Introduction to Data Compression in Python
Data compression is a crucial technique used to reduce the size of data while maintaining its integrity. Python offers several built-in libraries to perform data compression, making it easier to store and transfer large amounts of information efficiently.
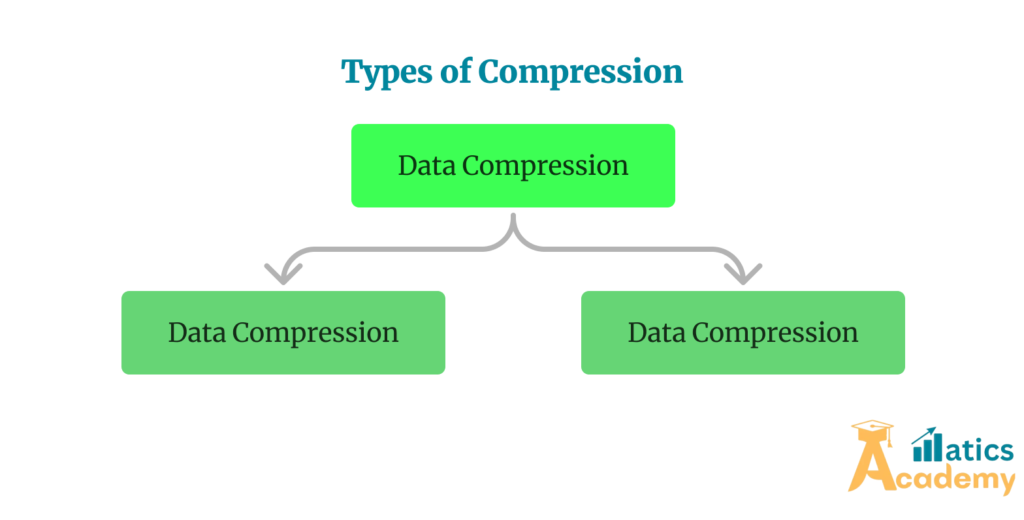
In this article, we’ll explore data compression techniques in Python, common libraries, algorithms, and best practices for optimizing storage and speed.
Why is Data Compression Important?
- Data compression is essential for:
- Reducing Storage Space: Saves disk space by minimizing file sizes.
- Faster Data Transmission: Compressed files transfer quicker over networks.
- Lower Bandwidth Consumption: Reduces the amount of data being sent.
- Improving Performance: Optimizes memory usage for applications.
- Efficient Backup and Archiving: Saves space while storing historical data.
Types of Data Compression
Python supports two major types of data compression:
1. Lossless Compression
- Ensures that no data is lost during compression and decompression.
- Commonly used for text, software, and database files.
- Examples: ZIP, GZIP, BZIP2, LZMA
2. Lossy Compression
- Reduces file size by eliminating less critical data.
- Commonly used for images, audio, and video files.
- Examples: JPEG, MP3, MPEG, WebP
Python Libraries for Data Compression
Python provides several built-in libraries for data compression:
1. zlib (GZIP Compression)
- Uses Deflate compression algorithm.
- Commonly used for compressing HTTP responses.
Example: Compress and Decompress Data using zlib
import zlib data = b"Hello, this is a test string for compression." compressed_data = zlib.compress(data) decompressed_data = zlib.decompress(compressed_data) print("Compressed:", compressed_data) print("Decompressed:", decompressed_data)
2. gzip (GNU Zip Compression)
- Works with
.gz
files. - Efficient for large text files.
Example: Writing and Reading Gzip Files
import gzip data = b"This is an example of gzip compression in Python." # Compressing data with gzip.open("example.gz", "wb") as f: f.write(data) # Decompressing data with gzip.open("example.gz", "rb") as f: decompressed_data = f.read() print("Decompressed:", decompressed_data)
3. bz2 (Bzip2 Compression)
- Uses Burrows-Wheeler Algorithm for better compression.
- Suitable for text and log files.
Example: Bzip2 Compression in Python
import bz2 data = b"Python Bzip2 Compression Example." # Compress data compressed_data = bz2.compress(data) print("Compressed:", compressed_data) # Decompress data decompressed_data = bz2.decompress(compressed_data) print("Decompressed:", decompressed_data)
4. lzma (Lempel-Ziv-Markov Chain Algorithm)
- Offers higher compression ratios than gzip and bzip2.
- Used in 7zip (.xz) format.
Example: LZMA Compression
import lzma data = b"Python LZMA Compression Example." # Compress data compressed_data = lzma.compress(data) print("Compressed:", compressed_data) # Decompress data decompressed_data = lzma.decompress(compressed_data) print("Decompressed:", decompressed_data)
Comparing Compression Algorithms in Python
Algorithm | Compression Speed | Decompression Speed | Compression Ratio | Best For |
---|---|---|---|---|
zlib (gzip) | Fast | Fast | Moderate | Web responses, log files |
gzip | Fast | Fast | Moderate | Large text files |
bz2 | Moderate | Moderate | High | Log files, backups |
lzma | Slow | Slow | Very High | Archiving, software distribution |
Best Practices for Data Compression
- Choose the Right Algorithm: Select the best compression method based on file type.
- Optimize Performance: Compress only large files to avoid unnecessary overhead.
- Balance Speed and Compression Ratio: Use gzip for speed, bz2 for better compression, and lzma for high compression efficiency.
- Use Streaming Compression: For large files, compress data in chunks rather than loading it all at once.
Additional Topics:
Interview Questions:
1. What is the difference between lossless and lossy compression?(Google)
Answer:
- Lossy compression removes less important data to achieve better compression ratios. Example: JPEG, MP3.
- Lossless compression retains all original data, allowing for exact reconstruction. Example: ZIP, PNG.
2. How do you compress a text file using Python?(Microsoft)
Answer:
You can use the gzip
or bz2
module:
import gzip with gzip.open("file.txt.gz", "wt") as f: f.write("This is a test file for compression.")
3. Which compression algorithm provides the best compression ratio?(Amazon)
Answer:LZMA
provides the best compression ratio, but it is slower compared to gzip
or bz2
.
Play by compress!
Question
Your answer:
Correct answer:
Your Answers