Pandas is a powerful and versatile library in Python designed for data manipulation and analysis. It provides fast, flexible, and expressive data structures, making it an essential tool for data scientists and analysts. This tutorial will cover the basics of Pandas, including its core functionalities, with practical examples to get you started. If you are looking for a comprehensive Pandas Python tutorial, you have come to the right place.

What is Pandas in Python?
Pandas is an open-source library that builds on top of NumPy. It provides data structures like Series and DataFrames, which help handle structured data efficiently. It excels in data cleaning, transformation, and exploration, enabling seamless handling of large datasets.
Why Use Pandas?
Pandas provides tools to manipulate data in various ways, such as merging, filtering, reshaping, and aggregating. It supports data from diverse sources like CSV, Excel, SQL databases, and more. With Pandas, you can quickly analyze and process large datasets efficiently.
Steps to Install Pandas
Step 1: Install Pandas
Ensure Pandas is installed on your system. You can install it using pip:
pip install pandas
Step 2: Import Pandas
Start your script by importing the Pandas library.
import pandas as pd
Step 3: Create a Data Structure
Creating a Series:
data = pd.Series([10, 20, 30, 40, 50]) print(data)
Creating a DataFrame:
data = {'Name': ['Alice', 'Bob'], 'Age': [25, 30]} df = pd.DataFrame(data) print(df)
Step 4: Load Data
You can load data from a CSV, Excel file, or database.
df = pd.read_csv('file.csv') # Load CSV file df = pd.read_excel('file.xlsx') # Load Excel file
Step 5: Explore Data
Understand the structure of your dataset.
print(df.head()) # Display first 5 rows print(df.info()) # Summary of dataset print(df.describe()) # Statistical details
Step 6: Clean Data
Handle missing or incorrect data.
df.fillna(0, inplace=True) # Replace NaN with 0 df.dropna(inplace=True) # Remove rows with NaN
Step 7: Filter and Sort Data
Filter rows and sort them by column values.
filtered = df[df['Age'] > 25] # Filter rows where Age > 25 sorted_df = df.sort_values(by='Name') # Sort by Name
Step 8: Analyze Data
Group data and perform aggregations.
grouped = df.groupby('Category')['Value'].sum() # Group and sum
Step 9: Save Processed Data
Save your changes back to a file.
df.to_csv('processed_file.csv', index=False) # Save to CSV
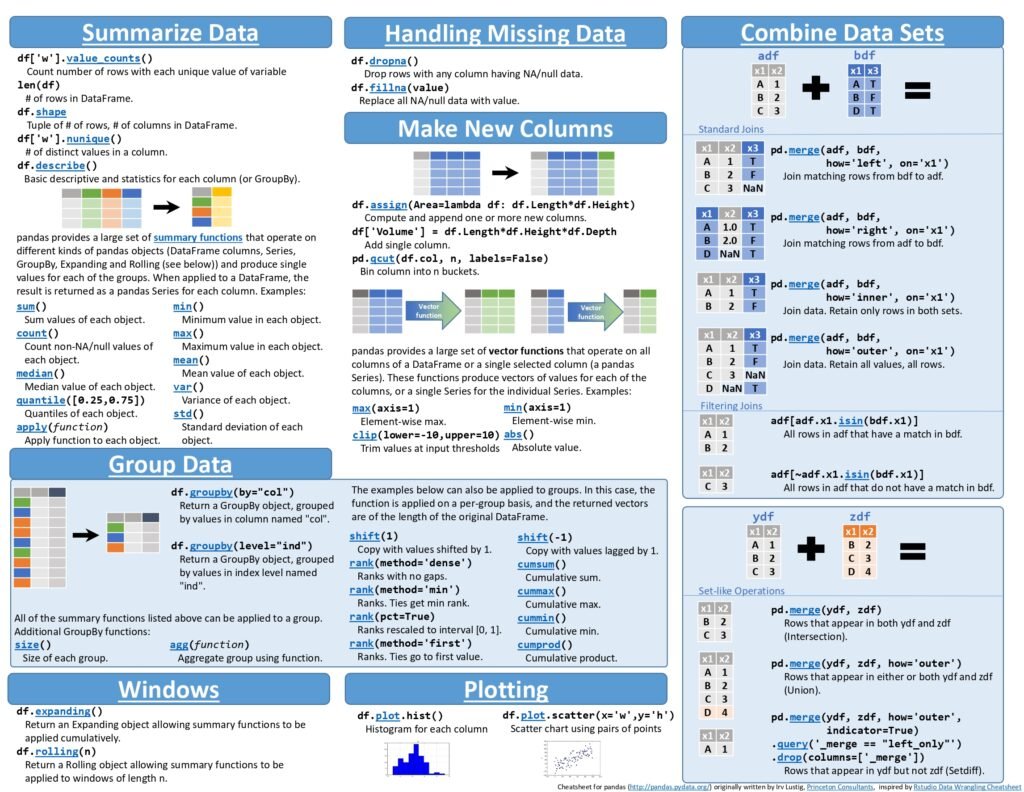
Core Data Structures in Pandas
1. Series
A Pandas Series is a one-dimensional array-like structure capable of holding data of any type.
Example:
import pandas as pd data = pd.Series([1, 2, 3, 4, 5]) print(data)
2. DataFrame
The DataFrame is a two-dimensional labeled data structure, much like a table in a relational database.
Example:
data = {'Name': ['Alice', 'Bob'], 'Age': [25, 30]} df = pd.DataFrame(data) print(df)
Key Features of Pandas
1. Data Import and Export
Pandas supports importing and exporting data from multiple formats, such as CSV, Excel, JSON, and SQL.
df = pd.read_csv('data.csv') df.to_excel('output.xlsx', index=False)
2. Data Cleaning
Pandas makes it easy to handle missing data and perform operations like filling, dropping, or interpolating missing values.
df.fillna(0, inplace=True) df.dropna(inplace=True)
3. Data Manipulation
You can filter rows, update values, and manipulate data with Pandas.
filtered_df = df[df['Age'] > 25]
4. Grouping and Aggregation
Pandas simplifies data aggregation with its groupby
functionality.
grouped = df.groupby('Category').sum()
5. Merging and Joining
Combine multiple datasets with merge and join operations.
merged_df = pd.merge(df1, df2, on='key')
Practical Examples
Loading a Dataset
import pandas as pd df = pd.read_csv('https://example.com/dataset.csv') print(df.head())
Handling Missing Data
df['column_name'].fillna(df['column_name'].mean(), inplace=True)
Sorting Data
sorted_df = df.sort_values(by='Age', ascending=True)
Advantages of Pandas
- Ease of Use: Pandas offers a simple and intuitive API for data manipulation.
- Performance: Built on NumPy, Pandas ensures high performance for large datasets.
- Compatibility: Supports integration with other libraries like Matplotlib and Scikit-learn for seamless data workflows.
Best Practices for Using Pandas
- Use Vectorized Operations: Avoid loops; instead, use built-in functions for better performance.
- Handle Missing Data Early: Clean your data before processing to avoid errors.
- Work with Copies: Use
.copy()
when modifying data to avoid unintended changes.
Conclusion
Pandas is a must-have library for anyone working with data in Python. Its robust features make data analysis faster and more efficient, whether you’re cleaning, transforming, or visualizing data. Start exploring Pandas today to unlock its full potential in your data projects.
Interview Questions
1.What is Pandas in Python, and why is it used? (Google)
Pandas is an open-source Python library widely used for data manipulation and analysis. It provides powerful data structures, such as Series and DataFrame, to efficiently handle and analyze structured data. Pandas simplifies tasks like cleaning, transforming, and aggregating data, making it essential for data science and machine learning workflows.
2.How is a Pandas DataFrame different from a NumPy array? (Amazon)
While NumPy arrays provide a foundation for numerical computations with homogeneous data types, Pandas DataFrames offer labeled axes (rows and columns) and can handle heterogeneous data types. DataFrames also provide functionalities for handling missing data, indexing, and group operations, making them more versatile for data analysis tasks.
3.Explain the difference between loc[]
and iloc[]
in Pandas? (Microsoft)
The loc[]
method is used for label-based indexing, allowing access to rows and columns using their labels. In contrast, iloc[]
is used for positional indexing, where rows and columns are accessed using integer indices. For example, df.loc[1, 'Name']
fetches data using labels, whereas df.iloc[1, 0]
fetches data using positional indices.
4.What are the common file formats supported by Pandas for data import and export? (Netflix)
Pandas supports various file formats, including CSV (read_csv()
and to_csv()
), Excel (read_excel()
and to_excel()
), JSON (read_json()
and to_json()
), and SQL databases (read_sql()
and to_sql()
). These functions make it easy to integrate Pandas with different data sources for streamlined workflows.
5. How do you handle missing data in Pandas? (Meta)
Missing data in Pandas can be managed using methods such as fillna()
to fill missing values with a specified value, dropna()
to remove rows or columns containing missing data, and isnull()
or notnull()
to identify missing data. These methods ensure data integrity and enable cleaner analysis.
Learn about Git Tutorial And Pandas
Lets play : SciPy Tutorial
Question
Your answer:
Correct answer:
Your Answers