When you create an object in Java, you need to set up its initial state. This is where a constructor comes in. Think of a constructor as a special method that runs when you create an object. It sets up the object’s default values and any other requirements. In this article, we’ll explore what constructors are, why they’re essential, and how to use them with simple examples.
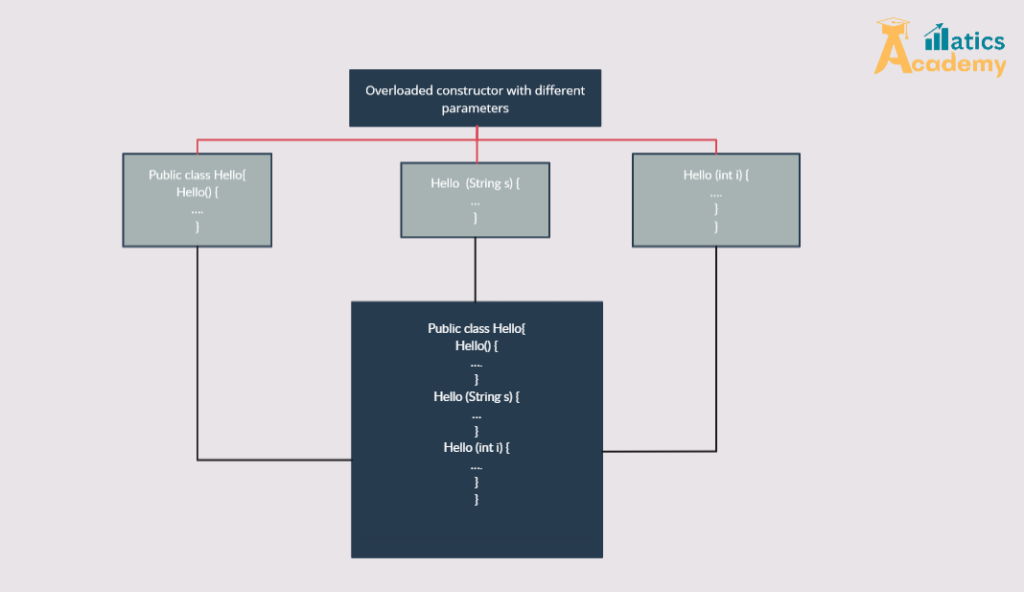
What is a Constructor in Java?
In Java, a constructor is a block of code called automatically when an object is created. The main job of the constructor is to initialize the object. It’s named after the class and doesn’t have a return type.
Types of Constructors in Java
1. Default Constructor
This is a no-argument constructor that Java provides if you don’t define any constructors. It sets default values for the object.
public class Car { private String model; private int year; // Default constructor public Car() { model = "Unknown"; year = 0; System.out.println("Default car created with model: " + model + ", year: " + year); } public static void main(String[] args) { Car myCar = new Car(); // Calls the default constructor } }
default-constructor-quiz
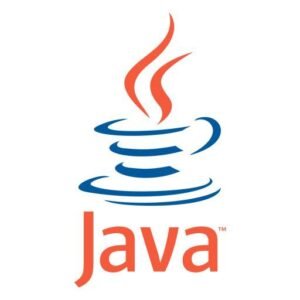
Question
Your answer:
Correct answer:
Your Answers
2. Parameterized Constructor
This constructor takes parameters to initialize an object with specific values.
private String model; private int year; // Parameterized constructor public Car(String model, int year) { this.model = model; this.year = year; System.out.println("Car created with model: " + model + ", year: " + year); } public static void main(String[] args) { Car myCar = new Car("Toyota", 2022); // Calls the parameterized constructor } }
parameterized-constructors in java
Question
Your answer:
Correct answer:
Your Answers
Why Use Constructors?
Constructors are essential because they:
- Set up an object with default or initial values.
- Ensure an object is usable right from creation.
- Make code more readable and maintainable by setting clear initial conditions for objects.
Creating a Constructor in Java
To create a constructor, write it with the same name as the class. Here’s a unique example to illustrate:
Example: CoffeeMachine Class
Imagine you’re creating a CoffeeMachine class. The constructor will help set the initial amount of coffee, water, and milk needed to make a coffee.
public class CoffeeMachine { private int coffee; private int water; private int milk; // Constructor with parameters public CoffeeMachine(int coffee, int water, int milk) { this.coffee = coffee; this.water = water; this.milk = milk; System.out.println("Coffee Machine is ready to serve!"); } public void makeCoffee() { if(coffee > 0 && water > 0 && milk > 0) { System.out.println("Enjoy your coffee!"); } else { System.out.println("Refill ingredients to make coffee."); } } }
In this example, when a CoffeeMachine object is created, the constructor assigns values to coffee, water, and milk. The machine can then check if these ingredients are available whenever we call makeCoffee()
.
Benefits of Using Constructors
- Automatic Initialization: Avoids the risk of using an object without setting essential data.
- Code Clarity: Anyone reading the code knows how objects are created and what initial data is needed.
- Efficiency: Saves time by automating initial setup, reducing repetitive code.
Tips for Using Constructors in Java
- Avoid Long Parameter Lists: Too many parameters can make code hard to read. Consider using setter methods if more initialization is needed.
2. Be Clear with Default Values: If your class has a default constructor, make sure it sets up meaningful default value
3. Use this
Keyword: Use this
to clarify which variables belong to the object.
Common Misconceptions About Constructors
- Constructors Aren’t Methods: They look like methods, but they don’t have a return type and only initialize objects.
- You Don’t Have to Write a Constructor: Java automatically provides a default constructor if none are defined.
Beginner Project: Library Book Management
Objective: Use constructors to manage book details in a small library application.
Project Description: Create a Book
class that stores information about books in a library, like title, author, and year published. Write two constructors: a default constructor that initializes each book with default values and a parameterized constructor that allows setting specific values for each new book.
Code:
public class Book { private String title; private String author; private int yearPublished; // Default Constructor public Book() { this.title = "Unknown Title"; this.author = "Unknown Author"; this.yearPublished = 0; } // Parameterized Constructor public Book(String title, String author, int yearPublished) { this.title = title; this.author = author; this.yearPublished = yearPublished; } public void displayBookInfo() { System.out.println("Title: " + title + ", Author: " + author + ", Year: " + yearPublished); } public static void main(String[] args) { // Using the default constructor Book book1 = new Book(); book1.displayBookInfo(); // Using the parameterized constructor Book book2 = new Book("Java Fundamentals", "John Doe", 2022); book2.displayBookInfo(); } }
Main Parts
- Default Constructor:
- This constructor doesn’t take any information. It sets the book’s title, author, and year to default values (like “Unknown Title,” “Unknown Author,” and
0
). This is helpful when we don’t have details for a book yet but still want to create it.
- This constructor doesn’t take any information. It sets the book’s title, author, and year to default values (like “Unknown Title,” “Unknown Author,” and
- Parameterized Constructor:
- This constructor takes information (title, author, and year) and sets the book’s details to those specific values. It’s useful when we know the details and want to create a book with accurate information right away.
- Display Method:
- The
displayBookInfo()
method shows the details of each book, helping us check that the constructors worked as expected.
- The
Interview Questions
1. Company: Zoho
Question: What are constructors in Java, and can you explain the difference between a default and parameterized constructor?
Answer: Constructors initialize objects. A default constructor has no parameters and provides default values, while a parameterized constructor allows custom initialization by taking arguments.
2. Company: TCS
Question: How does using constructors in Java enhance code clarity, especially when initializing objects with essential data?
Answer: Constructors enforce object initialization with required data, ensuring fields are set and improving readability and reliability.
3. Company: Infosys
Question: Can you discuss how the this
keyword is used within constructors and provide an example?
Answer: The this
keyword refers to the current instance. In constructors, it distinguishes class fields from parameters with the same name.
4. Company: Capgemini
Question: Why does Java automatically provide a default constructor if none is defined, and when might you choose to override it?
Answer: Java provides a default constructor for object creation. Override it when you need custom initialization or specific parameter handling.
Why This is Useful
This project shows how constructors work in Java. The default constructor sets up a basic book, while the parameterized one lets us set exact details. This makes managing data easier and is a basic but important concept in programming.