Introduction to Lambda Expressions In Java
Lambda expressions, introduced in Java 8, provide a way to write concise and readable code for implementing functional interfaces (interfaces with a single abstract method). By using lambda expressions, developers can simplify their code, especially in cases where small instances of functional interfaces are required, like in event handling, threading, or collection processing. Lambda expressions enable Java to support functional programming, which can lead to more efficient and modular code.
Key Features of Lambda Expressions In Java
- Conciseness: Lambdas reduce the boilerplate code associated with anonymous inner classes.
- Readability: Code becomes more readable and expressive.
- Functional Interface Compatibility: Lambdas can only be used with functional interfaces (interfaces with one abstract method), such as
Runnable
,Callable
, andComparator
.
Syntax of Lambda Expressions In Java
The basic syntax of a lambda expression in Java is:
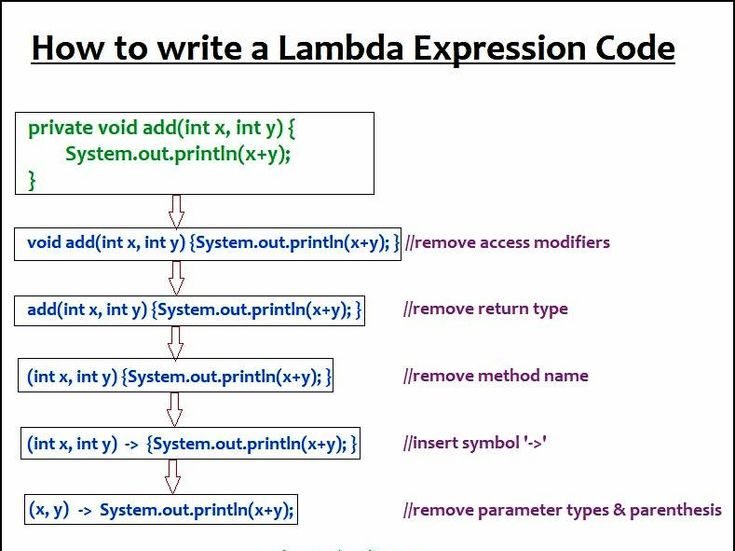
(parameters) -> expression
Or, for a block of statements:
(parameters) -> { statements }
- Parameters: Represent the inputs to the lambda function. Parentheses are optional if there is only one parameter.
- Arrow Token (
->
): Separates parameters and the body of the lambda expression. - Body: Defines the action of the lambda expression, either a single expression or a block of statements.
Examples of Lambda Syntax
Single parameter with single expression:
x -> x * 2
Multiple parameters with a block of statements:
(a, b) -> { int sum = a + b; return sum; }
Usage of Lambda Expressions
Lambda expressions are particularly useful in functional interfaces and make code more concise and readable. Here are some common use cases for lambda expressions:
1. Using Lambda Expressions with Functional Interfaces
- Functional interfaces like
Runnable
,Callable
,Comparator
, and custom functional interfaces are compatible with lambda expressions.
Example with Runnable
:
Runnable runnable = () -> System.out.println("Running in a lambda expression!"); new Thread(runnable).start();
2. Using Lambda Expressions in Collections
Lambda expressions simplify operations on collections, such as sorting, filtering, or mapping.
Example with Comparator
for Sorting:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie"); names.sort((a, b) -> a.compareTo(b));
3. Using Lambda Expressions with Stream API
The Stream API in Java works seamlessly with lambda expressions, making it easy to perform complex operations on collections, like filtering, mapping, and reducing.
Example with Stream Filtering:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5); List<Integer> evenNumbers = numbers.stream() .filter(n -> n % 2 == 0) .collect(Collectors.toList());
Detailed Example Code
Here is a full example showing lambda expressions used with a custom functional interface and collection operations:
import java.util.*; import java.util.function.Predicate; public class LambdaExample { public static void main(String[] args) { // Example 1: Custom Functional Interface MyFunctionalInterface messagePrinter = (message) -> System.out.println("Message: " + message); messagePrinter.printMessage("Hello from Lambda!"); // Example 2: Sorting a list of names with lambda List<String> names = Arrays.asList("Alice", "Eve", "Bob", "Charlie"); names.sort((a, b) -> a.compareTo(b)); System.out.println("Sorted names: " + names); // Example 3: Filtering with Predicate functional interface List<Integer> numbers = Arrays.asList(10, 15, 20, 25, 30); List<Integer> filteredNumbers = filterList(numbers, n -> n > 20); System.out.println("Filtered Numbers: " + filteredNumbers); } // Custom Functional Interface @FunctionalInterface interface MyFunctionalInterface { void printMessage(String message); } // Method using Predicate functional interface with lambda public static List<Integer> filterList(List<Integer> list, Predicate<Integer> predicate) { List<Integer> filteredList = new ArrayList<>(); for (Integer i : list) { if (predicate.test(i)) { filteredList.add(i); } } return filteredList; } }
Explanation of the Code:
- Custom Functional Interface:
MyFunctionalInterface
with a single abstract methodprintMessage
. - Sorting with Lambda: Using a lambda expression to define the sorting behavior for a list of names.
- Filtering with Lambda and Predicate: The
filterList
method uses aPredicate
functional interface with a lambda to filter numbers greater than 20.
Best Practices for Using Lambda Expressions
- Use Lambdas for Short and Simple Operations: Lambdas are ideal for short operations; for more complex logic, consider using methods or classes.
- Avoid Overusing Lambdas: Lambdas can reduce readability if overused, especially for complex logic.
- Use
Method References
When Possible: If the lambda expression is simply calling a method, consider using method references (ClassName::methodName
) for improved readability.
Advantages of Lambda Expressions
- Improved Code Readability: Reduces boilerplate code, making logic easier to follow.
- Functional Programming: Lambdas enable functional programming in Java, allowing more concise and modular code.
- Enhanced Productivity: Lambda expressions make it easier to work with Java’s collection and Stream APIs, resulting in more efficient data processing.
Limitations of Lambda Expressions
- Debugging Complexity: Lambdas can make stack traces harder to interpret, complicating debugging.
- Limited to Functional Interfaces: Lambdas are only compatible with functional interfaces, so they cannot replace classes with multiple abstract methods.
Learning Outcomes
- Understand the syntax and structure of lambda expressions.
- Implement lambda expressions with functional interfaces.
- Use lambda expressions effectively in collection and Stream API operations.
- Recognize best practices and limitations when using lambda expressions in Java.
Practice Exercises
- Exercise 1: Write a lambda expression to calculate the square of a number and print the result.
- Exercise 2: Use a lambda expression with
Comparator
to sort a list of strings by length. - Exercise 3: Write a lambda expression using
Predicate
to filter out strings shorter than 5 characters from a list.
Additional Resources
- Books: “Java 8 in Action” by Raoul-Gabriel Urma, Mario Fusco, and Alan Mycroft.
- Online: Oracle’s official Java documentation on lambda expressions and functional interfaces.
Lambda Expressions In Java
Question
Your answer:
Correct answer:
Your Answers