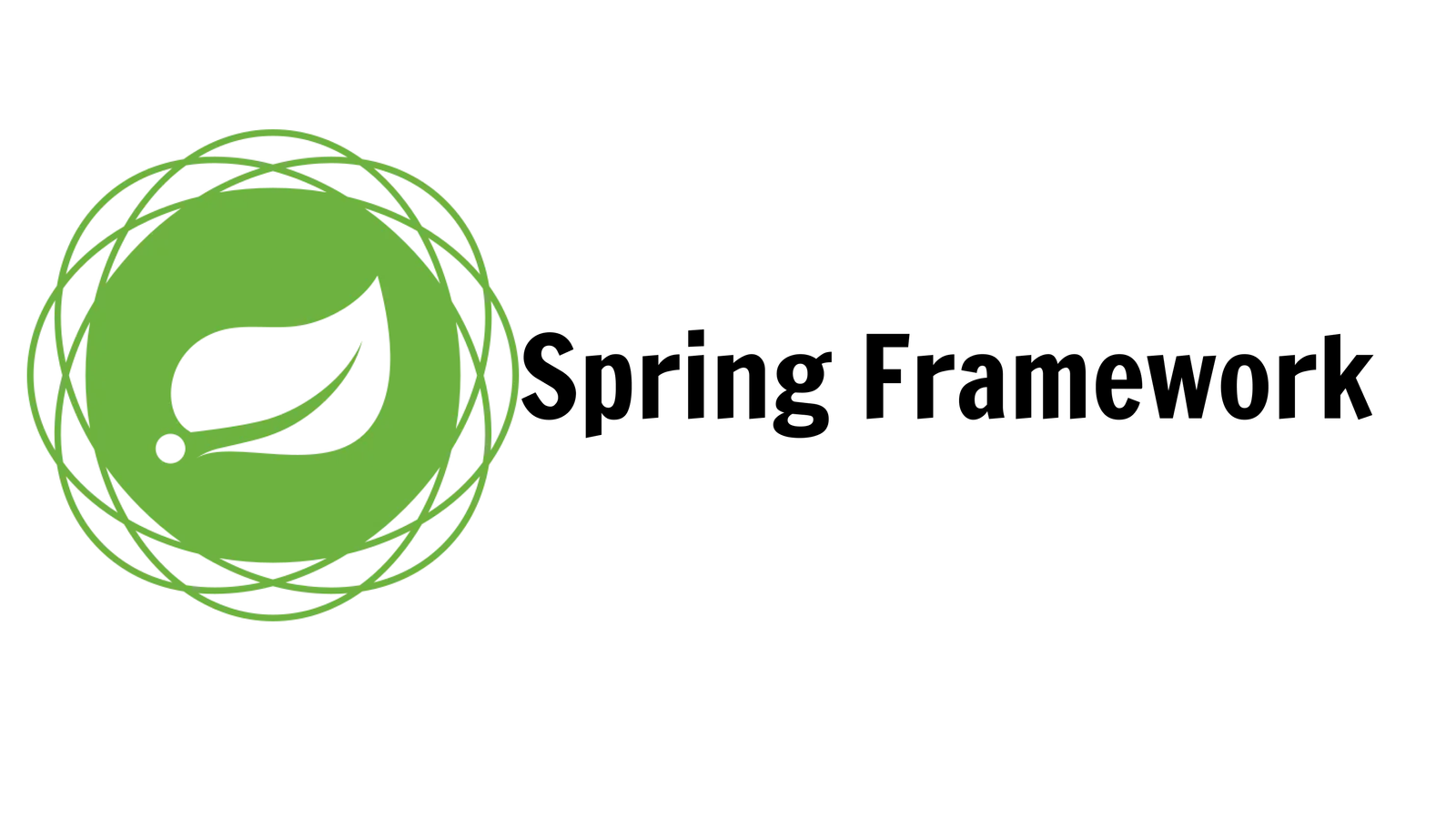
Introduction to the Spring Framework
Spring Framework in Java is a powerful, open-source framework widely used for building enterprise-level applications. Known for its lightweight and modular architecture, Spring simplifies Java development and includes features like dependency injection, aspect-oriented programming, and comprehensive transaction management. In this guide, we explore Spring Framework’s core components and key features, showing how it can streamline Java application development.
Core Concepts of Spring Framework in Java
The Spring Framework is based on core principles designed to simplify Java development:
- Dependency Injection (DI): Spring promotes modular and maintainable code by injecting dependencies at runtime, ensuring that objects are not hard-coded within the application.
- Aspect-Oriented Programming (AOP): With AOP, Spring allows you to separate cross-cutting concerns, such as logging and security, from core business logic. This makes code cleaner and easier to manage.
- Inversion of Control (IoC): IoC is a principle where control is shifted from the application to the framework, letting Spring handle object creation and management.
Key Features of Spring Framework
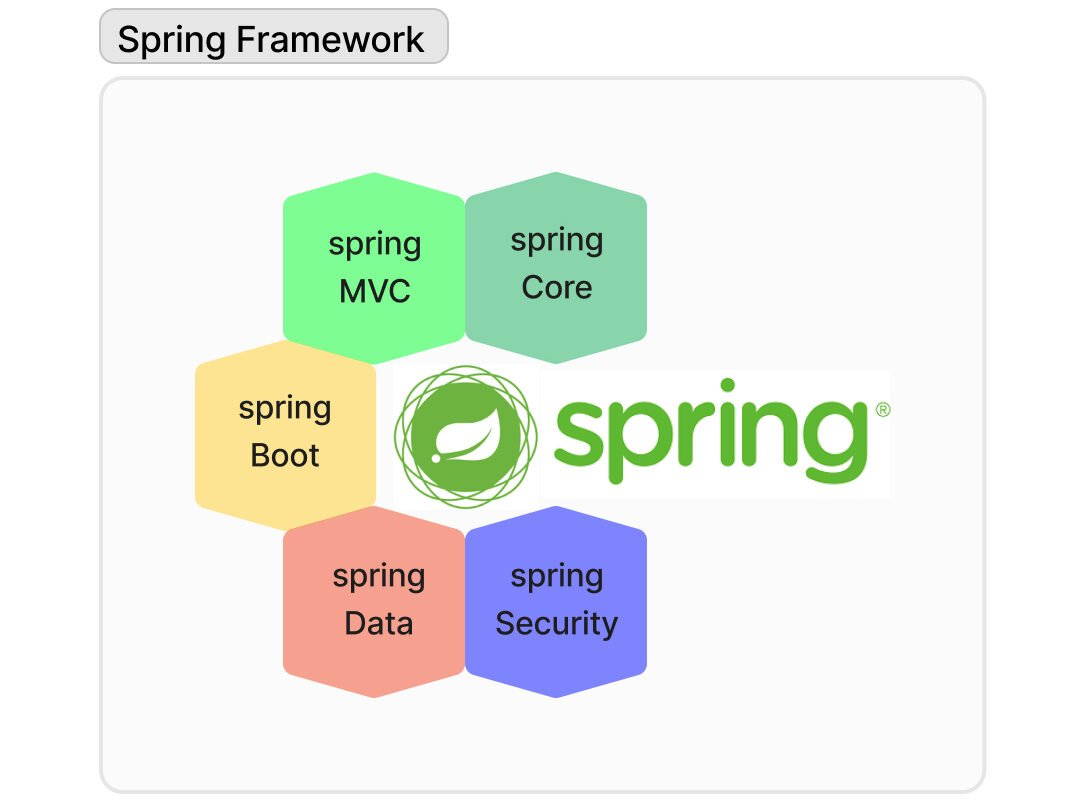
The Spring Framework in Java provides several core modules to streamline application development:
Spring Core
The core module provides fundamental Inversion of Control (IoC) and Dependency Injection (DI) capabilities, which form the base for other modules in Spring.
Spring MVC
As a Model-View-Controller framework, Spring MVC organizes web applications by separating presentation, logic, and data, ensuring a clean code structure.
Spring Boot
With features like auto-configuration and embedded servers, Spring Boot streamlines application setup, making it easy to create standalone applications rapidly.
Spring Data
By abstracting CRUD operations and complex queries, Spring Data simplifies database interactions, supporting databases such as MySQL and MongoDB.
Spring Security
Spring Security provides authentication, authorization, and protection against common threats, simplifying robust security implementation.
Setting Up Spring Framework in Java
To start with Spring, you need the following setup:
- Java Development Kit (JDK)
- Spring Tool Suite (STS)
- Maven or Gradle
Include Spring dependencies in your pom.xml (for Maven) or build.gradle (for Gradle) files to enable Spring functionality.
For example, to include Spring Core:
Maven:
<dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>5.3.10</version> </dependency>
For Gradle:
implementation 'org.springframework:spring-context:5.3.10'
Basic Example: Using Dependency Injection
To demonstrate Spring’s dependency injection:
public interface MessageService { void sendMessage(String message); } public class EmailService implements MessageService { @Override public void sendMessage(String message) { System.out.println("Email Message: " + message); } } @Configuration public class AppConfig { @Bean public MessageService messageService() { return new EmailService(); } } public class Application { public static void main(String[] args) { ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class); MessageService service = context.getBean(MessageService.class); service.sendMessage("Hello, Spring!"); } }
Advantages of Spring Framework in Java
- Modularity and Flexibility: Choose only the components you need, such as Spring Data or Spring Security.
- Ease of Testing: Spring makes testing easier because of its dependency injection capabilities and with tools like JUnit available for comprehensive testing.
- Community Support: Moreover, a large community contributes plugins, tools, and resources, which makes troubleshooting easier and ensures quick access to support.
- Enterprise Tool Integration: In addition, Spring integrates well with tools like Hibernate, JDBC, and JMS, making it an ideal choice for enterprise environments.
Conclusion
Spring Framework in Java revolutionizes development with a modular, efficient approach to building scalable applications. With tools like dependency injection, AOP, and Spring Boot, Spring simplifies and enhances Java development, empowering you to create robust, enterprise-ready applications.
Interview Questions
1. What is the core concept of Inversion of Control (IoC) in the Spring Framework?(Accenture)
IoC allows Spring to manage object creation and dependencies, removing the need for manual instantiation and coupling, improving flexibility.
2. Explain the difference between @Component
, @Service
, and @Repository
in Spring.(Accenture)
All three annotations are used for Spring beans, but @Service
is for service layer classes, @Repository
is for persistence layer classes, and @Component
is a generic annotation for other Spring-managed beans.
3. What is Dependency Injection (DI) and how is it implemented in Spring?(Cognizant)
DI is a design pattern in which dependencies are injected rather than created within objects. Spring implements DI using constructor, setter, or field injection.
4. How does Spring Boot simplify application development compared to Spring?(Cognizant)
Spring Boot offers auto-configuration, embedded servers, and streamlined setup, making it faster and easier to start a Spring project without extensive configuration.
5. What is Spring AOP and how is it used?(HCL)
Spring AOP (Aspect-Oriented Programming) is used to implement cross-cutting concerns like logging or transactions by separating them from business logic using aspects.
Remember What You Learned!
Question
Your answer:
Correct answer:
Your Answers