Introduction
Understanding the structure of a Java program is essential for writing clean, organized, and scalable code. A well-structured Java program ensures maintainability, debugging efficiency, and smooth collaboration with other developers. In this guide, we will explore the fundamental components of a Java program, from the package declaration to the main method.
Why Java Program Structure Matters
Learning Java’s structure is crucial for beginners because it sets the foundation for writing readable and efficient code. A clear structure helps you:
- Organize your code effectively.
- Avoid common coding errors.
- Make your code scalable for future modifications.
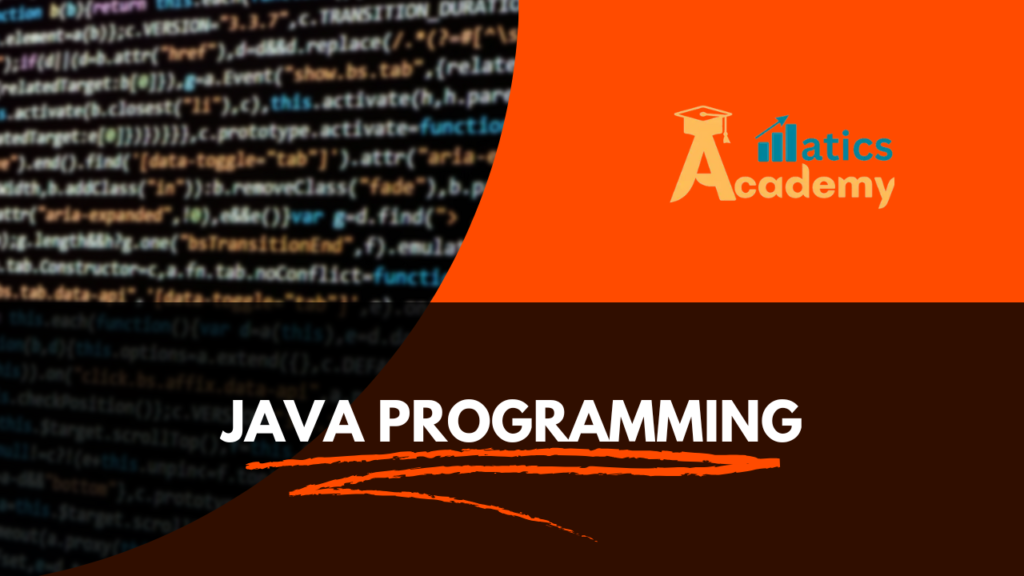
Key Elements of Java Program Structure
Every Java program follows a certain pattern, consisting of the following key elements:
- Package Declaration
- Import Statements
- Class Declaration
- Fields and Methods
- Main Method
1. Package Declaration
A Java program usually starts with the package declaration. This defines the namespace for the program, helping to organize classes and avoid naming conflicts.
package com.example; // Package Declaration
2. Import Statements
The import statement is used to bring in libraries or other classes from external packages. This is essential for accessing Java’s built-in functionality and any custom libraries you may need.
import java.util.*; // Import Statement
3. Class Declaration
The class declaration defines the blueprint for your program. Every Java program must have at least one class, and it’s here that you’ll define the fields and methods.
public class MyClass { // Class Declaration // Class components go here }
4. Fields and Methods
- Fields (or attributes) define the state of an object (i.e., the data it holds).
- Methods define the behavior of the object (i.e., actions it can perform).
Fields private int number; private String name; // Methods public void display() { System.out.println("Number: " + number + ", Name: " + name); }
5. Main Method
The main method serves as the entry point for any Java application. The Java Virtual Machine (JVM) looks for this method to start program execution. Here’s the structure:
public static void main(String[] args) { // Program execution begins here }
Full Example: Java Program Structure in Action
Let’s now combine all the elements into a simple Java program:
package com.example; // Package Declaration import java.util.*; // Import Statement public class MyClass { // Class Declaration // Fields private int number; private String name; // Constructor public MyClass(int number, String name) { this.number = number; this.name = name; } // Method to display field values public void display() { System.out.println("Number: " + number + ", Name: " + name); } // Main Method (entry point) public static void main(String[] args) { MyClass myObject = new MyClass(10, "Java"); myObject.display(); // Output: Number: 10, Name: Java } } 
Flowchart: Structure of a Java Program
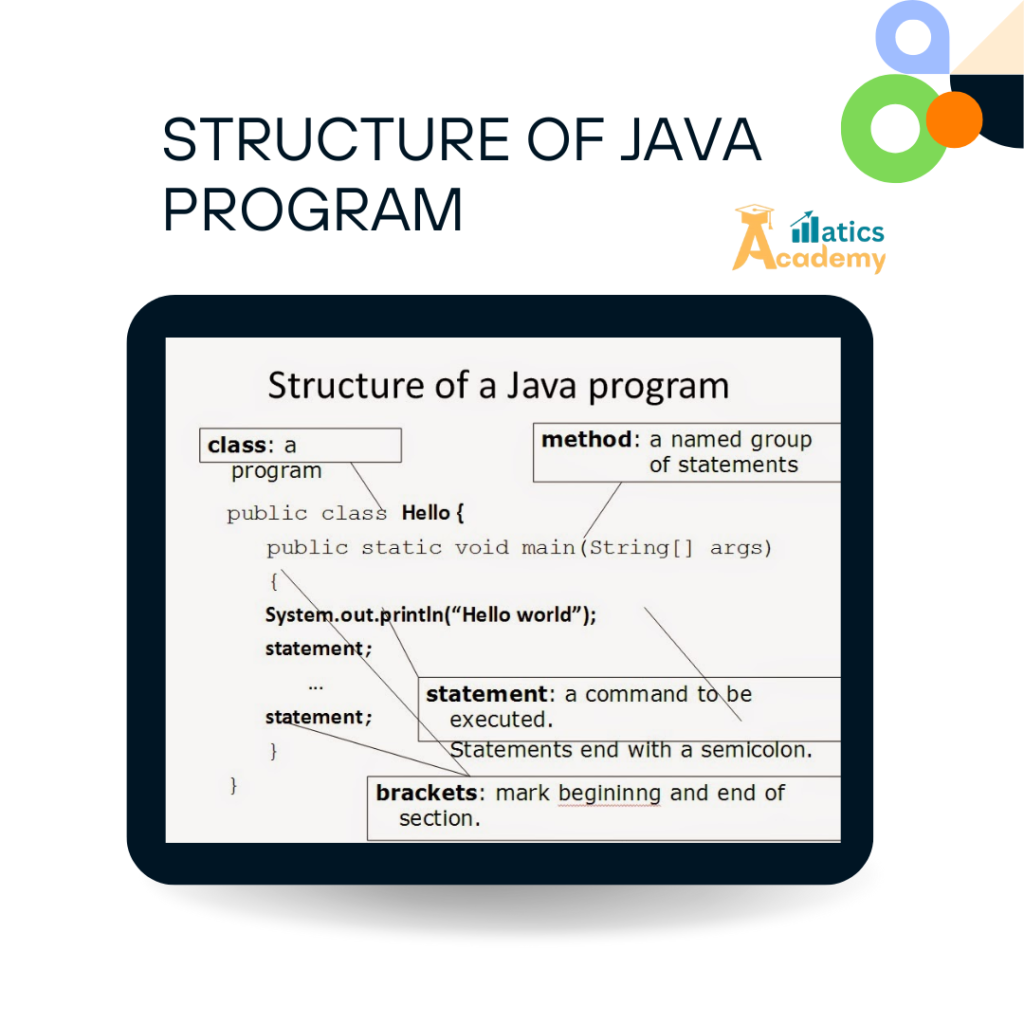
Flowchart Explanation: A visual flowchart can help understand the relationships between different components of a Java program. Above is a simple diagram to help visualize the structure:
6. Summary and Key Takeaways
A well-structured Java program includes the following components:
- Package and Import Statements: These help organize and modularize your code.
- Class Declaration: This is the blueprint for creating objects.
- Fields and Methods: Define the state and behavior of the object.
- Main Method: The entry point of any Java program.
Mastering these key elements ensures your programs are clean, maintainable, and scalable.
Learning Outcomes
After reading this guide, you’ll be able to:
- Identify the essential components of a Java program.
- Understand how to organize code with classes, methods, and fields.
- Write a simple Java program using the standard structure.
These foundational skills will help you as you progress toward more advanced Java topics.
Common Interview Questions on Java Program Structure
1. What is the main method in Java, and why is it important?
Company: Amazon
- Answer: The main method is the entry point for any Java program. It’s a static method with the signature
public static void main(String[] args)
, and it allows the Java Virtual Machine (JVM) to execute the code inside it. Without the main method, the JVM wouldn’t know where to start the program.
2. What are the differences between the import
and package
keywords in Java?
Company: Google
- Answer:
package
is used to define the namespace for the class, helping to organize the classes into a directory structure.import
is used to bring in classes or entire packages from other locations or libraries so they can be used in the current Java class.
3. Explain the structure and purpose of a Java class.
Company: Microsoft
- Answer: A Java class is a blueprint for creating objects. It defines the state (fields) and behavior (methods) of the objects it creates. The class is structured as follows:
- Class Declaration: Declares the class with the
class
keyword. - Fields (Attributes): Variables that hold the object’s state.
- Methods: Functions that define the object’s behavior.
- Constructors: Special methods used to initialize new objects.
- Class Declaration: Declares the class with the
4. What is the purpose of a constructor in Java? How does it differ from a method?
Company: IBM
- Answer: A constructor is a special type of method used to initialize objects when they are created. It has the same name as the class and does not have a return type. Unlike methods, constructors are called only once when an object is instantiated, and they are used to set initial values for the object’s fields.
5. What is the difference between a public
, private
, and protected
access modifier?
Company: Facebook
- Answer:
public
: The field or method is accessible from any other class.private
: The field or method is only accessible within the same class.protected
: The field or method is accessible within the same package or subclasses (even in different packages).
Practice Exercise: Multiple Choice Questions
What is the purpose of the main method in a Java program?
- A) It serves as a constructor.
- B) It is where execution begins.
- C) It imports classes from other packages.
- D) It defines a new class.
- Correct Answer: B) It is where execution begins.
Which keyword is used to define a class in Java?
- A) method
- B) class
- C) object
- D) function
- Correct Answer: B) class
What is the output of the following code?
public class Test { public static void main(String[] args) { System.out.println("Hello, World!"); } }
- A) Hello
- B) Hello, World!
- C) Compilation Error
- D) No output
- Correct Answer: B) Hello, World!
Additional Resources
- Books: “Effective Java” by Joshua Bloch, “Java: The Complete Reference” by Herbert Schildt.
- Online Courses: Coursera Java Programming.
- Tools: Use IntelliJ IDEA, Eclipse, or NetBeans to write and test Java code.
ITS TIME TO PLAY WITH JAVA!!
This section emphasizes the importance of understanding Java’s structure for writing well-organized, maintainable, and scalable code. Knowing the structure allows developers to write cleaner code, making it easier to read, debug, and collaborate on with other programmers. This foundational knowledge is especially beneficial for beginners.
Question
Your answer:
Correct answer:
Your Answers