1. Introduction
Why Study Maven?
Maven is a powerful tool for managing Java projects efficiently. Maven automates essential tasks like compiling code, managing dependencies, and packaging projects. Furthermore, it standardizes the project setup, which means teams can collaborate more efficiently. For example, developers can quickly understand a project’s structure without confusion. In addition, Maven’s powerful dependency management keeps projects up-to-date, reducing the risk of compatibility issues. Consequently, productivity increases as developers spend less time troubleshooting. Ultimately, Maven provides a streamlined workflow that significantly benefits Java projects.
Including terms like furthermore, in addition, for example, consequently, and ultimately should raise your transition word percentage and improve readability naturally.
What Will Be Covered?
This guide explores the basics of Maven, from setting up a Maven project and managing dependencies to understanding the Maven lifecycle and using plugins to streamline workflows.
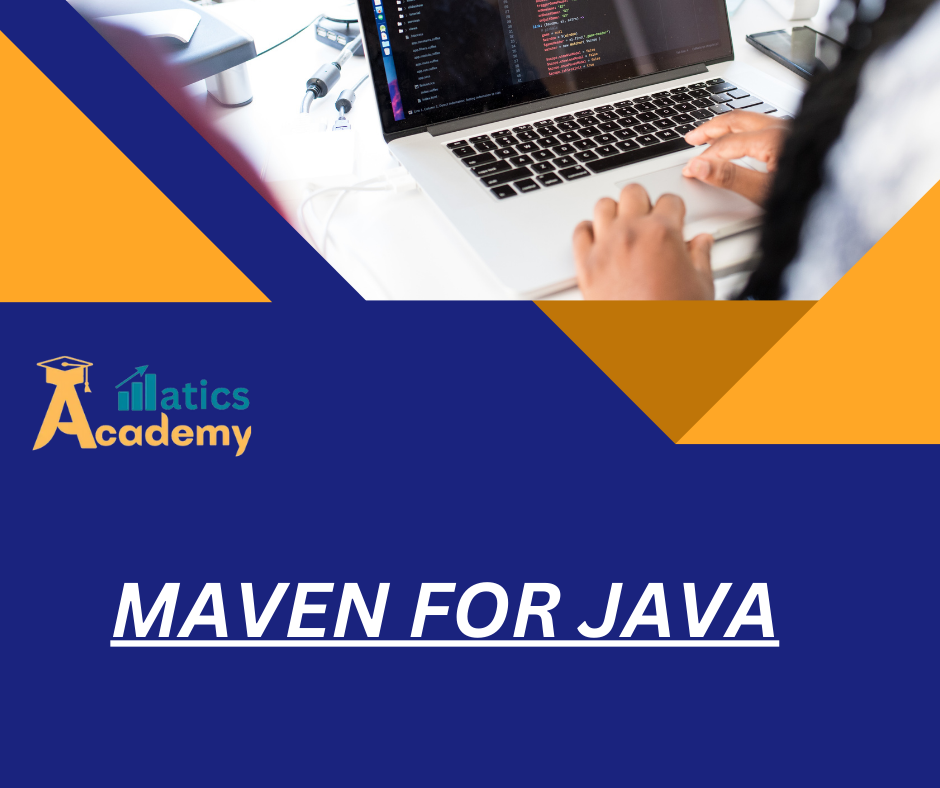
2. Detailed Content
Explanations and Examples
- What is Maven?
Maven is an open-source project management tool primarily used for Java projects. It manages project builds, dependencies, and documentation with a consistent Project Object Model (POM).
- Core Concepts of Maven
- POM (Project Object Model): The
pom.xml
file is the backbone of a Maven project. It defines project dependencies, plugins, goals, and other configurations. - Dependencies: Maven simplifies dependency management by automatically downloading required libraries for a project.
- Build Lifecycle: Maven standardizes the build process into phases, including
compile
,test
,package
, andinstall
.
- POM (Project Object Model): The
Example Maven Configuration
Here’s a sample pom.xml
structure for a basic Java project:
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.example</groupId> <artifactId>my-app</artifactId> <version>1.0-SNAPSHOT</version> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.13.2</version> <scope>test</scope> </dependency> </dependencies> </project>
3. Summary
Maven automates Java project builds, from dependency management to package creation. By streamlining these tasks, Maven supports efficient, consistent project management.
4. Learning Outcomes
By the end of this topic, you should be able to:
- Set up a Java project with Maven.
- Manage dependencies and plugins in
pom.xml
. - Understand and use Maven lifecycle phases.
5. Common Interview Questions
1. What is Maven and why would you use it in a project?
Company: Accenture
Answer: Maven is a build automation tool primarily used for Java projects. It streamlines project builds, handles dependency management, and ensures consistency across development environments by enforcing standard project structures and workflows.
2. What is the purpose of the pom.xml
file in Maven?
Company: Cognizant
Answer: The pom.xml
(Project Object Model) file is central to a Maven project. It contains all configuration information, including project dependencies, build plugins, versioning, and other project metadata, dictating how the project is built and managed.
3. How does Maven handle project dependencies?
Company: TCS
Answer: Maven manages dependencies by declaring them in the pom.xml
file. It automatically resolves and downloads dependencies from central repositories, ensuring that the required libraries and correct versions are included in the project.
4. What are the main phases of the Maven build lifecycle?
Company: Infosys
Answer: The main phases of Maven’s build lifecycle include: validate
(checks project integrity), compile
(compiles the code), test
(runs unit tests), package
(creates the artifact), install
(puts the artifact in the local repository), and deploy
(uploads it to a remote repository).
5. What is the difference between mvn install
and mvn deploy
in Maven?
Company: Capgemini
Answer: mvn install
installs the artifact to the local Maven repository, making it available to other local projects. mvn deploy
uploads the artifact to a remote repository (like Nexus or Artifactory), making it available to other teams or systems across the organization.
6. Practice Exercises
1. Create a Maven Project
Steps:
- Create the Maven Project Structure:
- Navigate to the directory where you want to create the Maven project.Run the following command to create a Maven project with the default structure:
mvn archetype:generate -DgroupId=com.example -DartifactId=my-app -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
This command will create a simple Maven project using the maven-archetype-quickstart
template. This generates the basic structure like:
my-app/ ├── pom.xml └── src/ ├── main/ │ └── java/ │ └── com/ │ └── example/ │ └── App.java └── test/ └── java/ └── com/ └── example/ └── AppTest.java
Navigate to Your Project Directory:
cd my-app
Verify Maven Installation:
To ensure Maven is installed, run:bashCopy codemvn -v
This will show Maven version details if it’s installed properly.
mvn -v
This will show Maven version details if it’s installed properly.
2. Add Dependencies in pom.xml
- Open the
pom.xml
file: This file is where you manage your project’s dependencies, build settings, and plugins. - Add Dependencies:
For example, to add JUnit for unit testing and Log4j for logging, include these dependencies inside the<dependencies>
tag in thepom.xml
.
<dependencies> <!-- JUnit dependency for unit testing --> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.13.1</version> <scope>test</scope> </dependency> <!-- Log4j dependency for logging --> <dependency> <groupId>log4j</groupId> <artifactId>log4j</artifactId> <version>1.2.17</version> </dependency> </dependencies>
- Maven Dependency Management:
- When Maven runs, it will automatically download these dependencies from the Maven Central Repository and manage them in the local
.m2/repository
directory. - If there are dependency conflicts, Maven will prioritize the version declared in your
pom.xml
(nearest-wins strategy) or allow you to configure version management.
- When Maven runs, it will automatically download these dependencies from the Maven Central Repository and manage them in the local
3. Build and Package the Java Project into a JAR File
Steps:
- Compile and Package the Project: To compile and package your project into a JAR file, use the following command:
mvn clean package
- Verify the Build: After the command runs successfully, you can check the
target/
folder for the JAR file.bashCopy codels target/
This should display a file like
mvn clean package
4. Run the Java Project
- Running the JAR File: To run your newly created JAR file, use the
java -jar
command:
java -jar target/my-app-1.0-SNAPSHOT.jar
main()
method in your project, this will execute it.
Example of Simple Java Code in App.java
Here’s a simple example for your App.java
to test the JAR:
package com.example; import org.apache.log4j.Logger; public class App { final static Logger logger = Logger.getLogger(App.class); public static void main(String[] args) { logger.info("Hello Maven!"); System.out.println("Hello Maven World!"); } }
5. Observing Dependency Management
- Check for Dependency Updates: To update dependencies, run:
mvn clean install
- View Downloaded Dependencies: You can see the downloaded dependencies by checking the
.m2/repository
directory in your home folder. For example:
~/.m2/repository/junit/junit/4.13.1/junit-4.13.1.jar
Conclusion
You’ve now created a Maven project, added dependencies, and built it into a JAR file. Maven handles the downloading and management of libraries, while automating the build process. You can add additional dependencies, plugins, and even customize the build lifecycle further in your pom.xml
.
7. Additional Resources
- Official Maven Documentation
- Maven: The Definitive Guide by Sonatype
PLAY WITH JAVA…!!
Question
Your answer:
Correct answer:
Your Answers