Introduction
In Python, dictionaries are versatile data structures that store key-value pairs. When working with dictionaries, it’s common to loop through their contents to extract keys, values, or both. Understanding how to Python loop through dictionaries efficiently can help you manage and manipulate data effectively in Python programming.
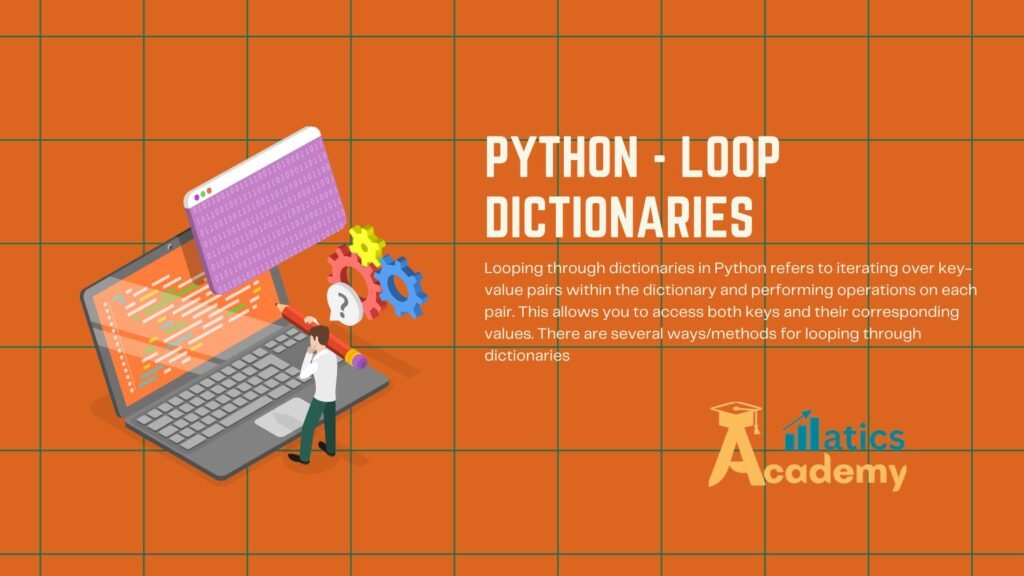
Understanding Python Dictionaries
A dictionary in Python is a collection that is unordered, mutable, and indexed. Each key is unique and maps to a specific value. For instance:b
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'}
This dictionary has three keys (name
,age
, andcity
) with their corresponding values.
Why Loop Through Dictionaries?
Looping through dictionaries allows you to:
- Access individual keys or values for processing.
- Perform operations like filtering or updating.
- Generate insights from stored data, especially in data-heavy applications.
How to Loop Through Dictionaries in Python
1. Loop Through Keys
Use a simple for
loop to iterate through dictionary keys:
my_dict = {'name': 'Alice', 'age': 25, 'city': 'New York'} for key in my_dict: print(key)
name age city
2. Loop Through Values
Use the .values()
method to access the dictionary’s values:
for value in my_dict.values(): print(value)
3. Loop Through Key-Value Pairs
The .items()
method allows you to loop through both keys and values simultaneously:
for key, value in my_dict.items(): print(f"{key}: {value}")
4. Using Enumerate with Dictionaries
If you need the index while iterating, combine enumerate()
with .items()
:
pythonCopy codefor index, (key, value) in enumerate(my_dict.items()): print(f"{index}: {key} -> {value}"
Advanced Dictionary Looping Techniques
Filtering While Looping
You can filter items in a dictionary during iteration:
filtered_dict = {key: value for key, value in my_dict.items() if isinstance(value, int)} print(filtered_dict)
Nested Dictionaries
When working with nested dictionaries, use nested loops:
nested_dict = {'user1': {'name': 'Alice', 'age': 25}, 'user2': {'name': 'Bob', 'age': 30}} for outer_key, inner_dict in nested_dict.items(): print(f"{outer_key}:") for inner_key, inner_value in inner_dict.items(): print(f" {inner_key}: {inner_value}")
Common Mistakes and Best Practices
Mistake 1: Modifying a Dictionary While Iterating
Avoid altering a dictionary directly during iteration. Instead, create a copy using .copy()
or a new dictionary for changes.
Mistake 2: Using Non-Hashable Keys
Ensure all keys are hashable (immutable types like strings, integers, or tuples).
Best Practice 1: Use Dictionary Comprehensions
For concise and readable looping, leverage dictionary comprehensions where appropriate:
squared_values = {key: value**2 for key, value in {'a': 2, 'b': 3}.items()} print(squared_values)
Best Practice 2: Use Built-In Methods
Rely on built-in dictionary methods (keys()
, values()
, items()
) for clarity and efficiency.
Key Takeaways
- Looping through dictionaries is essential for processing key-value pairs.
- Python provides multiple methods to iterate through keys, values, or both.
- Avoid common pitfalls and adopt best practices for efficient coding.
Mastering how to loop through dictionaries in Python empowers you to handle data with precision and efficiency. Whether you’re filtering, processing nested dictionaries, or applying advanced techniques, Python’s flexibility ensures a smooth experience. Practice these concepts to refine your skills and elevate your programming expertise.
Suggested Practice
- Write a Python program that prints all keys of a dictionary that have integer values greater than 10.
- Create a nested dictionary and extract all values from the inner dictionaries.
- Use dictionary comprehension to swap keys and values in a given dictionary.
By understanding and implementing these techniques, you’ll become proficient at managing Python dictionaries in real-world scenarios!
Interview Questions
1. What are Python dictionaries, and why are they useful?(Google)
Python dictionaries are unordered collections of key-value pairs that allow fast retrieval, insertion, and deletion of values based on unique keys. They are useful for mapping relationships, such as storing configurations, organizing data, or implementing lookups.
2. Can dictionary keys be of any data type? (Microsoft)
No, dictionary keys must be immutable (e.g., strings, numbers, tuples) and hashable, meaning their value cannot change during the lifetime of the dictionary.
3. How does Python handle duplicate keys in a dictionary? (Amazon)
If a dictionary contains duplicate keys, the last assignment overwrites the previous one. Python dictionaries do not allow true duplicates.
4. What is the time complexity of accessing an item in a dictionary? (Meta (FB))
The average time complexity for accessing an item in a dictionary is O(1) but it can degrade to O(n) in the worst case due to hash collisions.
5. What happens when you attempt to access a non-existent key in a dictionary? (Tesla)
Accessing a non-existent key directly raises a KeyError
. However, using the .get()
method returns None
or a specified default value.
Test Your Knowledge: python – loop dictionaries !
Question
Your answer:
Correct answer:
Your Answers
learn more about Python Dictionaries try this