Introduction to Python Dictionaries
Dictionaries in Python are unordered, mutable, and indexed collections. They store data in key-value pairs and are highly efficient for lookup operations.
Syntax:
my_dict = {"key1": "value1", "key2": "value2"}
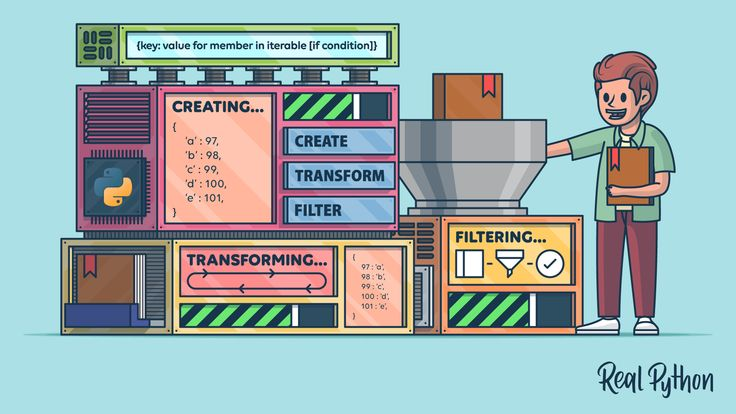
Key Features of Python Dictionaries
- Keys: Must be unique and immutable (e.g., strings, numbers, tuples).
- Values: Can be of any data type and duplicated.
- Dynamic: Dictionaries grow or shrink as needed.
- Fast Lookup: Keys are hashed, enabling rapid access.
Creating a Python Dictionary
You can create a dictionary using curly braces {}
or the dict()
constructor:
# Using curly braces my_dict = {"name": "Alice", "age": 25} # Using dict() my_dict = dict(name="Alice", age=25)
Accessing Values
Access values using the key:
print(my_dict["name"]) # Output: Alice print(my_dict.get("age")) # Output: 25
Use .get()
to avoid errors if the key doesn’t exist.
Modifying Dictionaries
- Add a key-value pair:
pythonCopyEditprint(my_dict["name"]) # Output: Alice print(my_dict.get("age")) # Output: 25
- Update a value:
my_dict["age"] = 26
Removing Items
pop(key)
: Removes the specified key-value pair.popitem()
: Removes the last inserted key-value pair.del
: Deletes a key or the entire dictionary.
my_dict.pop("age") del my_dict["location"]
Dictionary Methods
Method | Description | Example |
---|---|---|
clear() | Removes all items. | my_dict.clear() |
copy() | Returns a shallow copy. | copy_dict = my_dict.copy() |
keys() | Returns all keys as a view object. | print(my_dict.keys()) |
values() | Returns all values as a view object. | print(my_dict.values()) |
items() | Returns key-value pairs as a view. | print(my_dict.items()) |
update() | Updates the dictionary with another. | my_dict.update({"age": 30}) |
Looping Through Dictionaries
Iterate over keys, values, or items:
# Loop through keys for key in my_dict: print(key) # Loop through values for value in my_dict.values(): print(value) # Loop through items for key, value in my_dict.items(): print(key, value)
Nesting Dictionaries
Dictionaries can contain other dictionaries:
nested_dict = { "person1": {"name": "Alice", "age": 25}, "person2": {"name": "Bob", "age": 30} }nested_dict = { "person1": {"name": "Alice", "age": 25}, "person2": {"name": "Bob", "age": 30} }
Common Python Interview Questions (CIQ) on Dictionaries
How are dictionaries different from lists?
- Lists are ordered collections indexed by position, while dictionaries are unordered and indexed by keys
What happens if you use a mutable object as a dictionary key?
- Python raises a
TypeError
because dictionary keys must be immutable.
Explain the difference between pop()
and popitem()
methods in dictionaries.
pop(key)
removes the specified key-value pair, whilepopitem()
removes the last inserted pair.
How can you merge two dictionaries in Python 3.9+?
- Use the
|
operator:pythonCopyEditmerged_dict = dict1 | dict2
Can a dictionary have duplicate keys?
- No, keys must be unique. If a key is duplicated, the last value assigned to it is retained.
Additional Resources
- Python Official Documentation on Dictionaries
- Python Dictionary Methods Guide
- Practice Problems on Dictionaries
Nested Dictionaries
Question
Your answer:
Correct answer:
You got {{SCORE_CORRECT}} out of {{SCORE_TOTAL}}
Your Answers