1. Introduction of operators in java
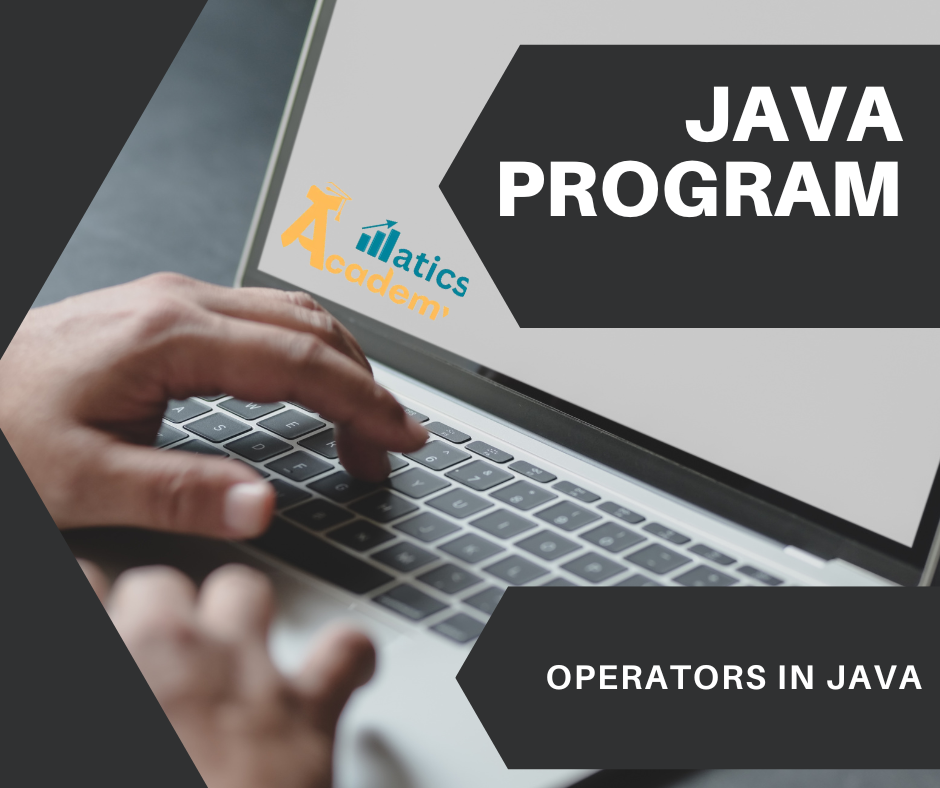
Why Study This Topic?
Operators in Java are fundamental elements that allow programmers to perform operations on variables and values, making them essential for effective coding. Understanding operators in Java not only enhances your programming skills but also prepares you for real-world applications and technical interviews.
What Will Be Covered?
This guide covers:
- Types of Java operators: Arithmetic, Relational, Logical, Assignment, Bitwise, Ternary, and Unary.
- How each operator works with syntax and examples.
- Real-world scenarios and exercises to reinforce learning.
2. Detailed Content of operators in java
Operator Type | Operators | Description | Example | Output |
---|---|---|---|---|
Arithmetic | + , - , * , / , % | Performs basic arithmetic operations. | int a = 10, b = 5; System.out.println(a + b); | 15 |
System.out.println(a % b); | 0 | |||
Relational | == , != , > , < , >= , <= | Compares values, returns true or false . | int x = 10, y = 20; System.out.println(x > y); | false |
System.out.println(x <= y); | true | |||
Logical | && , || , ! | Combines conditions, returns boolean results. | boolean a = true, b = false; System.out.println(a && b); | false |
System.out.println(a || b); | true | |||
System.out.println(!a); | false | |||
Assignment | = , += , -= , *= , /= , %= | Assigns values to variables, supports compound operations. | int num = 10; num += 5; System.out.println(num); | 15 |
Bitwise | & , | , ^ , ~ , << , >> | Operates on binary data at the bit level. | int a = 5, b = 3; System.out.println(a & b); | 1 |
System.out.println(a | b); | 7 | |||
Ternary | ?: | Shorthand for if-else , evaluates a condition in one line. | int score = 85; String result = (score >= 50) ? "Pass" : "Fail"; System.out.println(result); | Pass |
Unary | ++ , -- , + , - , ! , ~ | Performs operations on a single operand. | int a = 5; a++; System.out.println(a); | 6 |
System.out.println(-a); | -6 |
Explanations and Examples
a. Arithmetic Operators
- Used for basic calculations like addition and subtraction.
- Operators:
+
,-
,*
,/
,%
- Example:
- For more on data types used with operators in Java, see our Java Data Types Overview.
int a = 10, b = 5; System.out.println("Sum: " + (a + b)); // Output: 15
b. Relational Operators
- Compare two values and return a boolean result.
- Operators:
==
,!=
,>
,<
,>=
,<=
- Example:
int x = 10, y = 20; System.out.println(x > y); // Output: false
c. Logical Operators
- Combine multiple conditions to form complex logic.
- Operators:
&&
,||
,!
- Example:
- Check out our Conditional Statements in Java guide to see how logical operators work in conditions.
boolean a = true, b = false; System.out.println(a && b); // Output: false
d. Assignment Operators
- Assign values to variables.
- Operators:
=
,+=
,-=
,*=
,/=
,%=
- Example:
int num = 10; num += 5; // Equivalent to num = num + 5
e. Bitwise Operators
- Work with binary representations.
- Operators:
&
,|
,^
,~
,<<
,>>
- Example:
int a = 5, b = 3; System.out.println(a & b); // Output: 1
f. Ternary Operator
- Shortens
if-else
statements. - Syntax:
condition ? value_if_true : value_if_false
- Example:
int score = 85; String result = (score >= 50) ? "Pass" : "Fail";
3. Summary
- Operators in Java include arithmetic, relational, logical, assignment, bitwise, and ternary.
- Understanding these operators improves coding efficiency and simplifies complex logic creation.
- Each operator type has specific uses and advantages for Java development.
4. Learning Outcomes
After completing this guide, learners will be able to:
- Recognize and use each operator type effectively.
- Apply operators in Java to solve programming problems efficiently.
- Use operators in real-world programming tasks with enhanced confidence.
5. Common Interview Questions of operators in java
What is the difference between ==
and .equals()
in Java?
- Explanation:
==
checks for reference equality (whether two references point to the same object in memory), while.equals()
checks for logical equality (whether two objects have the same content).
- Companies: Amazon, Infosys, Cognizant
Explain how the ternary operator works in Java.
- Explanation: The ternary operator is a shorthand for
if-else
statements and has the syntaxcondition ? value_if_true : value_if_false
. It’s used to evaluate a condition in a single line. - Example:
int score = 75; String result = (score > 50) ? "Pass" : "Fail";
- Companies: Wipro, Oracle, Tata Consultancy Services (TCS)
What is the difference between &
and &&
in Java?
- Explanation:
&
is a bitwise AND operator that operates at the binary level, whereas&&
is a logical AND operator that evaluates two boolean expressions. The&&
operator also supports short-circuiting, meaning if the first condition is false, the second is not evaluated. - Companies: IBM, Capgemini, Tech Mahindra
How do increment (++
) and decrement (--
) operators work in Java?
- Explanation: The
++
and--
operators increase or decrease a variable’s value by one. They come in two forms: prefix (++a
) and postfix (a++
). Prefix increments the value before use, while postfix increments after use. - Example:
int a = 5; System.out.println(++a); // Output: 6
andint b = 5; System.out.println(b++); // Output: 5
- Companies: Microsoft, Google, Mindtree
What are bitwise shift operators (<<
, >>
, >>>
) in Java, and how do they work?
- Explanation: Bitwise shift operators manipulate bits of an integer:
<<
(left shift) shifts bits to the left and fills in zeros on the right.>>
(right shift) shifts bits to the right, preserving the sign bit.>>>
(unsigned right shift) shifts bits to the right and fills in zeros, ignoring the sign bit.
- Example:
int a = 8; System.out.println(a << 1); // Output: 16
- Companies: Accenture, JPMorgan Chase, HCL Technologies
6. Practice Exercises
1. Arithmetic Operator Practice
Question: Write a Java program to calculate the result of (a + b) * c
, where a = 5
, b = 3
, and c = 2
.
Answer:
public class ArithmeticExample { public static void main(String[] args) { int a = 5, b = 3, c = 2; int result = (a + b) * c; System.out.println("Result: " + result); // Output: 16 } }
Explanation: The program calculates (5 + 3) * 2
resulting in 16
.
2. Relational Operator Practice
Question: Write a Java program to check if x
is greater than y
, where x = 20
and y = 10
.
Answer:
public class RelationalExample { public static void main(String[] args) { int x = 20, y = 10; System.out.println(x > y); // Output: true } }
Explanation: Since 20
is greater than 10
, the output is true
.
3. Logical Operator Practice
Question: Given boolean a = true
and boolean b = false
, write a program to display the result of a || b
and a && b
.
Answer:
public class LogicalExample { public static void main(String[] args) { boolean a = true, b = false; System.out.println(a || b); // Output: true System.out.println(a && b); // Output: false } }
Explanation: a || b
is true
because at least one operand is true
. a && b
is false
because both operands are not true
.
4. Ternary Operator Practice
Question: Write a program to assign “Even” or “Odd” to a String
variable result
based on the value of num = 4
.
Answer:
public class TernaryExample { public static void main(String[] args) { int num = 4; String result = (num % 2 == 0) ? "Even" : "Odd"; System.out.println(result); // Output: Even } }
Explanation: num % 2 == 0
evaluates to true
for even numbers, so result
is assigned “Even.”
5. Bitwise Operator Practice
Question: Write a program to perform a bitwise AND on a = 6
and b = 4
, then print the result.
Answer:
public class BitwiseExample { public static void main(String[] args) { int a = 6; // Binary: 110 int b = 4; // Binary: 100 System.out.println(a & b); // Output: 4 } }
Explanation: Bitwise AND compares each bit and results in 100
(binary), which is 4
in decimal.
6. Unary Operator Practice
Question: Write a Java program to demonstrate the difference between pre-increment and post-increment using int x = 5
.
Answer:
public class UnaryExample { public static void main(String[] args) { int x = 5; System.out.println("Post-increment: " + (x++)); // Output: 5 System.out.println("After post-increment, x: " + x); // Output: 6 x = 5; // Reset x System.out.println("Pre-increment: " + (++x)); // Output: 6 } }
Explanation: In post-increment (x++
), x
is printed before incrementing. In pre-increment (++x
), x
is incremented first and then printed.
7. Assignment Operator Practice
Question: Write a program to use the +=
operator to add 10
to x
where x = 15
.
Answer:
public class AssignmentExample { public static void main(String[] args) { int x = 15; x += 10; // Equivalent to x = x + 10 System.out.println(x); // Output: 25 } }
Explanation: x += 10
adds 10
to the current value of x
, resulting in 25
.
7. Additional Resources
- Books: Effective Java by Joshua Bloch.
- Online Tutorials: Explore Oracle’s official Java Documentation.
- Tools: Practice in JDoodle or Repl.
PLAY WITH JAVA…!!
Question
Your answer:
Correct answer:
Your Answers