Java networking allows developers to build applications that communicate over the internet. Utilizing Java’s powerful networking capabilities, you can create robust applications that connect users and share data seamlessly. This guide explores the fundamentals of networking, key components involved, and provides practical examples to get started.
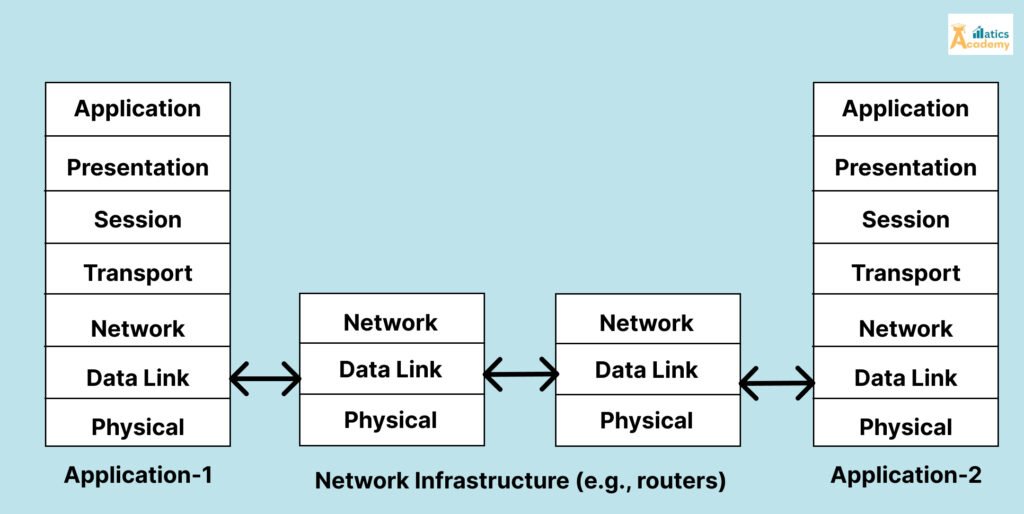
Understanding Java Networking
Standard networking protocols like TCP/IP form the foundation of networking. Java applications use these protocols to send and receive data over a network. The Java Networking API simplifies networking by providing classes and interfaces for various network tasks.
Key Components of Java Networking
When working with networking , several key components play a crucial role:
1. Sockets
Sockets serve as endpoints for sending and receiving data between two machines. In Java, the Socket
class is used for creating client sockets, while the ServerSocket
class is utilized for server sockets.
2. IP Addresses and Ports
Every device connected to a network has a unique IP address, which helps identify it. In addition, ports are used to distinguish between different services running on a machine. Java provides the InetAddress
class to handle IP addresses.
3. Client-Server Model
In Java Networking commonly follows the client-server model. In this architecture, the client initiates requests to the server, which processes these requests and sends back responses. This model is essential for applications requiring reliable communication between users.
4. Protocols
Java networking supports various protocols, including TCP (Transmission Control Protocol) and UDP (User Datagram Protocol). TCP ensures reliable communication by establishing a connection before data transmission, while UDP offers faster, connectionless communication.

Getting Started with Java Networking
To create a simple Java networking application, follow these steps:
Step 1: Create the Server
Use the ServerSocket
class to create a server that listens for incoming client connections. Here’s a basic example:
import java.io.; import java.net.; public class SimpleServer { public static void main(String[] args) { try (ServerSocket serverSocket = new ServerSocket(12345)) { System.out.println("Server is listening on port 12345"); Socket socket = serverSocket.accept(); System.out.println("Client connected"); // Handle client communication // ... } catch (IOException e) { e.printStackTrace(); } } }
Step 2: Create the Client
Next, create a client application that connects to the server using the Socket
class. Here’s a simple client example:
import java.io.; import java.net.; public class SimpleClient { public static void main(String[] args) { try (Socket socket = new Socket("localhost", 12345)) { System.out.println("Connected to the server")// Handle server communication // ... } catch (IOException e) { e.printStackTrace(); } }}
Step 3: Testing Your Application
Run the server and client applications in separate terminals. You will observe the connection established between the two, showcasing the power of networking.
Best Practices for Java Networking
To ensure your networking applications are efficient and secure, follow these best practices:
- Error Handling: Implement robust error handling to manage exceptions effectively.
- Resource Management: Always close sockets and streams after use to prevent resource leaks.
- Security: Use secure protocols, such as SSL/TLS, to protect sensitive data during transmission.
- Concurrency: For server applications, consider using threading to handle multiple client connections simultaneously.
Conclusion
Run the server and client applications in separate terminals. You will observe the connection established between the two, demonstrating the power of networking.
Interview Questions
1. What are the main classes used for networking in Java, and what are their purposes?
- Company: TCS, Accenture, Cognizant
- Explanation: You should be familiar with key networking classes in Java, including
Socket
,ServerSocket
,URL
, andHttpURLConnection
. TheSocket
class lets you connect to a specific IP address and port, whileServerSocket
listens for incoming connections. You can useURL
andHttpURLConnection
to access and manage data over HTTP.
// Establishing a connection to a server Socket socket = new Socket("example.com", 80); System.out.println("Connected to server: " + socket.isConnected()); socket.close();
2. Explain the client-server model in Java networking. How do you create a simple client-server application using Socket
and ServerSocket
?
- Company: Wipro, Infosys, Capgemini
- Explanation: This question tests your understanding of the client-server architecture, where the client initiates a request and the server responds. Show how to set up a basic client-server application using
Socket
(client-side) andServerSocket
(server-side).
// Server ServerSocket serverSocket = new ServerSocket(1234); Socket clientSocket = serverSocket.accept(); // Waits for client System.out.println("Client connected!"); clientSocket.close(); serverSocket.close(); // Client Socket socket = new Socket("localhost", 1234); System.out.println("Connected to server!"); socket.close();
3. What is the difference between TCP
and UDP
, and how can you implement each in Java?
- Company: Oracle, Microsoft, HCL
- Explanation: You should explain
TCP
(Transmission Control Protocol) andUDP
(User Datagram Protocol).TCP
is connection-oriented and ensures reliable data transfer, whileUDP
is connectionless and faster but doesn’t guarantee delivery. You can use theSocket
class for TCP andDatagramSocket
for UDP in Java.
// UDP Server DatagramSocket serverSocket = new DatagramSocket(9876); byte[] receiveData = new byte[1024]; DatagramPacket receivePacket = new DatagramPacket(receiveData, receiveData.length); serverSocket.receive(receivePacket); System.out.println("Received: " + new String(receivePacket.getData())); serverSocket.close();
4. How can you send HTTP requests in Java, and what are the advantages of using the HttpURLConnection
class?
- Company: Amazon, Google, IBM
- Explanation: You may be asked about sending HTTP requests in Java, such as GET and POST. Use the
HttpURLConnection
class to manage HTTP connections. It lets you set request headers, handle input/output streams, and manage the HTTP response.
URL url = new URL("https://example.com/api/data"); HttpURLConnection connection = (HttpURLConnection) url.openConnection(); connection.setRequestMethod("GET"); int responseCode = connection.getResponseCode(); System.out.println("Response Code: " + responseCode); connection.disconnect();
5. How do you handle exceptions and timeouts in Java networking to make a program more robust?
- Company: JP Morgan Chase, Goldman Sachs, Deloitte
- Explanation: You may need to handle networking exceptions, such as
UnknownHostException
,SocketTimeoutException
, andIOException
. Set read and connect timeouts to handle slow or unresponsive servers gracefully.
try { Socket socket = new Socket(); socket.connect(new InetSocketAddress("example.com", 80), 5000); // 5-second timeout socket.setSoTimeout(3000); // Read timeout System.out.println("Connected to server"); socket.close(); } catch (UnknownHostException e) { System.out.println("Host could not be found"); } catch (SocketTimeoutException e) { System.out.println("Connection timed out"); } catch (IOException e) { System.out.println("An I/O error occurred"); }
Quizzes
Networking in Java Quiz
Question
Your answer:
Correct answer:
Your Answers