Templating in Python is a powerful approach to dynamically generate content by combining static templates with dynamic data. From web development to report generation, templating plays a vital role in creating reusable and efficient workflows. This article explores templating in Python, its importance, popular tools, and real-world examples.
What is Templating in Python?
Templating is the process of defining a static structure with placeholders, which can be replaced dynamically with variable data during runtime. It enables the separation of content and logic, making applications easier to maintain and extend.
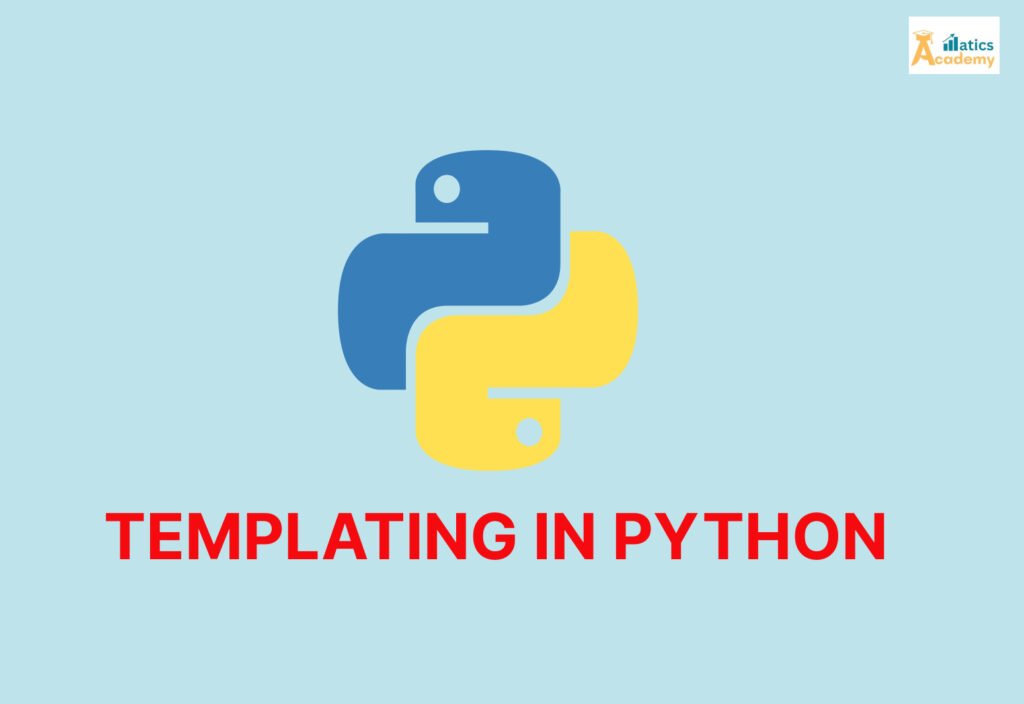
Key Features of Templating in Python:
- Reusability: Use the same template with different data inputs.
- Separation of Concerns: Keeps design (HTML or layout) separate from programming logic.
- Dynamic Content Generation: Automate the creation of dynamic files, web pages, or documents.
Why Use Templating?
Templating offers numerous benefits for developers, including:
- Efficient Content Creation: Reduce repetitive tasks by reusing templates.
- Maintainability: Simplify updates by modifying templates instead of hardcoding changes.
- Customizability: Dynamically inject variable data into templates for personalized outputs.
Popular Tools for Templating in Python
Python provides several templating libraries, each suited for specific use cases:
- Jinja2: A high-performance templating engine, widely used in web frameworks like Flask.
- Django Templates: Built into the Django web framework, offering secure and robust templating for web applications.
- Mako: A fast templating library designed for Python applications needing high efficiency.
- string.Template: A simple built-in module for straightforward text substitution tasks.
How Templating Works in Python
Templating involves defining a base template with placeholders (like {{ placeholder }}
) and substituting these placeholders with actual data during execution. Libraries like Jinja2 and Django Templates make this process seamless.
Examples of Templating in Python
1. Templating with Jinja2
Jinja2 is one of Python’s most popular templating engines. Here’s a simple example:
from jinja2 import Template # Define a template template = Template("Hello, {{ name }}! Welcome to {{ platform }}.") # Render the template with data rendered_text = template.render(name="Alice", platform="Maticts Academy") print(rendered_text) # Output: Hello, Alice! Welcome to Maticts Academy.
2. Django Templates for Web Pages
Django templates are ideal for web development and support conditional statements, loops, and filters.
Example template (index.html):
<!DOCTYPE html> <html> <head> <title>{{ title }}</title> </head> <body> <h1>Welcome, {{ user_name }}!</h1> <p>Your registered email is: {{ email }}</p> </body> </html>
Rendering the template in Django:
from django.shortcuts import render def index(request): context = { 'title': 'Homepage', 'user_name': 'Bob', 'email': 'bob@example.com' } return render(request, 'index.html', context)
3. Simple Text Substitution with string.Template
The string.Template
module is a lightweight option for templating.
from string import Template template = Template("Hello, $name! This is your $task.") # Substitute placeholders with actual data result = template.substitute(name="Charlie", task="assignment") print(result) # Output: Hello, Charlie! This is your assignment.
Advantages and Disadvantages of Templating in Python
Advantages:
- Reusable Code: Write once and use with different data sets.
- Readable Layouts: Clear separation between design and logic.
- Dynamic Outputs: Easily create customized outputs for different users.
Disadvantages:
- Learning Curve: Some templating engines (e.g., Django templates) may require learning specific syntax.
- Performance Overhead: Templating can slow down applications with high dynamic content needs.
- Limited to Template Engine Capabilities: Advanced logic might be challenging to implement.
Tips for Using Templating in Python
- Choose the Right Tool: Use Jinja2 for Flask, Django Templates for Django, and
string.Template
for simple tasks. - Keep Templates Clean: Avoid embedding complex logic directly into templates for better maintainability.
- Test Outputs: Always test rendered outputs for accuracy and security.
- Optimize for Performance: Use caching mechanisms to improve the performance of dynamic content generation.
Conclusion
Templating in Python is an essential tool for generating dynamic and reusable content efficiently. By mastering tools like Jinja2, Django Templates, and string.Template
, developers can create scalable applications with clean, maintainable, and dynamic outputs.
INTERVIEW QUESTIONS
1. What is the Purpose of Templating in Python?
Company: Google
Answer:
Templating in Python is used to separate the logic of an application from its presentation. It allows developers to dynamically generate HTML, text, or other output formats by embedding variables, loops, and conditionals within templates. This is particularly useful in web development, where templates can create dynamic content based on user input or backend data.
For example, in a web application, templates can render user-specific pages by combining static HTML with dynamic data like user names, preferences, or search results.
2. How Do You Use Jinja2 for Templating?
Company: Amazon
Answer:
Jinja2 is a popular Python templating engine. It allows developers to create dynamic templates with placeholders, loops, and conditionals.
Example:
from jinja2 import Template # Define a template with placeholders template = Template(""" <html> <head><title>{{ title }}</title></head> <body> <h1>Hello, {{ user }}!</h1> <ul> {% for item in items %} <li>{{ item }}</li> {% endfor %} </ul> </body> </html> """) # Data to render the template data = { "title": "Welcome Page", "user": "Alice", "items": ["Item 1", "Item 2", "Item 3"] } # Render the template output = template.render(data) print(output)
3. What Are the Advantages of Django Templates?
Company: Microsoft
Answer:
Django templates offer several advantages for web development:
- Separation of Concerns: Keeps presentation (HTML) separate from business logic.
- Security: Automatically escapes special characters to prevent XSS attacks.
- Integration with Views: Directly integrates with Django views, making data passing seamless.
- Customizable: Allows custom filters and tags for specific needs.
- Template Inheritance: Supports extending base templates, reducing code duplication.
Example:
A Django template can safely render user input:
<p>{{ user_input }}</p>
Even if user_input
contains <script>alert('Hack')</script>
, it is escaped as plain text, ensuring security.
4. Difference Between Jinja2 and string.Template
Company: Infosys
Answer:
Aspect | Jinja2 | string.Template |
---|---|---|
Complexity | Advanced with support for loops, conditionals, and filters. | Simpler, with basic placeholder replacement. |
Syntax | Uses {{ variable }} and {% logic %} syntax. | Uses $variable or ${variable} syntax. |
Use Cases | Ideal for complex templates, especially in web development. | Suitable for simple text replacement tasks. |
Performance | Slightly slower due to its advanced features. | Faster for lightweight operations. |
Dependencies | Requires installation (pip install jinja2 ). | Built into Python’s standard library. |
5. How to Pass Dynamic Data to a Template in Django?
Company: TCS
In Django, dynamic data is passed to templates using context dictionaries. This allows you to render data from the backend in the frontend.
QUIZZES
Templating in python Quiz
Question
Your answer:
Correct answer:
Your Answers