Introduction
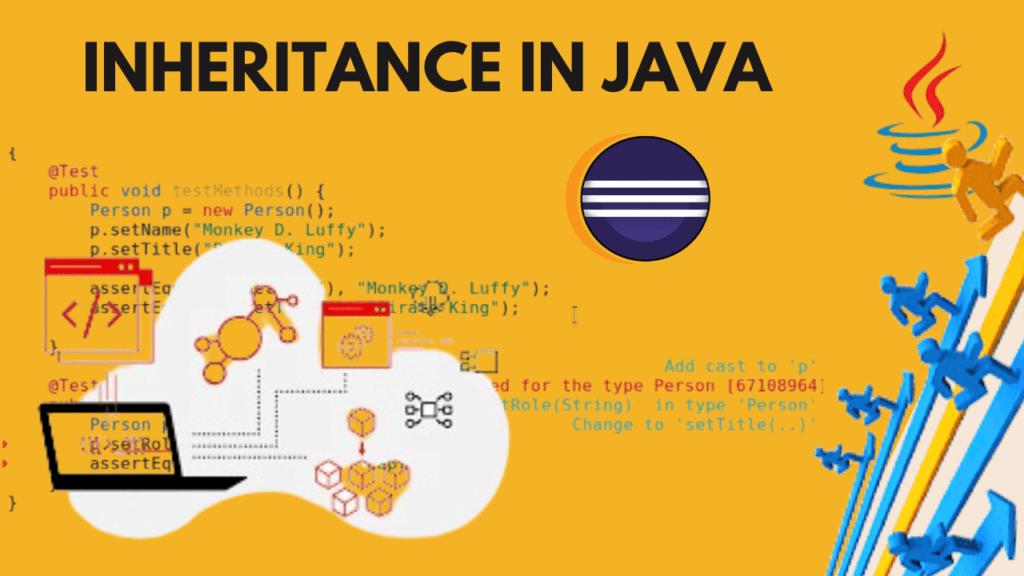
Why Study Inheritance in Java?
Inheritance is a fundamental concept in Java that enables one class to inherit properties and methods from another class. This feature promotes code reusability and establishes a clear relationship between classes. Understanding inheritance significantly enhances your programming skills and prepares you for advanced object-oriented programming topics.
What Will Be Covered?
You will learn about:
- The definition of inheritance.
- Types of inheritance: single, multilevel, hierarchical, and multiple (via interfaces).
- Practical examples for each type.
- Key takeaways and practice exercises.
What is Inheritance?
Inheritance allows a new class (subclass) to inherit attributes and behaviors (methods) from an existing class (superclass). This mechanism enables developers to extend existing code without modifying it, fostering a modular design.
Types of Inheritance
1.Single Inheritance
In single inheritance, one subclass inherits from one superclass. This straightforward hierarchy simplifies understanding.
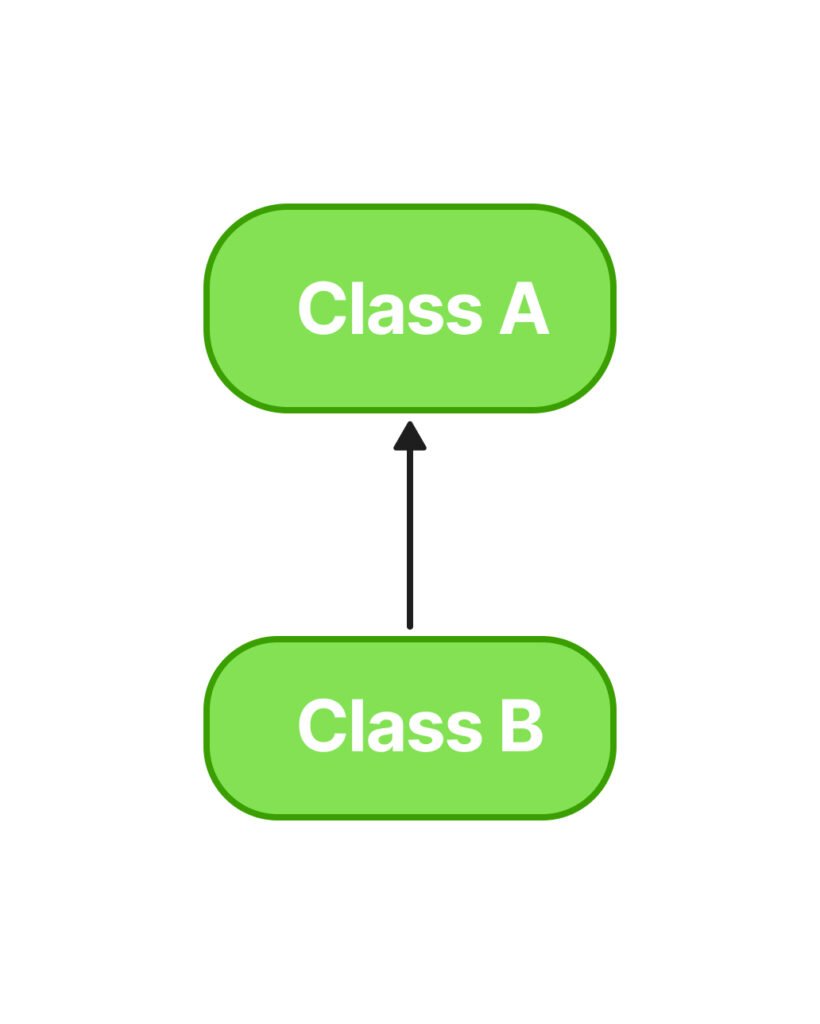
Example:
javaCopy codeclass Animal { void sound() { System.out.println("Some sound"); } } class Dog extends Animal { void sound() { System.out.println("Bark"); } }
2. Multilevel Inheritance
In multilevel inheritance, a subclass inherits from a superclass, and another subclass inherits from that subclass, forming a chain of inheritance.
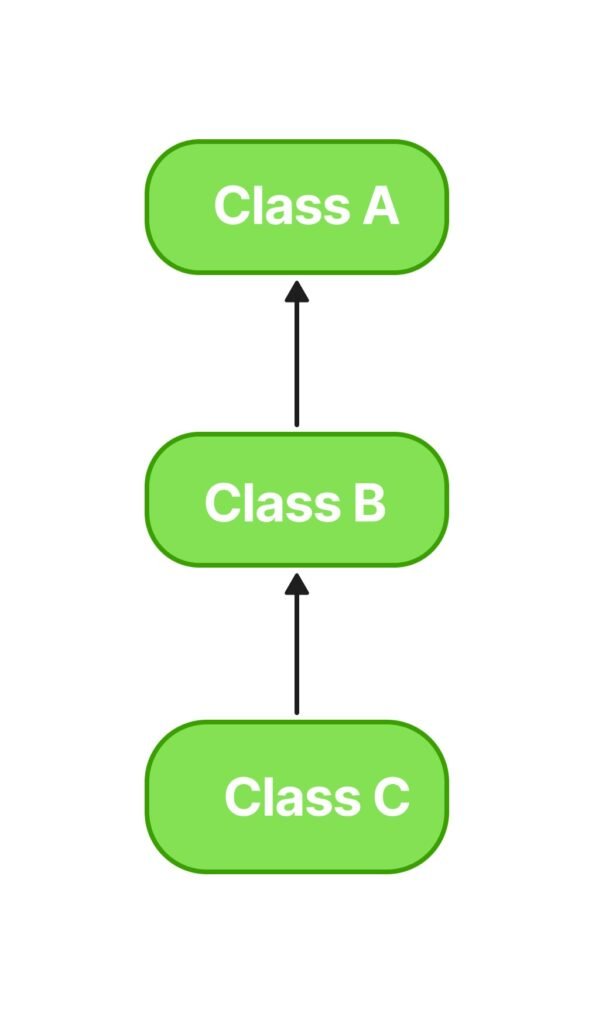
Example:
javaCopy codeclass Animal { void sound() { System.out.println("Some sound"); } } class Dog extends Animal { void sound() { System.out.println("Bark"); } } class Puppy extends Dog { void sound() { System.out.println("Yap"); } }
3. Hierarchical Inheritance
Hierarchical inheritance occurs when multiple subclasses inherit from a single superclass. This structure allows shared properties among subclasses.
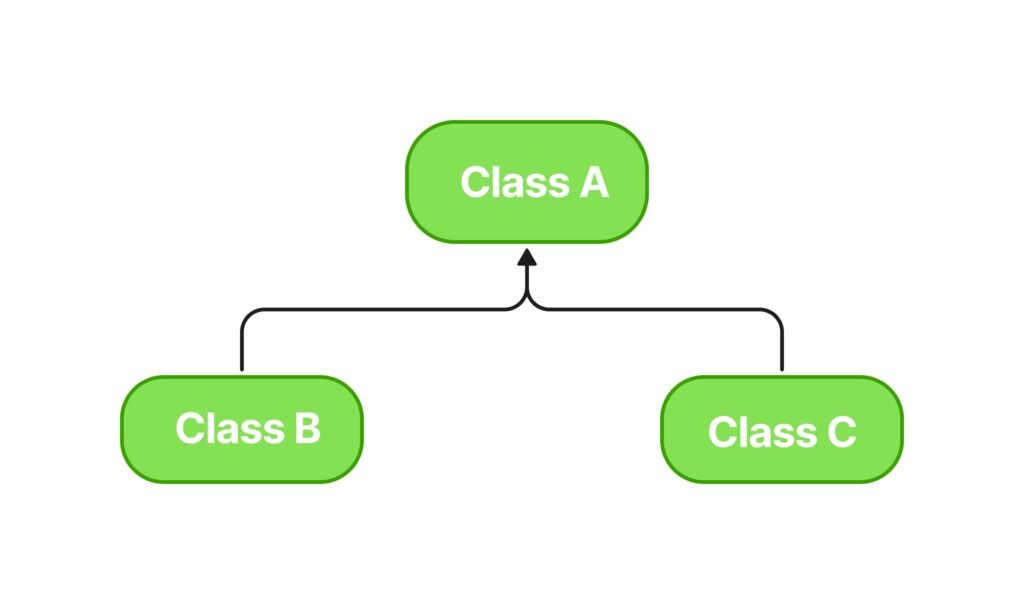
Example:
javaCopy codeclass Animal { void sound() { System.out.println("Some sound"); } } class Dog extends Animal { void sound() { System.out.println("Bark"); } } class Cat extends Animal { void sound() { System.out.println("Meow"); } }
4. Multiple Inheritance (via Interfaces)
Java does not support multiple inheritance directly through classes to avoid ambiguity. However, a class can implement multiple interfaces, allowing it to inherit methods from more than one source.
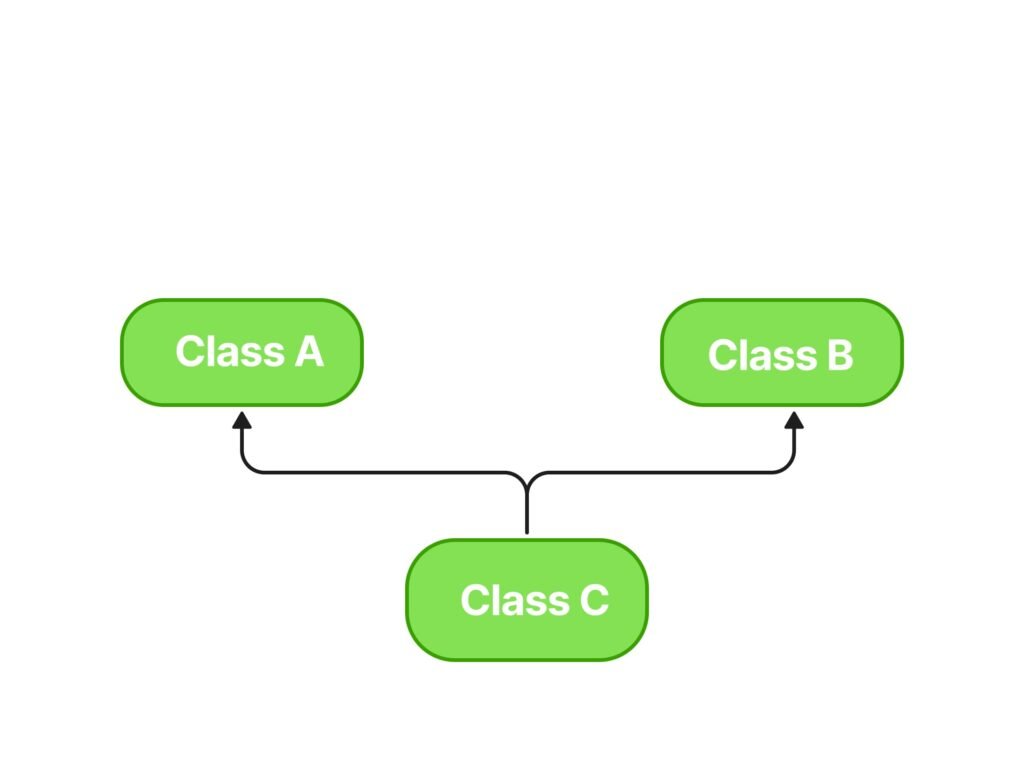
Example:
// First interface interface Printable { void print(); } // Second interface interface Showable { void show(); } // Implementing both interfaces in a single class class Document implements Printable, Showable { // Implementing the print method from Printable public void print() { System.out.println("Printing the document..."); } // Implementing the show method from Showable public void show() { System.out.println("Showing the document..."); } } public class MultipleInheritanceExample { public static void main(String[] args) { Document doc = new Document(); doc.print(); // Calls the print method doc.show(); // Calls the show method } }
5.Hybrid Inheritance (via Interfaces)
Hybrid inheritance is a mix of different inheritance types. Since Java does not support hybrid inheritance with classes, it can be achieved using interfaces.
interface A { void methodA(); } interface B extends A { void methodB(); } interface C extends A { void methodC(); } class D implements B, C { public void methodA() { System.out.println("Method A"); } public void methodB() { System.out.println("Method B"); } public void methodC() { System.out.println("Method C"); } } public class HybridInheritanceExample { public static void main(String[] args) { D obj = new D(); obj.methodA(); obj.methodB(); obj.methodC(); } }
The Diamond Problem in Java
The diamond problem occurs when a class inherits from two classes that share a common superclass, leading to ambiguity in method resolution.
Example of the Diamond Problem (Not Allowed in Java)
class A { void show() { System.out.println("A's method"); } } class B extends A {} class C extends A {} class D extends B, C { // Error: Java does not support multiple inheritance void show() { System.out.println("D's method"); } }
Summary
Inheritance allows classes to share functionality, enhancing code reusability and organization. The main types of inheritance are single, multilevel, hierarchical, and multiple (via interfaces). Each type provides a unique way to structure your classes, making it easier to maintain and scale your programs.
Learning Outcomes
After completing this topic, you will be able to:
- Define and differentiate the types of inheritance .
- Implement inheritance concepts in your applications.
- Understand the significance of inheritance in object-oriented programming.
Common Interview Questions
1.At [TechSolutions Inc.], we value code efficiency. Can you explain what inheritance is and why it’s important in Java?
Inheritance is a mechanism that allows a new class to inherit properties and methods from an existing class, promoting code reusability and logical class relationships.
2.[InnovateSoft] is working on a project that requires a hierarchical class structure. Could you explain single inheritance and give an example?
In single inheritance, one subclass directly inherits from one superclass. Example:
class Animal { void sound() { System.out.println("Some sound"); } } class Dog extends Animal { void sound() { System.out.println("Bark"); } }
3.[SkyNet Innovations] values the modular design of code. What is the role of the super
keyword in inheritance?
The super
keyword accesses superclass methods and properties, often used in method overriding and to call superclass constructors.
4.[FusionTech], method overriding is frequently used. How do you override a method in Java, and why is method overriding essential in inheritance?
Method overriding is done by defining a method in the subclass with the same signature as the superclass method. It allows subclasses to provide specific implementations.
5.[NextGen Software], code maintenance is crucial. What benefits does inheritance provide in Java applications?
Inheritance promotes code reuse, reduces redundancy, improves maintainability, and establishes a clear hierarchy in code design.
Practice Exercises
- Exercise 1: Create a class hierarchy using multilevel inheritance. Implement methods that demonstrate method overriding.
- Exercise 2: Write a program that uses hierarchical inheritance to model different animals.
- Quiz: Develop a short quiz to test your understanding of inheritance concepts.
Additional Resources
- Books: “Effective Java” by Joshua Bloch
- Articles: “Understanding Java Inheritance” on Oracle’s website
- Tutorials: Java inheritance tutorials on Maticsacademy.
Take Quiz:
Question
Your answer:
Correct answer:
Your Answers