In today’s digital age, where data is growing exponentially, Data compression in Python has become a crucial technique to reduce file sizes and save storage space. Python provides a range of libraries and tools to implement data compression efficiently. In this article, we’ll explore what data compression is, its types, and how to perform it using Python with practical examples.
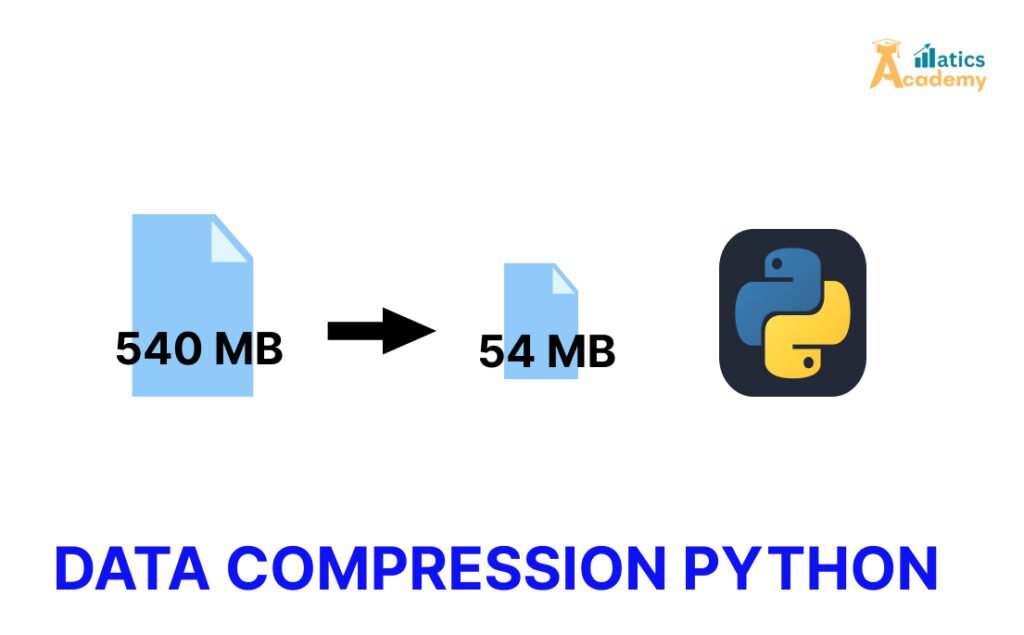
What is Data Compression in Python?
Data compression is the process of reducing the size of a file or dataset while preserving its original content or information as much as possible. It is widely used in various applications such as file storage, data transmission, and multimedia processing.
Key Benefits of Data Compression in Python :
- Storage Optimization: Reduces the storage space required for large files.
- Faster Data Transmission: Compressed files take less time to transfer over networks.
- Cost Efficiency: Saves bandwidth and storage costs.
Types of Data Compression in Python
1. Lossless Compression
Lossless compression reduces file size without losing any information. This is ideal for text files, code, or any data where accuracy is critical. Examples: ZIP files, PNG images.
2. Lossy Compression
Lossy compression achieves higher compression rates by discarding some data. This is commonly used for images, audio, and videos. Examples: MP3, JPEG.
How Data Compression Works
Compression works by identifying patterns or redundancies in data and representing them more efficiently. Some popular algorithms include:
- Huffman Coding: Reduces data redundancy by assigning shorter codes to frequently used characters.
- Run-Length Encoding (RLE): Compresses repetitive data sequences.
- Lempel-Ziv-Welch (LZW): The foundation of ZIP file compression.
Libraries for Data Compression
Python offers powerful libraries for data compression. Some of the most widely used ones are:
- zlib: Used for lossless compression.
- gzip: Compresses files in GZIP format.
- bz2: Provides high compression ratios.
- lzma: Implements LZMA and XZ compression.
- tarfile: Works with compressed tar archives.
Practical Examples of Data Compression in Python
1. Compressing Data Using zlib
The zlib
library provides fast and efficient compression for lossless data.
import zlib data = b"This is a sample text for compression using zlib." compressed_data = zlib.compress(data) decompressed_data = zlib.decompress(compressed_data) print("Original Data:", data) print("Compressed Data:", compressed_data) print("Decompressed Data:", decompressed_data)
2. File Compression with gzip
The gzip
library is used to compress and decompress files in GZIP format.
import gzip # Compressing a file with open("sample.txt", "rb") as f_in, gzip.open("sample.txt.gz", "wb") as f_out: f_out.writelines(f_in) # Decompressing a file with gzip.open("sample.txt.gz", "rb") as f_in, open("decompressed_sample.txt", "wb") as f_out: f_out.writelines(f_in)
3. Using bz2 for High Compression Ratios
The bz2
library is suitable for compressing data with high ratios.
import bz2 data = b"This is another example for bz2 compression." compressed = bz2.compress(data) decompressed = bz2.decompress(compressed) print("Compressed Data:", compressed) print("Decompressed Data:", decompressed)
4. Creating Compressed Archives with tarfile
The tarfile
module allows creating and extracting compressed tar archives.
import tarfile # Creating a compressed tar file with tarfile.open("archive.tar.gz", "w:gz") as tar: tar.add("sample.txt") # Extracting a tar file with tarfile.open("archive.tar.gz", "r:gz") as tar: tar.extractall()
Applications of Data Compression
- Data Storage: Compressing backup files, databases, and archives.
- Data Transmission: Reducing bandwidth usage in network communications.
- Multimedia: Compressing images, audio, and video for faster loading and streaming.
- Web Optimization: Reducing the size of web assets like CSS, JavaScript, and images for faster page loads.
Advantages and Disadvantages of Data Compression in Python
Advantages:
- Saves storage space.
- Reduces bandwidth requirements for data transmission.
- Improves application performance in constrained environments.
Disadvantages:
- Lossy compression results in irreversible data loss.
- Compression and decompression may require additional computation.
Tips for Efficient Data Compression in Python
- Choose the Right Algorithm: Select a compression method based on your use case and data type.
- Understand the Trade-offs: Lossy compression offers smaller sizes but at the cost of data quality.
- Test Compression Ratios: Experiment with different libraries to achieve optimal results.
Conclusion
Data compression in Python is an essential skill for developers working with large datasets, files, or multimedia. Python makes it incredibly simple to implement data compression using built-in libraries. Whether you’re compressing files for storage or optimizing data for transmission, understanding the basics of compression can greatly enhance your efficiency.
INTERVIEW QUESTIONS
1. What is the Difference Between Lossless and Lossy Compression?
Company: Amazon
Answer:
Example: JPEG images.
Lossless Compression: Reduces file size without losing any data. The original data can be fully reconstructed.
Example: ZIP files.
Lossy Compression: Reduces file size by removing some data, which leads to a loss in quality but reduces the size more effectively.
2. How Does Run-Length Encoding (RLE) Work?
Company: Microsoft
Answer:
Run-Length Encoding (RLE) is a simple compression method that stores sequences of repeated characters as a single character followed by its count.
Example:
- Input:
"AAAABBBCCDAA"
- Output:
"4A3B2C1D2A"
3. Explain Huffman Coding with an Example in Data Compression in Python
Company: Google
Answer:
- Huffman Coding is a lossless data compression algorithm that uses variable-length codes for encoding characters, with shorter codes assigned to more frequent characters.
- The process involves creating a binary tree where:
- Characters are stored as leaf nodes.
- Nodes with the lowest frequencies are combined to form internal nodes until only one node remains (the root).
Example:
- For the input
"ABRACADABRA"
, the frequency of characters are:A:5, B:2, R:2, C:1, D:1
Using Huffman coding, we would create a tree where A
gets the shortest binary code, like 0
, and other characters get longer codes, such as 10
, 110
, etc.
4. What Are the Advantages and Disadvantages of Lossy Compression?
Company: Infosys
Answer:
Advantages:
- Smaller File Sizes: Ideal for reducing file sizes, especially for images, videos, and audio.
- Faster Transmission: With smaller file sizes, it becomes easier to transmit or upload data.
Disadvantages:
Irreversibility: Once compressed, lossy files cannot be fully restored to their original state.
Loss of Quality: Data is lost, so the file quality may degrade, making it less suitable for applications that require high accuracy.
5. Implement a Basic Data Compression in Python Algorithm
Company: TCS
Answer:
def rle_compress(input_string): compressed = "" count = 1 for i in range(1, len(input_string)): if input_string[i] == input_string[i - 1]: count += 1 else: compressed += str(count) + input_string[i - 1] count = 1 compressed += str(count) + input_string[-1] return compressed input_string = "AAAABBBCCDAA" print(rle_compress(input_string)) # Output: "4A3B2C1D2A"
QUIZZES
Data Compression in python Quiz
Question
Your answer:
Correct answer:
Your Answers