The Optional class in Java is a powerful feature introduced in Java 8 that helps developers handle the absence of values without the risk of NullPointerExceptions
. This guide will explore how to use the Java Optional class effectively, providing examples and best practices for clean, maintainable code. By understanding the Optional type in Java, you can improve your code’s safety and readability. This optional class that related to object.
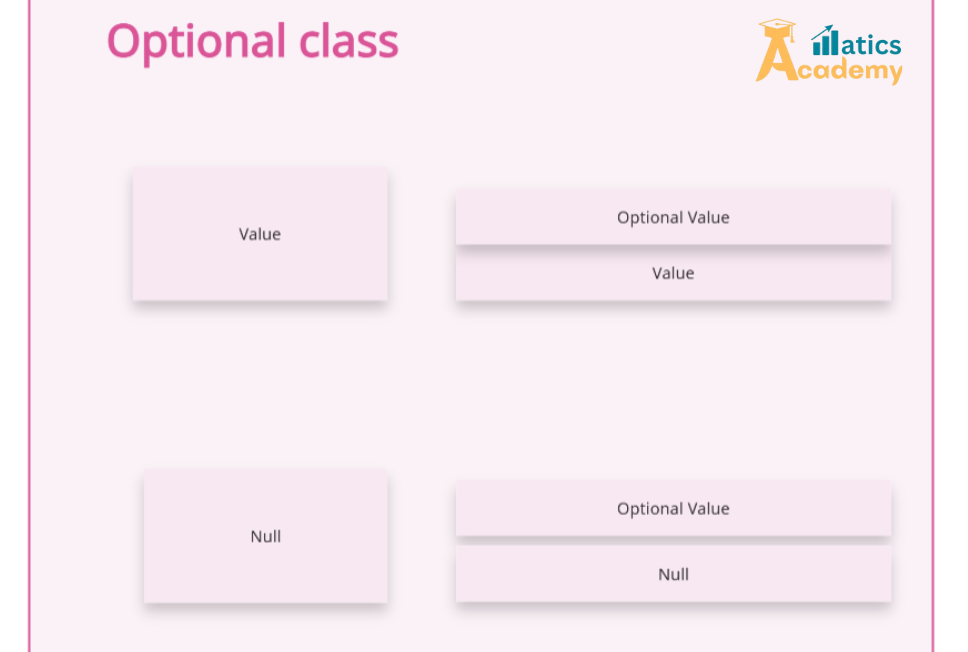
Why Use the Optional Class in Java?
Handling null values has always been a challenge in Java programming. The Optional
class simplifies this by providing a container that may or may not hold a value. Using Optional
enhances code readability and reduces the likelihood of runtime errors.
How to Create Optional Instances
The Optional
class provides several methods to create instances:
Optional.of(value)
: Creates anOptional
that contains the specified non-null value. ThrowsNullPointerException
if the value is null.
Optional.ofNullable(value)
: Creates anOptional
that can either hold a non-null value or be empty (null-safe).
Optional.empty()
: Returns an emptyOptional
instance, indicating no value is present.
Key Methods of the Optional Class
Understanding the key methods of the Optional
class is crucial for effective usage:
isPresent()
: Returnstrue
if a value is present; otherwise,false
.
get()
: Retrieves the value if present; throwsNoSuchElementException
if not.
ifPresent(Consumer<? super T> action)
: Executes a specified action if a value is present.
orElse(T other)
: Returns the value if present; otherwise, returns a specified default value.
orElseGet(Supplier<? extends T> other)
: Similar toorElse
, but uses aSupplier
to generate the default value.
map(Function<? super T,? extends U> mapper)
: Applies a transformation function to the value if present and returns anOptional
of the transformed value.
flatMap(Function<? super T,Optional<U>> mapper)
: Similar tomap
, but the function must return anOptional
.
Example of Using the Optional Class in Java
Here’s a practical example demonstrating how to use the Optional
class in a Java program.
import java.util.Optional; public class OptionalExample { public static void main(String[] args) { // Creating an Optional with a non-null value Optional<String> optionalValue = Optional.of("Hello, Optional!"); // Checking if a value is present if (optionalValue.isPresent()) { System.out.println("Value is present: " + optionalValue.get()); } else { System.out.println("No value present."); } // Creating an empty Optional Optional<String> emptyOptional = Optional.empty(); // Using ifPresent to execute a block of code if a value is present optionalValue.ifPresent(value -> System.out.println("Optional contains: " + value)); // Providing a default value if the Optional is empty String defaultValue = emptyOptional.orElse("Default Value"); System.out.println("Value: " + defaultValue); // Using map to transform the value if present Optional<String> transformedValue = optionalValue.map(String::toUpperCase); transformedValue.ifPresent(value -> System.out.println("Transformed Value: " + value)); } }
Explanation of the Example
- Creating an Optional:
- The
Optional
namedoptionalValue
is created with a non-null string. - An empty
Optional
namedemptyOptional
is also created.
- The
- Checking Presence:
- The
isPresent()
method checks ifoptionalValue
has a value, andget()
retrieves it.
- The
- Using ifPresent:
- The
ifPresent
method prints the content ofoptionalValue
if it is present.
- The
- Providing Default Values:
- The
orElse
method provides a default value foremptyOptional
.
- The
- Transforming Values:
- The
map
method is used to transformoptionalValue
to uppercase if it is present.
- The
Output:
Value is present: Hello, Optional!
Optional contains: Hello, Optional!
Value: Default Value
Transformed Value: HELLO, OPTIONAL!
Explanation of the Output:
- Value Presence Check: The program first checks if
optionalValue
contains a value. Since it does, it prints:pythonCopy codeValue is present: Hello, Optional!
Value is present: Hello, Optional!
2. Using ifPresent
: It then checks if optionalValue
is present and prints:mathematicaCopy codeOptional contains: Hello, Optional!
Optional contains: Hello, Optional!
3. Default Value for Empty Optional: The empty Optional
does not contain a value, so it returns the default value:vbnetCopy codeValue: Default Value
Value: Default Value
4. Transforming the Value: Finally, it transforms the value in optionalValue
to uppercase and prints:vbnetCopy codeTransformed Value: HELLO, OPTIONAL!
Transformed Value: HELLO, OPTIONAL!
Best Practices for Using Optional in Java
- Return Optional from Methods: Use Optional as the return type for methods that might return a null value to enhance clarity and prevent errors.
- Avoid Optional in Fields: It’s best not to use Optional for fields in classes or as method parameters to maintain simplicity.
- Utilize Optional Methods: Leverage the various methods provided by Optional to improve null handling and enhance code readability.
Beginner Project Idea: “Optional Value Checker”
Create a simple Java project that demonstrates how the Optional
class can be used to handle user inputs and check for optional values (e.g., a name or age).
Project Description:
- Input Gathering: Ask the user to input their name and age.
- Optional Usage: Store the name and age using
Optional.ofNullable()
to handle cases where they may or may not provide these values. - Null Safety: Use the
isPresent()
,orElse()
, andifPresent()
methods to check and handle missing or present data. - Transform Data: Optionally, convert the name to uppercase and print it if the value is present.
Sample Code:
NOTE: this code doesn’t run any online compiler instead use the IDE (eclipse or intellij)
import java.util.Optional; import java.util.Scanner; public class OptionalValueChecker { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); // Ask user for name and age System.out.println("Enter your name (or leave blank):"); String nameInput = scanner.nextLine(); System.out.println("Enter your age (or leave blank):"); String ageInput = scanner.nextLine(); // Using Optional to handle possible null values Optional<String> name = Optional.ofNullable(nameInput.isEmpty() ? null : nameInput); Optional<String> age = Optional.ofNullable(ageInput.isEmpty() ? null : ageInput); // Display name if present name.ifPresent(nameVal -> System.out.println("Name: " + nameVal)); System.out.println("Age: " + age.orElse("Not provided")); // Optionally transform the name to uppercase name.map(String::toUpperCase).ifPresent(nameVal -> System.out.println("Name in uppercase: " + nameVal)); scanner.close(); } }
Explanation:
- The program asks the user to input their name and age. If the user leaves the input blank, it creates an
Optional
with a null value. - It demonstrates how
Optional
handles optional values safely by using methods likeisPresent()
,orElse()
, andifPresent()
. - The name is optionally transformed to uppercase and printed if present.
Interview Questions
1. Company: Zoho
Question: How does the Optional
class improve code readability in Java? Can you provide an example of using Optional
to avoid multiple null checks and make the code cleaner?
Answer: The Optional
class reduces the need for repetitive null checks, making code more readable and concise. For example, instead of checking if an object is null before accessing a method, Optional
allows chaining with methods like ifPresent
to handle values only if they exist.
2. Company: TCS
Question: What is the difference between Optional.of()
and Optional.ofNullable()
? In what scenarios would you use each when handling potential null values?
Answer: Optional.of()
is used when the value is guaranteed to be non-null, while Optional.ofNullable()
can handle both null and non-null values. Use ofNullable()
when a value might be null to avoid NullPointerException
.
3. Company: Infosys
Question: Explain how ifPresent()
in the Optional
class can help avoid NullPointerException
in Java. Could you provide an example where this method simplifies handling an optional value?
Answer: ifPresent()
executes a code block only if a value is present, helping prevent NullPointerException
. For example, optionalValue.ifPresent(val -> System.out.println(val))
safely prints the value if it’s present, without needing a null check.
4. Company: Capgemini
Question: What are the key differences between map()
and flatMap()
in the Optional
class? Could you illustrate when you would use each method in the context of optional values in Java?
Answer: map()
transforms values within an Optional
, while flatMap()
is used when the transformation itself returns an Optional
. Use map()
for simple transformations and flatMap()
when nested optionals need to be flattened into a single result.
Conclusion
The Optional class in Java is an essential tool for safely handling optional values. By effectively utilizing this class, developers can write clearer, more expressive code while significantly reducing the risk of null-related errors.
optional-class-quiz
Question
Your answer:
Correct answer:
Your Answers