Math Operations in Python provides a powerful and flexible platform , making it a favorite choice for developers, data scientists, and engineers. This article explores the various ways you can handle math in Python, from basic arithmetic to advanced mathematical operations.
1. Introduction to Python’s Math Capabilities
Python has built-in operators for basic arithmetic, as well as the math
module for more advanced functions. Libraries like NumPy and SciPy extend these capabilities to support high-level mathematical and statistical computations.
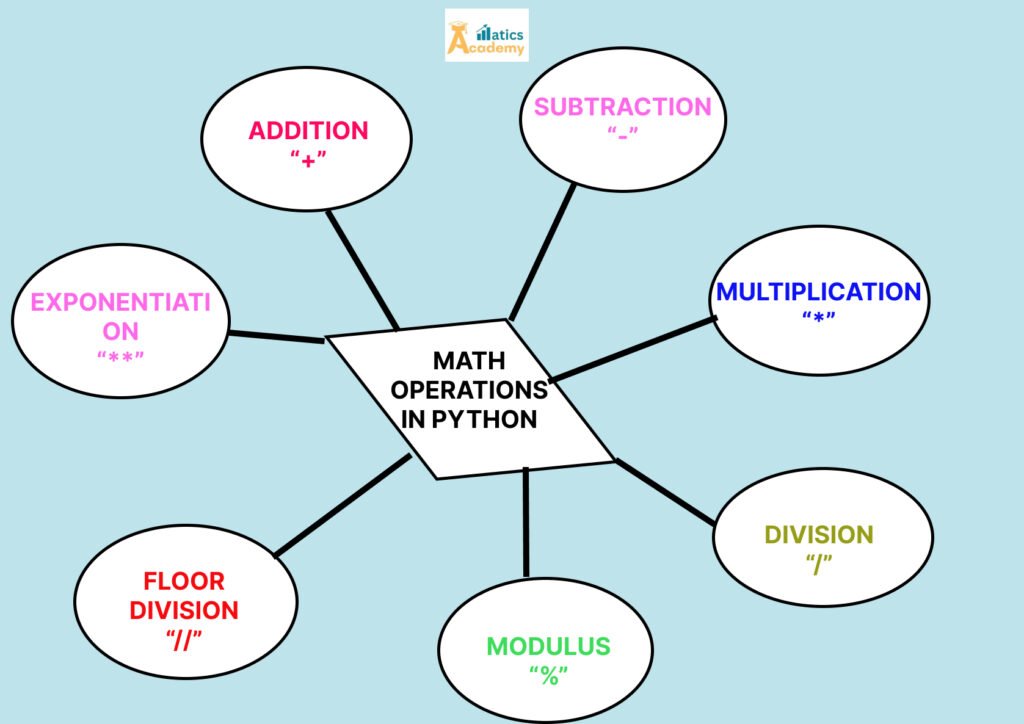
2. Basic Arithmetic in Python
basic Math Operations in Python include:
- Addition:
+
- Subtraction:
-
- Multiplication:
*
- Division:
/
- Floor Division:
//
- Modulus (Remainder):
%
- Exponentiation:
**
Example:
a = 10 b = 3 print("Addition:", a + b) # Output: 13 print("Subtraction:", a - b) # Output: 7 print("Multiplication:", a * b) # Output: 30 print("Division:", a / b) # Output: 3.3333... print("Floor Division:", a // b) # Output: 3 print("Modulus:", a % b) # Output: 1 print("Exponentiation:", a ** b) # Output: 1000
3. Using the math
Module
The math
module provides advanced mathematical functions. You can import it using:
import math
Commonly Used Functions
- Square Root:
math.sqrt(x)
- Power:
math.pow(x, y)
- Logarithm:
math.log(x)
(natural log),math.log10(x)
(base-10 log) - Trigonometry:
math.sin(x)
,math.cos(x)
,math.tan(x)
- Constants:
math.pi
,math.e
Example:
import math x = 16 print("Square Root:", math.sqrt(x)) # Output: 4.0 print("Power:", math.pow(2, 3)) # Output: 8.0 print("Natural Logarithm:", math.log(2)) # Output: 0.6931... print("Pi Value:", math.pi) # Output: 3.14159...
4. Rounding Numbers
Python offers several ways to round numbers:
round(x, n)
: Roundsx
ton
decimal places.math.ceil(x)
: Rounds up to the nearest integer.math.floor(x)
: Rounds down to the nearest integer.
Example:
import math num = 7.45 print("Round:", round(num, 1)) # Output: 7.5 print("Ceil:", math.ceil(num)) # Output: 8 print("Floor:", math.floor(num)) # Output: 7
5. Working with Random Numbers
The random
module is used to generate random numbers:
random.random()
: Generates a random float between 0 and 1.random.randint(a, b)
: Generates a random integer betweena
andb
.random.choice(sequence)
: Selects a random element from a sequence.
Example:
import random print("Random Float:", random.random()) # Example Output: 0.7352 print("Random Integer:", random.randint(1, 10)) # Example Output: 5
6. Advanced Math with NumPy
For advanced Math Operations in Python, NumPy is a powerful library that provides support for arrays, matrices, and high-level math functions.
Key Features of NumPy:
- Element-wise operations on arrays.
- Linear algebra functions.
- Statistical computations.
Example:
import numpy as np arr = np.array([1, 2, 3, 4]) print("Array:", arr) print("Square Root:", np.sqrt(arr)) # Output: [1. 1.414 1.732 2.] print("Mean:", np.mean(arr)) # Output: 2.5 print("Sum:", np.sum(arr)) # Output: 10
7. Complex Numbers in Python
Python natively supports complex numbers using the syntax a + bj
:
- Real part:
.real
- Imaginary part:
.imag
Example:
c = 3 + 4j print("Complex Number:", c) # Output: (3+4j) print("Real Part:", c.real) # Output: 3.0 print("Imaginary Part:", c.imag) # Output: 4.0
8. Error Handling in Math Operations
When performing Math Operations in Python, errors like division by zero or invalid input can occur. Python provides tools to handle these gracefully.
Example:
try: result = 10 / 0 except ZeroDivisionError: print("Error: Division by zero!")
9. Real-World Applications
Math Operations in Python are widely used in:
- Data Analysis: For statistical computations.
- Machine Learning: For linear algebra and calculus.
- Gaming: For physics-based motion.
- Finance: For calculating interest and risk.
10. Conclusion
Python offers robust tools for Math Operations, from the basics to advanced computations with external libraries like NumPy. Understanding and leveraging these tools can help you solve complex mathematical problems efficiently.
Interview Questions
1. Question from Google
Q: How would you calculate the factorial of a number in Python using the math
module? Can you write a sample code snippet?
Expected Answer: Use math.factorial()
to compute the factorial of a number.
Sample Code:
import math num = 5 print("Factorial:", math.factorial(num)) # Output: 120
2. Question from Microsoft
Q: What is the difference between /
(division) and //
(floor division) in Python? Give an example where floor division would be useful.
Expected Answer:
/
performs regular division and returns a float.//
performs floor division and returns the largest integer less than or equal to the result.
Example:
print(7 / 2) # Output: 3.5 print(7 // 2) # Output: 3
3. Question from Amazon
Q: How would you generate a random number between 1 and 100 using Python? Write a code snippet to demonstrate.
Expected Answer: Use the random.randint()
function from the random
module.
Sample Code :
import random print(random.randint(1, 100)) # Output: Random number between 1 and 100
4. Question from Meta (formerly Facebook)
Q:Â How can you calculate the sine of an angle in Python? Write a code snippet to find the sine of 30 degrees using Math Operations in Python.
Expected Answer: Use the math.sin()
function, ensuring the angle is in radians. Convert degrees to radians using math.radians()
.
Sample Code:
import math angle_deg = 30 angle_rad = math.radians(angle_deg) print("Sine of 30 degrees:", math.sin(angle_rad)) # Output: 0.5
5. Question from Tesla
Q: Explain the difference between math.sqrt()
 and math.pow()
 for calculating square roots in Math Operations in Python. Provide an example.
Expected Answer:
math.sqrt(x)
calculates the square root directly.math.pow(x, 0.5)
calculates the square root by raisingx
to the power of 0.5.
Example:
import math x = 16 print("Using sqrt():", math.sqrt(x)) # Output: 4.0 print("Using pow():", math.pow(x, 0.5)) # Output: 4.0
Quizzes
Let’s Play With Math Operations in Python
Question
Your answer:
Correct answer:
Your Answers