execution, especially in complex, multithreaded applications.
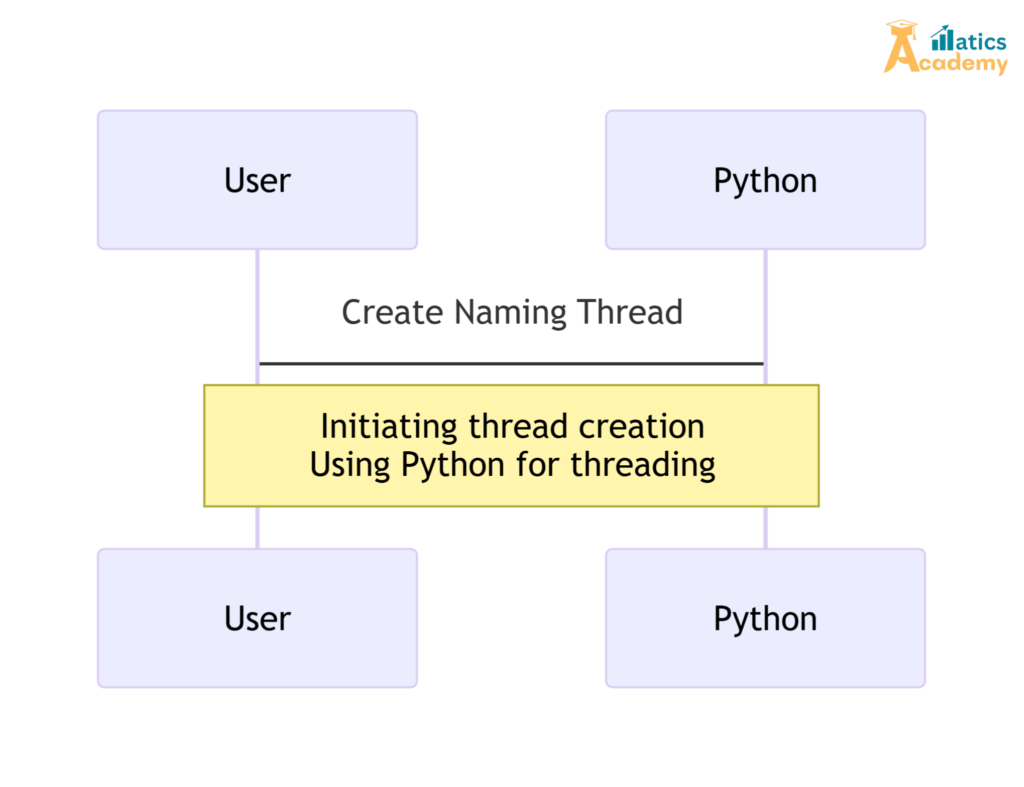
Why Name Threads in Python?
- Debugging Made Easy: Named threads make log messages more informative, helping you pinpoint issues quickly.
- Improved Readability: Descriptive thread names clearly communicate the role of each thread in the application.
- Efficient Monitoring: Tools that track threads display their names, making it easier to monitor performance and behavior.
How to Name a Threads in Python
Python provides the name
property to assign or modify thread names. Here’s how:
1. Assign Name at Creation: Use the name
argument while creating a thread:
import threading def task(): print(f"Running task in thread: {threading.current_thread().name}") thread = threading.Thread(target=task, name="DataProcessor") thread.start() thread.join()
2. Modify Thread Name Later: You can change a thread’s name after creation:
thread.name = "NewThreadName"
3. Get Current Thread Name: Use threading.current_thread().name
to retrieve the active thread’s name.
Best Practices for Name a Threads
- Be Descriptive: Use names that reflect the thread’s function, such as
FileDownloader
orUserInputHandler
. - Use Consistent Formatting: Follow a naming convention, like PascalCase or snake_case, for uniformity.
- Keep Names Short but Informative: Avoid overly long names to maintain clarity.
Mini Project(Name a thread): Thread Logger
In this project, we will simulate a logging system that logs the activity of different threads with their names. This will help demonstrate the significance of naming threads.
Mini Project Code
import threading def log_activity(thread_name): print(f"Thread {thread_name} started logging activity.") # Creating named threads thread1 = threading.Thread(target=log_activity, args=("Thread-1",)) thread2 = threading.Thread(target=log_activity, args=("Thread-2",)) # Start threads thread1.start() thread2.start() # Wait for threads to complete thread1.join() thread2.join()
Interview Questions for “TCS”
1. What is the significance of name a threads?
Answer: Naming a thread helps identify it easily in logs and debugging.
2. Can we change a thread’s name after it has started?
Answer: Yes, you can change the name of a thread using the setName()
method.
3. How do you assign a name to a threads?
Answer: You pass the name
parameter when creating the thread: thread = threading.Thread(name="CustomName")
.
4. What happens if you don’t name a threads?
Answer: Python assigns a default name to the thread, typically “Thread-N.”
5. Is naming threads mandatory in Python?
Answer: No, name a threads is optional. However, it can be useful for debugging and logging.
Conclusion
name a threads in Python is a simple yet powerful way to enhance the maintainability and debug-ability of your code. By leveraging the threading
module’s name
property, you can create multithreaded programs that are easier to understand and manage. Whether you’re building data processing tools, real-time applications, or multitasking systems, naming your threads is a best practice you shouldn’t overlook.