Introduction
JUnit is a popular open-source testing framework for Java, essential for verifying code functionality and ensuring software quality. With JUnit, developers can create automated test cases, improve reliability, and catch bugs early in the development cycle. Understanding and implementing JUnit in Java helps enhance software quality and maintain code stability.
What is JUnit?
JUnit is a unit testing framework for the Java programming language. It supports test-driven development (TDD), enabling developers to write tests before implementing code. JUnit provides simple annotations and assertions to define and validate tests, making it easier to organize and execute automated tests.
Benefits of JUnit:
- Supports test-driven development (TDD).
- Enables faster debugging and reduces manual testing.
- Helps maintain code quality over time.
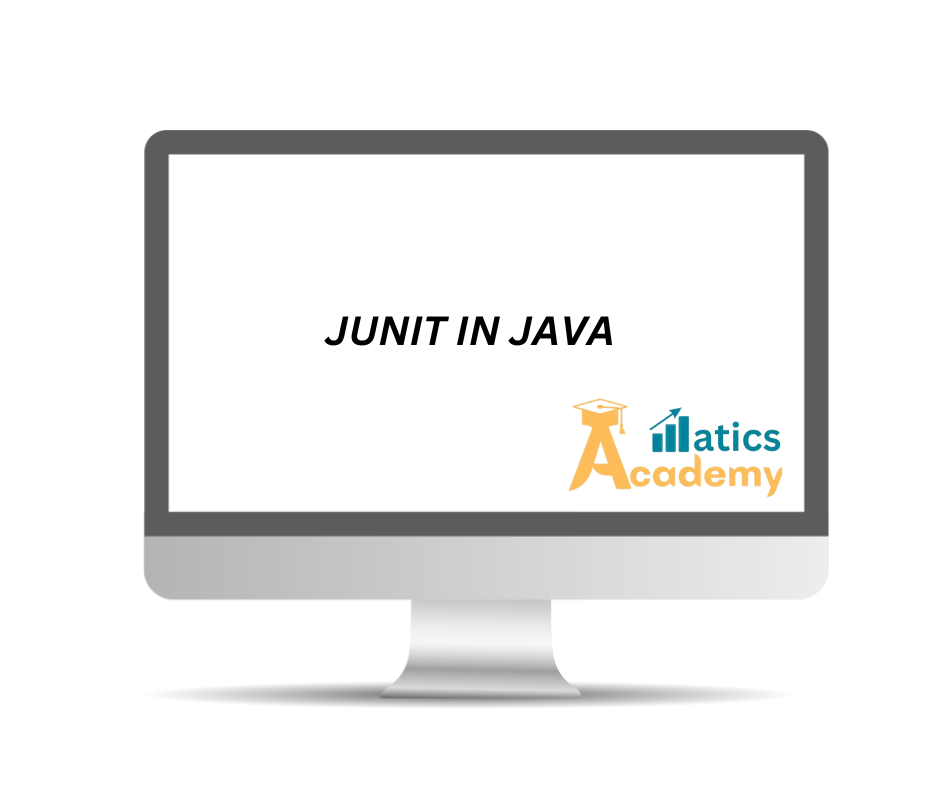
Core Features of JUnit
- Annotations: Key to organizing test cases, making them easy to understand and execute.
- Assertions: Validate the correctness of code by comparing expected and actual results.
- Test Runners: Execute test cases and provide test reports.
JUnit Annotations
- @Test: Marks a method as a test case.
- @Before: Executes code before each test case, useful for setup.
- @After: Runs after each test, ideal for cleanup.
- @BeforeClass and @AfterClass: Execute once before or after all test cases in a class, useful for initializing shared resources.
Example: Setting Up JUnit in Java
To get started with JUnit, add JUnit to your project, either through Maven, Gradle, or by downloading the JUnit JAR file.
- Maven Dependency
XML <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.13.2</version> <scope>test</scope> </dependency>
Basic Test Case Example:
import org.junit.Test; import static org.junit.Assert.assertEquals; public class CalculatorTest { @Test public void testAddition() { Calculator calculator = new Calculator(); int result = calculator.add(5, 3); assertEquals(8, result); } }
This code tests the add
method of the Calculator
class, verifying that 5 + 3
equals 8
.
Writing Effective JUnit Tests
- Use Assertions: Validate outcomes with assertions like
assertEquals
,assertTrue
, andassertFalse
. - Isolate Tests: Each test case should be independent and avoid relying on the results of other tests.
- Use Setup and Teardown Methods: Initialize resources in
@Before
methods and release them in@After
.
Example:
import org.junit.After; import org.junit.Before; import org.junit.Test; public class SampleTest { @Before public void setUp() { System.out.println("Setting up resources."); } @Test public void testExample() { assertEquals(10, 5 + 5); } @After public void tearDown() { System.out.println("Releasing resources."); } }
Advanced JUnit Features
- Parameterized Tests: Run the same test with different input values.
- Exception Testing: Verify that certain methods throw expected exceptions using
@Test(expected = Exception.class)
. - Timeouts: Use
@Test(timeout=1000)
to ensure a test completes within a specified time.
Common Interview Questions.
What is JUnit, and why is it important?
Company: Infosys
Answer: JUnit is a Java testing framework that enables unit testing for code reliability and maintenance. It automates tests for fast feedback, reducing errors and supporting agile practices like continuous integration.
2. Explain different JUnit annotations and their uses.
Company: Cognizant
Answer:
- @Test: Marks a method as a test.
- @BeforeEach and @AfterEach: Run before and after each test, respectively, for setup and cleanup.
- @BeforeAll and @AfterAll: Run once before/after all tests, typically for shared resources.
- @Disabled: Skips the specified test.
3. How does Test-Driven Development (TDD) relate to JUnit?
Company: Wipro
Answer: TDD uses JUnit to write tests before code, focusing on test-first development. JUnit tests guide code creation, ensuring that new code meets requirements and is defect-free.
4. What is the difference between @BeforeEach
and @BeforeAll
in JUnit?
Company: TCS
Answer: @BeforeEach
runs before each test, resetting conditions individually, while @BeforeAll
runs once before all tests, useful for initializing shared resources. @BeforeAll
must be static.
5. How does JUnit support exception testing?
Company: Accenture
Answer: JUnit’s @Test(expected = Exception.class)
checks if a method throws the expected exception. Alternatively, assertThrows()
verifies specific exception handling, ensuring code robustness under error conditions.
Practice Exercises
Write a JUnit test case for a method that calculates the factorial of a number.
Let’s assume a simple MathUtils
class with a factorial(int n)
method:
public class MathUtils { public int factorial(int n) { if (n < 0) { throw new IllegalArgumentException("Factorial is not defined for negative numbers."); } int result = 1; for (int i = 1; i <= n; i++) { result *= i; } return result; } }
Implement and test exception handling in a divide
method of a calculator class.
This JUnit test case checks normal and edge cases, including handling invalid input (negative numbers).
import static org.junit.jupiter.api.Assertions.*; import org.junit.jupiter.api.Test; public class MathUtilsTest { MathUtils mathUtils = new MathUtils(); @Test public void testFactorialPositiveNumber() { assertEquals(120, mathUtils.factorial(5), "Factorial of 5 should be 120"); } @Test public void testFactorialZero() { assertEquals(1, mathUtils.factorial(0), "Factorial of 0 should be 1"); } @Test public void testFactorialNegativeNumber() { assertThrows(IllegalArgumentException.class, () -> mathUtils.factorial(-1), "Factorial of negative numbers should throw IllegalArgumentException"); } }
Additional Resources
- JUnit Documentation
- Effective Unit Testing by Lasse Koskel
PLAY WITH JAVA..!!
Question
Your answer:
Correct answer:
Your Answers