Arrays are a powerful data structure in Python, enabling you to store multiple items of the same type in a single variable. Accessing items in an array is fundamental for manipulating and analyzing data. This guide will delve into the essentials of accessing array items in Python, along with practical examples and tips to optimize your code. Python Access Array indexing is a key concept to understand.
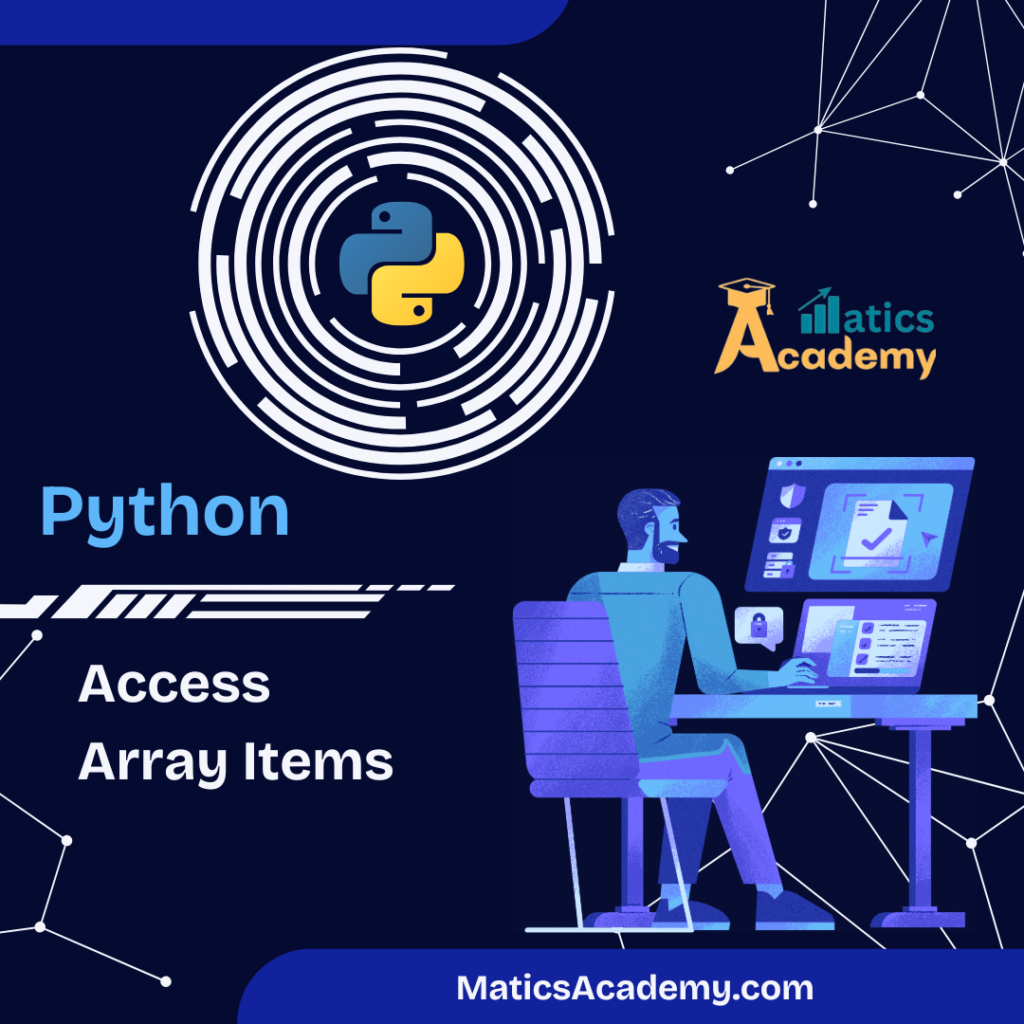
What Are Array Items in Python?
Array items refer to the individual elements stored within an array. Each item is assigned a unique index, starting from 0
for the first item. By accessing these items, you can retrieve, modify, or process data stored in the array using Python array indexing.
How to Access Array Items in Python?
1. Accessing Array Elements by Index
To access a specific element, use the index number in square brackets []
.
Example:
import array my_array = array.array('i', [10, 20, 30, 40]) print(my_array[1]) # Output: 20
Explanation: The index 1
retrieves the second element (20
). Mastering the concept of array indexing is crucial when learning Python and trying to access an array effectively.
2. Accessing Items Using Negative Indexing
Python arrays allow negative indexing to access elements from the end of the array.
Example:
print(my_array[-1]) # Output: 40
Explanation: The index -1
fetches the last element of the array. Using Python array indexing in this way is very useful.
3. Accessing Multiple Items Using Slicing
Slicing retrieves a portion of the array by specifying a start and end index.
Example:
print(my_array[1:3]) # Output: array('i', [20, 30])
Explanation: This method extracts elements from index 1
to 2
(end index 3
is excluded). Slicing is another form of array indexing in Python.
Best Practices for Accessing Array Items
- Validate Indexes: Always ensure the index is within the valid range to avoid
IndexError.
Proper use of Python array indexing will help. - Use Slicing for Subsets: Utilize slicing to work efficiently with parts of the array. Understand Python’s array indexing to enhance slicing techniques for accessing arrays.
- Consider NumPy Arrays: For advanced operations, NumPy arrays provide additional capabilities.
Common Errors and How to Avoid Them
- IndexError:
Occurs when trying to access an index that doesn’t exist.
Fix: Check the length of the array usinglen()
before accessing elements to prevent errors in Python array indexing. - TypeError:
Happens when you use invalid index types like floats.
Fix: Ensure indexes are integers, especially when performing array indexing in Python.
Real-World Applications of Accessing Array Items
- Data Analysis: Extracting specific data points for analysis often depends on Python array indexing.
- Machine Learning: Preprocessing datasets by slicing arrays uses Python array indexing principles.
- Web Development: Managing arrays for dynamic front-end interactions is simpler with a clear understanding of Python array indexing techniques.
Key Benefits of Mastering Array Access in Python
- Enhanced Efficiency: Retrieve data quickly and efficiently by leveraging Python array indexing.
- Improved Debugging: Identify and isolate issues in data more easily when adept at Python array indexing.
- Versatile Applications: Apply the skill across various domains like AI, data science, and web development by using Python array indexing and Dictionaries.
Interview Questions
1.What is the difference between positive and negative indexing in Python arrays?(Google)
Positive indexing starts from 0
for the first element and increments by 1
. Negative indexing, on the other hand, starts from -1
for the last element and decrements as you move backward. Negative indexing is particularly useful when accessing elements from the end of the array without knowing its length
2.How can slicing be used to access multiple elements in a Python array? (Amazon)
Slicing allows you to access a subset of elements by specifying a start index, end index, and an optional step. For example, my_array[1:4]
retrieves elements from index 1
to 3
(excluding 4
). Slicing is an efficient way to work with portions of the array
3.What are some common errors encountered while accessing array items in Python? (Microsoft)
The most common errors include:
- IndexError: Occurs when trying to access an index outside the range of the array.
- TypeError: Happens if the index provided is not an integer (e.g., a float or string).
To avoid these, validate the index and ensure it matches the data type requirement.
4.Can you explain the role of the len()
function when accessing array items? (Meta)
The len()
function returns the total number of elements in an array. It’s often used to determine valid index ranges, ensuring that no IndexError
occurs. For example, before accessing my_array[5]
, checking len(my_array)
ensures that 5
is within bounds.
5.How would you access the last element in an array dynamically without knowing its length? (IBM)
To access the last element, use negative indexing with -1
. For example, my_array[-1]
retrieves the last item regardless of the array’s length. This is a convenient method to dynamically handle arrays of varying sizes
Lets play : Access Array Items
Question
Your answer:
Correct answer:
Your Answers