Practicing array exercises is essential for mastering arrays in Python. Whether you’re a beginner or someone looking to strengthen your array handling skills, solving array exercises will help you become proficient in using arrays to manage data effectively. In addition, this guide presents a series of Python array exercises with solutions, so you can learn through practice.
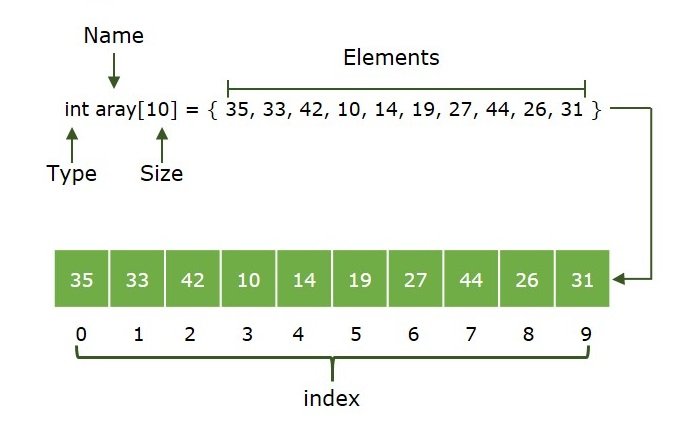
Why Practice Python Array Exercises?
Practicing array exercises allows you to familiarize yourself with how arrays work in Python. Arrays are widely used in data processing, machine learning, and numerical computations, so building your proficiency is crucial. By solving different array exercises and you can learn how to manipulate arrays, implement efficient algorithms, and handle data more effectively.
Array Exercises for Beginners
1. Create an Array
Create a Python array of integers and add multiple elements using the append()
and extend()
methods and Learn how to add elements to an array dynamically.
Example:
from array import array arr = array('i', [1, 2, 3]) arr.append(4) # Adds 4 at the end arr.extend([5, 6]) # Adds multiple elements print(arr) # Output: array('i', [1, 2, 3, 4, 5, 6])
2. Insert Elements
Insert a number at a specific index within an existing array using the insert()
method and Understand how to place an element at a specific index.
Example:
arr.insert(2, 10) # Inserts 10 at index 2 print(arr) # Output: array('i', [1, 2, 10, 3, 4, 5, 6])
3. Remove an Element by Value
Use the remove()
method to remove the first occurrence of a specific value from the array and Learn how to delete elements by value.
Example:
arr.remove(4) # Removes the first occurrence of 4 print(arr) # Output: array('i', [1, 2, 10, 3, 5, 6])
4. Pop Elements in Array
Remove and return the last element or an element from a specific index using the pop()
method and Explore how to manage array elements dynamically.
Example:
popped_element = arr.pop(3) # Removes and returns the element at index 3 print(arr) # Output: array('i', [1, 2, 10, 5, 6]) print(popped_element) # Output: 3
5. Reverse the Order
Reverse the elements of an array in place using the reverse()
method and Understand how to reverse arrays for data manipulation.
Example:
arr.reverse() # Reverses the array print(arr) # Output: array('i', [6, 5, 10, 2, 1])
Advanced Python Array Exercises
6. Find the Index
Use the index()
method to find the position of an element in the array and Learn how to search for elements in arrays.
Example:
index_of_10 = arr.index(10) # Finds the index of 10 print(index_of_10) # Output: 2
7. Count Occurrences
Use the count()
method to determine how many times a specific element appears in the array and Learn how to count occurrences of elements in an array.
Example:
arr = array('i', [1, 2, 3, 2, 5, 2]) count_of_2 = arr.count(2) print(count_of_2) # Output: 3
8. Convert Array to List
Convert a Python array to a regular Python list using the tolist()
method and Understand how to convert arrays to lists for additional operations.
Example:
arr_as_list = arr.tolist() print(arr_as_list) # Output: [6, 5, 10, 2, 1]
9. Array Memory
Use the buffer_info()
method to get memory details about the array and Gain insight into the internal workings of arrays and their memory usage.
Example:
memory_info = arr.buffer_info() print(memory_info) # Output: (memory_address, number_of_elements)
Best Practices for Array Exercises
- Start small: Begin with simple exercises and gradually increase the complexity as you become more comfortable with arrays.
- Understand the problem: Read each exercise carefully and ensure you understand the task before jumping into coding.
- Practice regularly: The more exercises you solve, the better you’ll get at using arrays in Python and
- Use array methods wisely: Python provides various methods to manipulate arrays efficiently, so make sure you’re using the most suitable method for the task.
Applications of Array Exercises in Python
- Data Processing Arrays are highly efficient for handling and processing large datasets, especially in numerical or scientific computing. By practicing array exercises, you’ll learn how to manage data such as sensor readings, financial records, and statistical data.
- Mathematical and Statistical Calculations Arrays are ideal for performing mathematical operations like matrix multiplication, solving systems of linear equations, and other numerical computations. By solving array exercises, you gain familiarity with implementing algorithms for statistical analysis, machine learning, and AI applications.
- Image and Video Processing Arrays are extensively used in image and video processing, where each pixel of an image can be stored as an element in a 2D or 3D array. Practicing array exercises allows you to efficiently manipulate data for tasks like image filtering, transformations, and object detection.
- Gaming and Graphics Arrays are used in games for storing and manipulating game states, grids, and object positions. Understanding how arrays work will help you in creating and managing game worlds, coordinates, and animations.
- Memory Management and Optimization Arrays are more memory-efficient than lists, which makes them useful for applications that need to optimize memory usage, such as embedded systems, IoT devices, and performance-critical applications.
- Sorting and Searching Algorithms Arrays are commonly used in sorting and searching algorithms, such as binary search or quicksort. By practicing array exercises, you’ll learn how to implement these algorithms efficiently.
Conclusion
Practicing array exercises is one of the best ways to learn how to manipulate and manage data in Python. By solving problems related to array creation, manipulation, searching, and deletion, you will solidify your understanding of arrays and gain practical experience. and it Start practicing these exercises today to improve your Python programming skills and enhance your ability to handle arrays efficiently.
Interview Questions
1.What is an array in Python, and how does it differ from a list? (Google)
In Python, an array is a collection of elements of the same data type. Arrays are provided by the array
module, and they store data in contiguous memory locations. This homogeneity ensures that arrays are optimized for numerical operations and memory-efficient.
Key Differences Between Arrays and Lists in Python
- Data Type Arrays can only store elements of the same type (like all numbers), which helps keep things simple and organized. However, lists can store different types of elements (like numbers, strings, etc.), giving them more flexibility.
- Memory Efficiency Arrays use less memory because they store only one type of data. This makes them better for large sets of numbers. In comparison, The lists use more memory because they can store different types of data and which can make them less efficient when working with a lot of data.
- Operations Arrays are faster when performing math operations (like adding or multiplying numbers) because they are designed for numerical tasks. but On the other hand and lists are more versatile and can store different types of data, but they are slower for mathematical operations on large datasets.
2.Explain the purpose of the array()
constructor in Python? (TCS)
The array()
constructor in Python is used to create an array and The constructor takes two main arguments:
- The type code (a character indicating the data type of the elements in the array).
- A sequence of initial values (such as a list or tuple) and to populate the array.
3.What are the common methods available for manipulating arrays in Python? (IBM)
The array
module in Python offers several built-in methods for manipulating arrays. Some of the most commonly used methods include:
- append(): Adds a single element at the end of the array.
- extend(): Adds multiple elements from another iterable ( like a list or another array).
- insert(): Inserts an element at a specific index in the array.
- pop(): Removes and returns the element at the specified index.
- remove(): Removes the first occurrence of a specified value.
- reverse(): Reverses the order of elements in the array.
- count(): Returns the number of occurrences of a specified value in the array.
- index(): Returns the index of the first occurrence of a specified value.
- tolist(): Converts the array into a list and
- buffer_info(): Returns memory address information and the number of elements in the array.
4.What is the use of the index()
method in Python arrays? (Google)
The index()
method in Python arrays is used to find the index of the first occurrence of a specified value in the array and If the value is not found, it raises a ValueError
.
5. How do you reverse an array in Python? (Infosys)
To reverse the elements of an array in Python, you can use the reverse()
method and This method reverses the elements of the array in place (i.e., modifies the original array).
Learn about arrays and Dictionary
Lets play : array Eercises
Question
Your answer:
Correct answer:
Your Answers