Introduction
TestNG is a versatile testing framework for Java that goes beyond basic unit testing to support integration testing, parallel execution, and data-driven testing. Widely used in enterprise environments, TestNG provides a powerful suite of features, making it an essential tool for ensuring software quality in large-scale applications.
What is TestNG?
TestNG (Next Generation) is a testing framework inspired by JUnit and NUnit. It’s designed to cover a broader range of test categories: unit, functional, end-to-end, and integration testing. TestNG’s powerful annotations, flexible configuration, and support for parallel execution make it ideal for scalable testing setups.
Key Benefits:
- Supports parameterized and data-driven tests.
- Facilitates parallel and sequential test execution.
- Provides flexible configuration options and detailed reports.
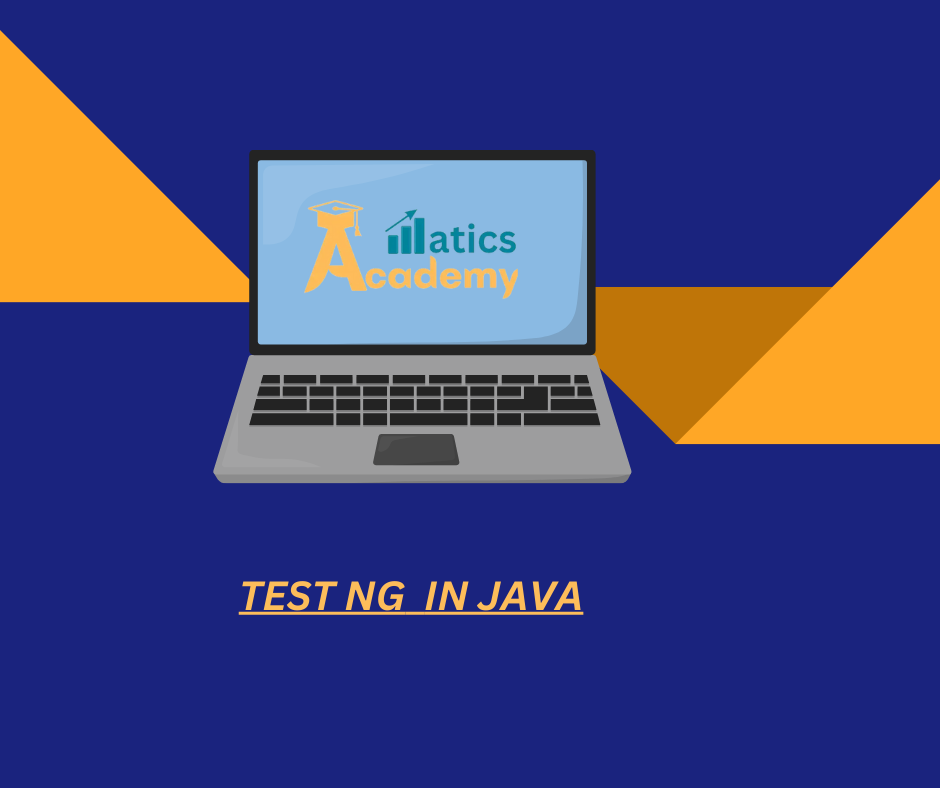
Core Features of TestNG
- Annotations: Rich set of annotations for organizing complex test cases.
- Data-Driven Testing: Enables running tests with multiple sets of data.
- Parallel Execution: Allows tests to run in parallel, optimizing testing time.
- Detailed Reports: Generates HTML and XML reports to visualize test results.
TestNG Annotations
- @Test: Marks a method as a test case.
- @BeforeSuite, @AfterSuite: Run before and after all tests in a suite, respectively.
- @BeforeClass, @AfterClass: Execute before and after each test class.
- @BeforeMethod, @AfterMethod: Execute before and after each test method.
Example: Setting Up TestNG in Java
To use TestNG, add it to your project either by installing the TestNG library or configuring it in your build file (e.g., Maven)
Maven Dependency:
<dependency> <groupId>org.testng</groupId> <artifactId>testng</artifactId> <version>7.4.0</version> <scope>test</scope> </dependency>
Basic Test Case Example:
import org.testng.annotations.Test; import static org.testng.Assert.assertEquals; public class CalculatorTest { @Test public void testAddition() { Calculator calculator = new Calculator(); int result = calculator.add(5, 3); assertEquals(result, 8); } }
This test checks that the add
method in the Calculator
class correctly computes 5 + 3
.
Advanced Features of TestNG
- Parameterized Tests: Pass multiple sets of data to a single test
- Parallel Execution: Configure parallel test execution to speed up testing time, especially useful for large test suites.
- Grouping Tests: Organize related tests into groups with the
groups
attribute in the@Test
annotation.
Common Interview Questions
1. What advantages does TestNG offer over JUnit?
Company: Capgemini
Answer: TestNG provides more powerful and flexible features than JUnit, such as built-in support for parallel test execution, dependent test methods, data-driven testing using @DataProvider
, and detailed HTML reports. This makes TestNG highly suitable for complex test scenarios and large test suites.
2. Explain key TestNG annotations and their roles.
Company: TCS
Answer: Key TestNG annotations include:
- @Test: Marks a method as a test case.
- @BeforeMethod and @AfterMethod: Run before and after each test method, respectively, for setup and cleanup.
- @BeforeClass and @AfterClass: Execute once before and after all methods in a class, typically for initializing and releasing shared resources.
- @DataProvider: Supplies data for parameterized tests, supporting data-driven testing.
3. How can you run multiple test cases in parallel with TestNG?
Company: Wipro
Answer: TestNG enables parallel execution by configuring the parallel
attribute in the XML file or by setting parallel = true
in test annotations. You can specify parallelism at the suite, test, or method level, optimizing test execution time across multiple threads.
4. What is the use of @DataProvider
in TestNG, and how does it work?
Company: Infosys
Answer: @DataProvider
is used for parameterized testing, allowing a test method to run multiple times with different sets of data. It returns an Object[][]
, where each set is provided as arguments to the test, making data-driven testing seamless and efficient in TestNG.
5. How does TestNG support test dependency management, and when would you use it?
Company: Accenture
Answer: TestNG’s dependsOnMethods
and dependsOnGroups
attributes let you specify dependencies between tests. This is helpful when certain tests rely on the success of others, ensuring logical order and selective execution, especially in integration or end-to-end testing scenarios.
Practice Exercises
Write a TestNG test case for a method that checks if a number is prime.
First, we’ll create a MathUtils
class with an isPrime(int n)
method, and then write a TestNG test case for it.
- Create a test suite with multiple classes and test methods using parallel execution.
public class MathUtils { public boolean isPrime(int n) { if (n <= 1) return false; for (int i = 2; i <= Math.sqrt(n); i++) { if (n % i == 0) return false; } return true; } }
TestNG Test Case for Prime Number
import org.testng.Assert; import org.testng.annotations.Test; public class MathUtilsTest { MathUtils mathUtils = new MathUtils(); @Test public void testIsPrimePositiveCase() { Assert.assertTrue(mathUtils.isPrime(7), "7 should be prime"); } @Test public void testIsPrimeNegativeCase() { Assert.assertFalse(mathUtils.isPrime(4), "4 should not be prime"); } @Test public void testIsPrimeForOne() { Assert.assertFalse(mathUtils.isPrime(1), "1 is not prime"); } @Test public void testIsPrimeForNegativeNumber() { Assert.assertFalse(mathUtils.isPrime(-5), "-5 is not prime"); } }
2. TestNG Test Suite with Parallel Execution
To demonstrate parallel execution, let’s create an additional class with a different test, then set up a TestNG XML configuration file to run these test classes in parallel.
public class Calculator { public int add(int a, int b) { return a + b; } }
CalculatorTest Class
import org.testng.Assert; import org.testng.annotations.Test; public class CalculatorTest { Calculator calculator = new Calculator(); @Test public void testAddition() { Assert.assertEquals(calculator.add(5, 3), 8, "5 + 3 should be 8"); } }
Additional Resources
- TestNG Documentation
- Practical Unit Testing with TestNG and Mockito by Tomek Kaczanowski
PLAY WITH JAVA..!!
Question
Your answer:
Correct answer:
Your Answers