Introduction
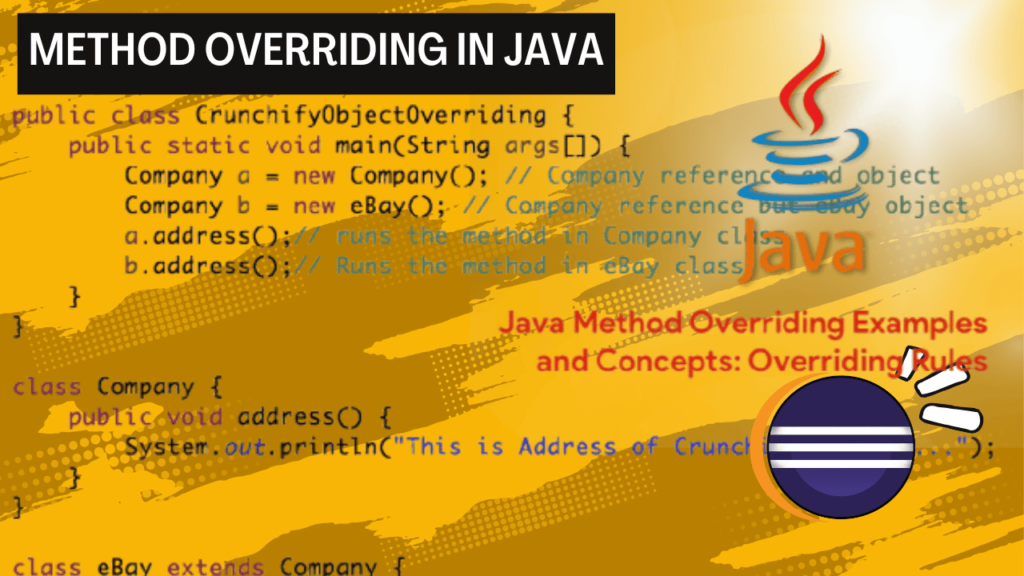
Why Study Method Overriding?
overriding is a core concept in Java’s object-oriented programming (OOP) paradigm. It allows a subclass to provide a specific implementation of a method already defined in its superclass. Understanding this concept enhances your programming skills and is essential for career growth in software development
What Will Be Covered?
This guide introduces the principles of overriding, provides examples, and covers its applications. You’ll also explore common interview questions and related practice exercises.
What is Method overriding?
Explanations and Examples
Overriding allows a subclass to implement a unique version of an inherited method, essential for achieving runtime polymorphism.
// Superclass class Animal { // Method to be overridden public void sound() { System.out.println("Animal makes a sound"); } } // Subclass class Dog extends Animal { // Overriding the sound method @Override public void sound() { System.out.println("Dog barks"); } } public class MethodOverridingExample { public static void main(String[] args) { // Create an Animal object Animal myAnimal = new Animal(); myAnimal.sound(); // Calls the Animal class sound method // Create a Dog object Dog myDog = new Dog(); myDog.sound(); // Calls the Dog class sound method (overridden method) } }
In this example, the Dog
class overrides the sound
method of the Animal
class. When you call myDog.sound()
, it invokes the Dog
‘s implementation.
The Role of the super
Keyword in Overriding
The super
keyword is used in method overriding to call the superclass version of the overridden method.
class Animal { public void sound() { System.out.println("Animal makes a sound"); } } class Dog extends Animal { @Override public void sound() { super.sound(); // Calls the method from the superclass System.out.println("Dog barks"); } } public class SuperKeywordExample { public static void main(String[] args) { Dog myDog = new Dog(); myDog.sound(); } }
Output
Animal makes a sound Dog barks
Summary
It is a powerful feature in Java that allows subclasses to implement specific behaviors for methods defined in superclasses. It promotes code reusability and enhances flexibility in your programming.
Learning Outcomes
After completing this topic, you’ll be able to:
- Explain what method overriding does.
- Implement it through practical examples.
- Recognize its advantages in OOP.
Common Interview Questions
1.What is method overriding in Java, and why is it important?[Google]
Overriding allows a subclass to provide a specific implementation of a method defined in its superclass. It’s essential for runtime polymorphism and enables flexibility by allowing subclasses to adapt inherited behaviors.
2.How does method overriding support runtime polymorphism in Java?[Microsoft]
At runtime, Java uses dynamic method dispatch to determine which overridden method to call based on the object instance, allowing for behavior adaptation in subclasses.
3.What role does the @Override
annotation play in overriding?[Amazon]
The @Override
annotation is optional but recommended. It helps the compiler verify that a method indeed overrides a superclass method, reducing errors.
4.Explain the difference between overloading and overriding.[Meta]
Overloading occurs within the same class and allows different method signatures with the same name, while overriding allows a subclass to provide a specific implementation of a superclass method.
5.What are the requirements for a method to be overridden in Java?[IBM]
The method must have the same name, parameters, and return type (or a covariant return type) as in the superclass and should not be final
or static
.
Practice Exercises
- Coding Challenge: Create a superclass named
Vehicle
with a methodmove()
. Override this method in subclasses likeCar
andBicycle
. Implement different behaviors for each.
Additional Resources
- Effective Java by Joshua Bloch
- Java: The Complete Reference by Herbert Schildt
- Online tutorials on Java
- Oracle’s Java Documentation
Take Quiz:
Question
Your answer:
Correct answer:
Your Answers