Introduction to Java Stream API
Why Study Java Stream API ?
Java Stream API is essential for efficient data processing. Introduced in Java 8, the Stream API allows developers to work with collections of data in a functional style, making code more readable, concise, and maintainable.
What Will Be Covered in Java Stream API ?
This guide will explore:
- The basics of the Stream API, including what streams are and how they work.
- Key operations in the Stream API, including filtering, mapping, and reduction, are essential.
- Practical examples of Stream API usage in Java applications.
- Best practices for using streams efficiently in real-world applications.
Explanations and Examples
What Is the Java Stream API?
The Stream API is a powerful feature in Java that allows processing sequences of data in a declarative and functional manner. Essentially, streams are not data structures; they are abstractions over sequences of elements, like those found in collections (lists, sets, etc.), arrays, or I/O channels.
Creating a Stream
In Java, streams are typically created from collections or arrays.
import java.util.Arrays; import java.util.List; import java.util.stream.Stream; public class StreamExample { public static void main(String[] args) { List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5); Stream<Integer> numberStream = numbers.stream(); } }
Stream Operations
The Stream API has two types of operations: intermediate and terminal.
- Intermediate Operations: These operations transform a stream but don’t terminate it. Examples include
filter()
,map()
, andsorted()
. - Terminal Operations: These complete the pipeline of operations. Examples include
forEach()
,collect()
, andreduce()
.
1. Intermediate Operators
These operators transform elements in the stream.
1.1 filter(Predicate<T>)
– Filters elements based on a condition
import java.util.*; import java.util.stream.*; public class StreamExample { public static void main(String[] args) { List<Integer> numbers = Arrays.asList(10, 15, 20, 25, 30); // Filter numbers greater than 20 List<Integer> result = numbers.stream() .filter(n -> n > 20) .collect(Collectors.toList()); System.out.println(result); // Output: [25, 30] } }
2. Terminal Operators
These operators consume the stream and produce a result.
2.1 collect(Collectors.toList())
– Collect elements into a list
import java.util.*; import java.util.stream.*; public class StreamExample { public static void main(String[] args) { List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5); // Convert stream to list List<Integer> squaredNumbers = numbers.stream() .map(n -> n * n) .collect(Collectors.toList()); System.out.println(squaredNumbers); // Output: [1, 4, 9, 16, 25] } }
Summary of Operators

Example Code for Filtering and Mapping:
import java.util.Arrays; import java.util.List; import java.util.stream.Collectors; public class StreamExample { public static void main(String[] args) { List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David"); // Filtering names with more than 3 characters and converting to uppercase List<String> filteredNames = names.stream() .filter(name -> name.length() > 3) .map(String::toUpperCase) .collect(Collectors.toList()); System.out.println(filteredNames); // Output: [ALICE, CHARLIE, DAVID] } }
In this example, the filter()
method selects names with more than 3 characters, and map()
converts them to uppercase.
Reduction Operations
The reduce() method is often used to accumulate stream elements into a single result. For instance, we could use reduce() to sum a list of integers:
import java.util.Arrays; import java.util.List; public class ReductionExample { public static void main(String[] args) { List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5); int sum = numbers.stream().reduce(0, Integer::sum); System.out.println("Sum: " + sum); // Output: Sum: 15 } }
Visual Aids
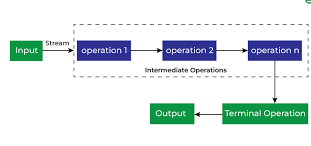
Summary
The Java Stream API offers a modern and functional approach to data processing. In summary, the main takeaways include understanding the differences between intermediate and terminal operations, learning how to use filtering, mapping, and reducing, and knowing best practices for efficient stream usage.
Learning Outcomes
After completing this topic, learners will be able to:
- Understand the structure and purpose of the Stream API in Java.
- Apply intermediate and terminal operations to process data.
- Write concise and functional code for data processing tasks in Java.
Common Interview Questions
What is the difference between map()
and filter()
in the Stream API?
map()
transforms each element, while filter()
only selects elements that meet a certain condition.
Explain how reduce()
works in Java streams.
The reduce()
method accumulates elements into a single result based on a provided binary operation.
What are the advantages of using the Stream API?
It simplifies code, supports parallel processing, and enables a functional programming approach.
Can streams process data in parallel?
Yes, by using parallelStream()
, which divides the task across multiple threads for faster processing.
What is the main difference between streams and collections?
Streams process data on demand and don’t store it, while collections store data in memory.
Practice Exercises
- Filtering and Mapping: Create a list of integers, filter out even numbers, and multiply each odd number by 2.
- Reduction Challenge: Given a list of strings, find the total length of all strings combined using
reduce()
. - Complex Pipeline: Build a pipeline that sorts a list of names alphabetically, removes duplicates, and converts them to lowercase.
Solutions
- Example solution for Filtering and Mapping:javaCopy code
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5); List<Integer> result = numbers.stream() .filter(n -> n % 2 != 0) .map(n -> n * 2) .collect(Collectors.toList()); System.out.println(result);
Additional Resources
- Books: Java 8 in Action by Raoul-Gabriel Urma for in-depth Stream API insights.
- Articles: Oracle’s official Java Stream documentation provides a complete reference.
- Online Tutorials: “Java Streams API” on Java Code Geeks includes practical examples.
Java Stream API
Question
Your answer:
Correct answer:
Your Answers